Quick start (with cohosting)
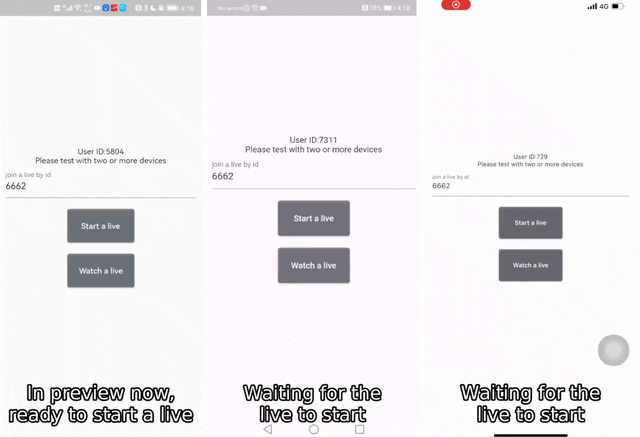
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Integrate the SDK
Import the SDK
- Add @zegocloud/zego-uikit-prebuilt-live-streaming-rn as dependencies.
yarn
npm
yarn add @zegocloud/zego-uikit-prebuilt-live-streaming-rn
1
npm install @zegocloud/zego-uikit-prebuilt-live-streaming-rn
1
- Add other dependencies.
Run the following command to install other dependencies for making sure the @zegocloud/zego-uikit-prebuilt-live-streaming-rn
can work properly:
yarn
npm
yarn add @zegocloud/zego-uikit-rn react-delegate-component zego-express-engine-reactnative@^3.14.5 zego-zim-react-native@2.16.0 @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-device-info react-native-orientation-locker@^1.7.0
1
npm install @zegocloud/zego-uikit-rn react-delegate-component zego-express-engine-reactnative@^3.14.5 zego-zim-react-native@2.16.0 @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-device-info react-native-orientation-locker@^1.7.0
1
Using the Live Streaming Kit
- Specify the
userID
anduserName
for connecting the LiveStreaming Kit service. - Create a
liveID
that represents the live streaming you want to make. - Set the
plugins
toZIM
plug-in.
Note
userID
andliveID
can only contain numbers, letters, and underlines (_).- Using the same
liveID
will enter the same live streaming.
Warning
With the same liveID
, only one user can enter the live stream as host. Other users need to enter the live stream as the audience.
HostPage.js
import React from 'react';
import { StyleSheet, View } from 'react-native';
import ZegoUIKitPrebuiltLiveStreaming, { HOST_DEFAULT_CONFIG } from '@zegocloud/zego-uikit-prebuilt-live-streaming-rn';
import * as ZIM from 'zego-zim-react-native';
export default function HostPage(props) {
return (
<View style={styles.container}>
<ZegoUIKitPrebuiltLiveStreaming
appID={yourAppID}
appSign={yourAppSign}
userID={userID}
userName={userName}
liveID={liveID}
config={{
...HOST_DEFAULT_CONFIG,
onStartLiveButtonPressed: () => {},
onLiveStreamingEnded: () => {},
onLeaveLiveStreaming: () => { props.navigation.navigate('HomePage') },
}}
plugins={[ZIM]}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
zIndex: 0,
}
});
1
Configure your project
- Android:
- Open the
my_project/android/app/src/main/AndroidManifest.xml
file and add the following:
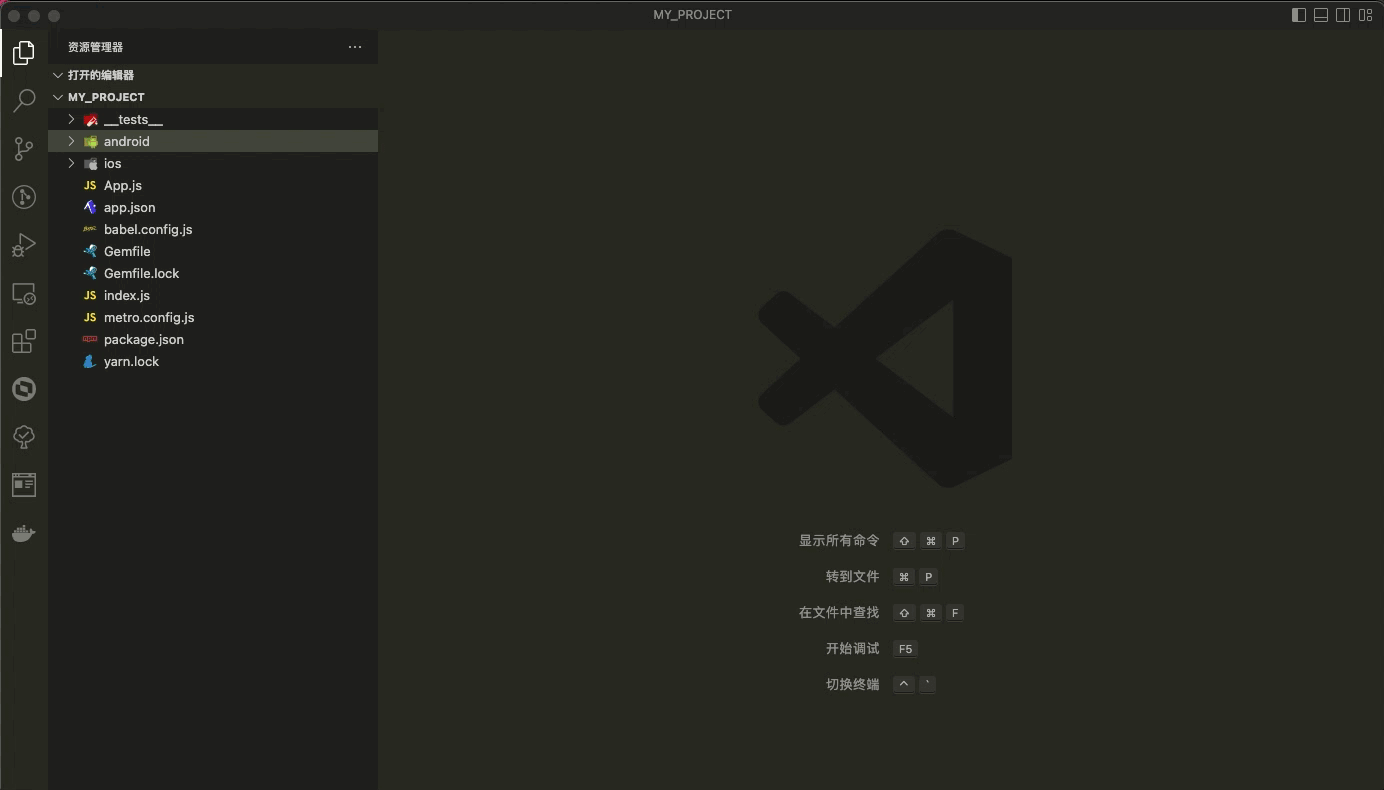
Untitled
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-permission android:name="android.permission.VIBRATE"/>
1
- Open the
my_project/android/app/proguard-rules.pro
file and add the following:
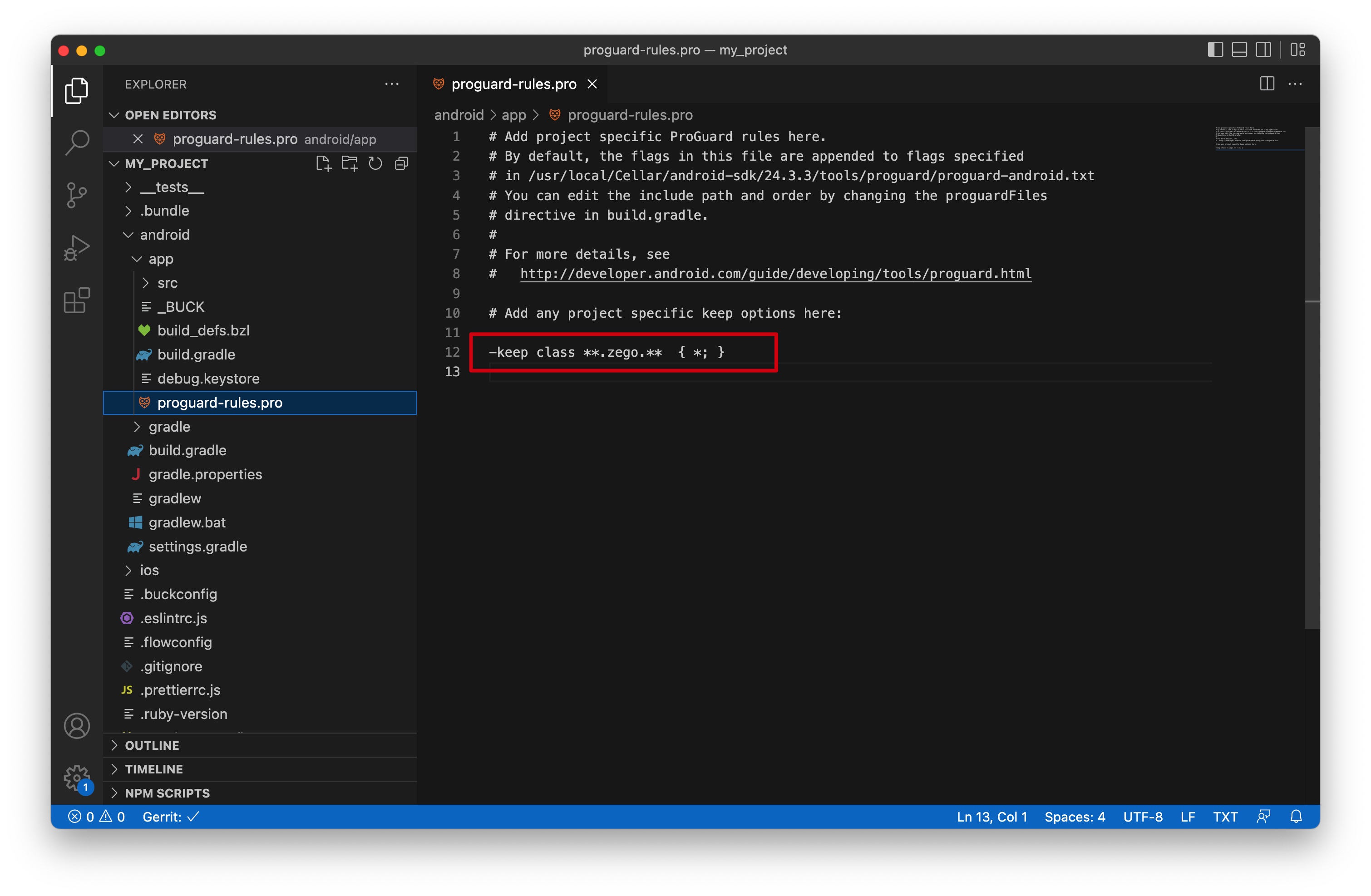
Untitled
-keep class **.zego.** { *; }
1
- iOS:
Open the my_project/ios/my_project/Info.plist
file and add the following:
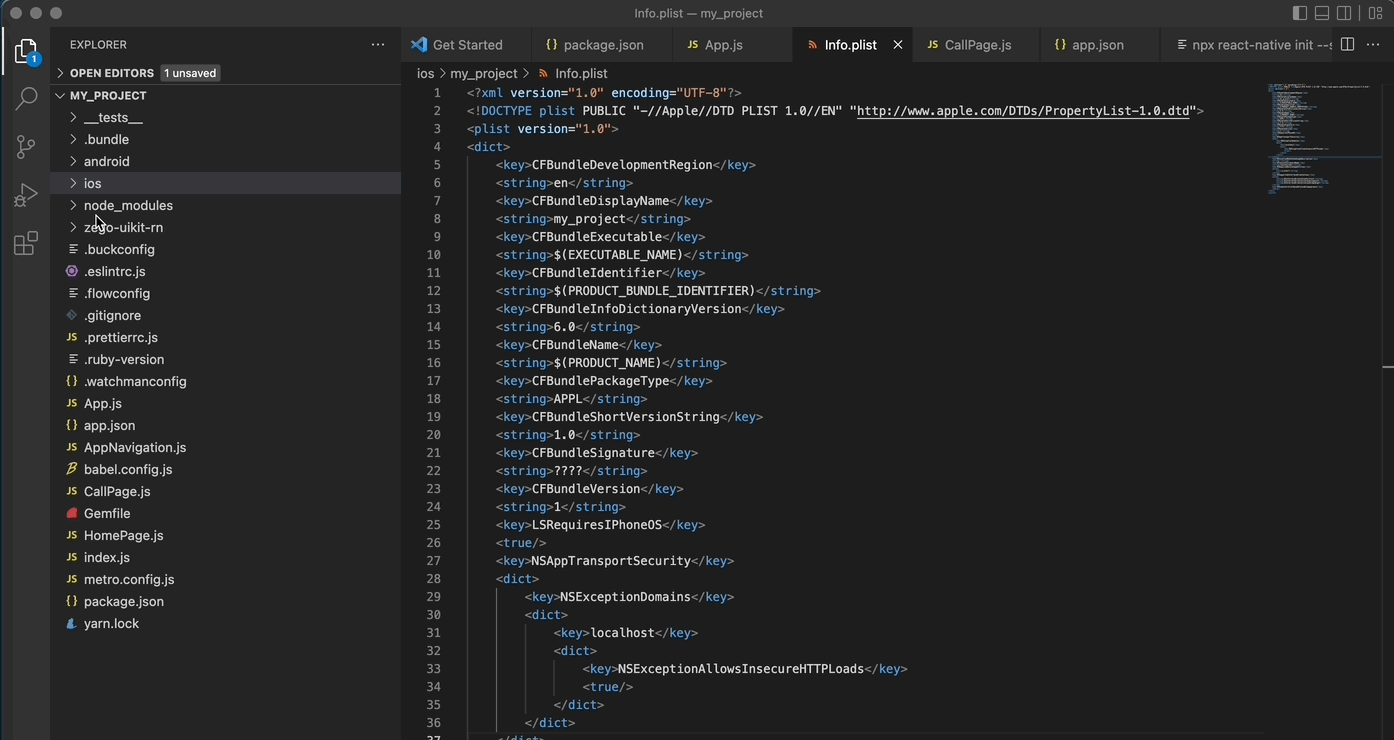
Untitled
<key>NSCameraUsageDescription</key>
<string>We need to use the camera</string>
<key>NSMicrophoneUsageDescription</key>
<string>We need to use the microphone</string>
1
Run & Test
Note
If your device is not performing well or you found a UI stuttering, run in Release mode for a smoother experience.
- Run on an iOS device:
yarn
npm
yarn ios
1
npm run ios
1
- Run on an Android device:
yarn
npm
yarn android
1
npm run android
1
Resources
Sample code
Click here to get the complete sample code.