While appearing for an interview for an iOS app developer position, you need to be well-prepared beforehand to secure the job. To take your preparations to the next level, go through a set of iOS interview questions that interviewers usually ask. Once you know the answers to the common app development questions, you will have the confidence to ace the interview. This article introduces you to the best iOS coding interview questions for developers of every skill set.
iOS Basic Interview Questions for Freshers
When you have no previous working experience, the interviewer will only judge you based on the knowledge you display during the interview. So, freshers should work hard and fully prepare to display their app development acumen. The following iOS developer interview questions can help you secure your first job.
Question 1. Explain different iOS app states as a fresher developer.
When answering this iOS coding interview question, you must show your knowledge about different app states by detailing their explanation one by one, as follows:
- Not Running: While discussing this state, you should tell the interviewer that it occurs when the system or user hasn’t launched the app.
- Inactive: This state shows that the app is running in the foreground but is not receiving events. Moreover, you can further add that this can happen when there’s an interruption, such as an incoming call.
- Active: As a fresher, you should also know that this state shows that the app is running in the foreground and is receiving events. Other than that, we recommend you display your knowledge by explaining that it is the normal state for foreground apps and where most app activity occurs.
- Background: Additionally, explain that apps enter this state when the user presses the home button or switches to another app.
- Suspended: Finally, interview candidates need to explain that the app in this state remains in memory but does not execute any code. If the system needs more memory, it may remove suspended apps without notice to make space for the foreground app.
Question 2. What is the most common programming language used for iOS app building?
Every experienced and fresher developer should know the answer to this iOS interview question. So, while answering such an easy question, explain that Swift stands out as the most common programming language for iOS app development.
Using this language developed by Apple, programmers can build apps for iOS and other such operating systems. Moreover, its modern features and performance make it the go-to option for iOS developers.
Question 3. Can you give us an overview and use cases of Core Data?
A fresher candidate should have knowledge about Core Data, as it is a persistence framework provided by Apple for the iPhone and its other operating system applications. When answering this iOS developer interview question, explain it lets developers manage the model layer objects. Other than that, you can explain its use cases to have a better impact on the interviewer.
- Object Graph Management: This framework can manage complex object graphs, including managing object life cycles and relationships between objects.
- Data Model: In addition, Core Data uses a model to define the structure of your data.
- Performance Optimization: You can also explain that it can efficiently fetch, filter, and manipulate large amounts of data to improve app performance.
- Undo and Redo: With the help of this feature, this framework allows undo and redo of single and multiple operations, which can be useful in applications.
Question 4. Talk us through the conditional conformances in Swift
While discussing the answer to this iOS coding interview question, you should explain the primary function of conditional conformances in Swift. So, you can explain that it allows a generic type to conform to a protocol only if its type parameters meet certain conditions.
For example, you can make an array conform to a protocol only if its elements conform to that protocol. Therefore, this conformance enhances type safety and flexibility by enabling more precise and contextual behavior.
Question 5. Discuss the significant difference between an array and a set
To answer this iOS interview question, describe the major difference between an array and a set in how they store and manage elements. On one hand, an array shows an ordered collection that allows duplicate elements and maintains the order of insertion.
In contrast, a set is an unordered collection that ensures all elements are unique and does not maintain any specific order. Moreover, sets are optimized for fast membership tests, making them efficient for checking the presence of elements.
Question 6. Can you briefly describe the types of control transfer statements used in Swift?
As a fresher developer, you should know that control transfer statements change the flow of execution in a program in Swift. So, while answering this iOS developer interview question, briefly mention the function of each of these statements as follows:
- Break: Exits the current loop or switch statement immediately.
- Continue Skips the current loop iteration and proceeds to the next.
- Return: Leaves a function to come back to a value to the caller.
- Throw: Push away an error from a function to indicate that an error has occurred.
- Defer: Schedules code to be executed just before the current scope exits.
Question 7. How do you make use of the guard statement in iOS apps?
The interviewer may ask this iOS coding interview question from a fresher to test his knowledge. To show your wisdom, you should describe that it transfers program control out of scope if a condition is not met.
Apart from that, it helps in early exit by ensuring that certain conditions are true before proceeding with the rest of the code. Furthermore, the guard statement must have an “else” clause, which executes if the condition is false, typically containing a return or throw statement to exit the current scope.
Question 8. List and explain two different types of classes in Swift
When answering this basic iOS interview question, you should make sure to explain the following types of classes in detail:
- Regular Classes: As a standard class, they can easily be instantiated and subclassed into further segments. Moreover, you can make use of these classes to enclose data and behavior, as they support inheritance, methods, and initializers.
- Final Classes: On the other hand, these classes cannot be subclassed, as their name suggests. Therefore, declaring a class as final ensures that it is the end of its inheritance hierarchy.
Question 9. Will you be able to elaborate on the architecture of iOS?
During the interview, this iOS developer interview question will surely come into discussion. As a fresher candidate, the interviewer will be expecting you to detail the architecture in the following manner:
- Cocoa Touch: This top layer provides frameworks for building applications, including UIKit for UI elements, Foundation for basic data types, and more.
- Media: When talking about this layer, describe that it offers multimedia services, including graphics, audio, and video frameworks, such as Core Graphics.
- Core Services: Moreover, you can mention that this layer provides fundamental services, including Core Foundation, SQLite, and networking.
- Core OS: It stands out as the lowest layer, containing the kernel, device drivers, and low-level interfaces to ensure security and power management.
Question 10. What do you mean by inheritance in Swift programming language?
While answering this basic iOS interview question, explain that this property allows one class to inherit methods and other characteristics from another class. In addition, these subclasses can override superclass methods to provide specific implementations and add new properties. Other than that, you should explain that Swift supports single inheritance, meaning a class can inherit from only one superclass.
iOS Coding Interview Questions for Intermediate
After gaining experience in the field, you may look to upgrade to a better job. For this purpose, developers also have to acquire advanced knowledge of iOS application development. To help your cause, we will discuss the advanced iOS interview questions.
Question 1. Can you state the significant difference between “didSet” and “willSet” in Swift?
As an intermediate-level developer, you should know that these property observers run code in response to changes in a property’s value. When answering this iOS coding interview question, you can explain the difference between them as follows:
- willSet: When it comes to this observer, the developer calls it before they set the value of the property. It receives the new value as a parameter, allowing you to perform actions or validations before the property actually changes.
- didSet: On the other hand, “didSet”’ comes into action immediately after the change in the property’s value. Therefore, developers can utilize this observer to perform actions or updates based on the new value.
Question 2. Discuss the function of Method Swizzling in app development
Developers with a good knowledge of app building will know that method swizzling is a technique in Objective-C and Swift. Using this technique, the implementation of a method in a class is swapped with another method, typically at runtime. To add more depth to your answer, discuss the following application of this technique while answering this iOS interview question:
- Logging and Analytics: You can swizzle methods to automatically track user interactions, such as view appearances or button taps.
- Bug Fixes and Patching: This technique can be used to patch or fix bugs in system classes or third-party libraries without waiting for an official update.
- Custom Behavior: It also enables the customization of system behavior, such as changing the default behavior of UIKit components to meet specific app requirements.
Question 3. Tell us about the purpose of the reuse identifiers
When answering this iOS developer interview question, explain that reuse identifiers act as an essential part of the efficient memory management strategies in iOS development. Moreover, they work towards performance optimization with table views (UITableView) and collection views (UICollectionView). While mentioning its purpose, you can tell the interviewer that these identifiers allow for the reuse of cells that are no longer visible on the screen.
Question 4. List down all the types supported by NSUserDefaults
iOS app developers who are up to date with the development field know that “NSUserDefaults” is now known as UserDefaults in Swift. When talking about the types it supports, list down the following names while answering this iOS coding interview question:
- String
- Double
- URL
- Data
- Array
- Dictionary
Question 5. Will you be able to write about the JSON framework the iOS apps support?
Advanced or even intermediate-level developers should know that SBJSON has emerged as a popular JSON parsing library for handling JSON data in iOS applications. As it parses JSON data arriving incrementally, this framework can prove to help handle large datasets efficiently.
Other than that, it offers different methods and options for generating JSON, allowing developers to choose the approach that best fits their needs.
Question 6. What do you know about Dynamic Dispatch in iOS app development?
You should handle such an advanced iOS interview question with extra care, as the interviewer will be looking for technicalities in the answer. So, start by explaining that dynamic dispatch in iOS development refers to the runtime mechanism where the Objective-C runtime determines which method implementation to execute.
So, this allows for polymorphism, where objects of different classes can respond differently to the same message. In Objective-C, this behavior is built-in due to its dynamic nature, while in Swift, you can control it using the “@obj” attribute or protocols.
Question 7. Give details about some iOS implementation options for persistence and storage
When asked such a technical iOS interview question, you should take your time and think about all the options available. Afterward, you can explain any of the following options to have a good impact on the interviewer:
- Core Data: This framework provides an object-oriented way to manage and persist data while developing iOS apps. In addition, Core Data supports relationships, versioning, and schema migrations, which makes it ideal for complex data models.
- SQLite: While using Direct SQLite through libraries or frameworks like FMDB, developers can manage relational databases directly. Therefore, this option is suitable for applications requiring SQL querying capabilities and complex data structures.
- File System: During the app development, iOS provides APIs to read from and write to the file system. So, you can use this approach to store large files, such as images, videos, or documents.
- Realm: It stands out as a mobile database that provides a simpler alternative to Core Data or SQLite. Apart from that, Realm is known for its fast performance and support for reactive programming with real-time updates.
Question 8. Which languages are utilized for iOS app development other than Swift?
As an intermediate-level developer, you should be able to build iOS apps utilizing different programming languages. To justify your experience, you can list down the following languages while answering this iOS coding interview question:
- Objective-C
- JavaScript
- C++
- Python
- Ruby
Question 9. Explain different types of design patterns in iOS app development
You need to have an in-depth knowledge of the design patterns for iPhone applications to answer this iOS interview question.
- Facade: Such a design pattern provides a simplified interface to a complex subsystem of classes, which makes it easier to use and reduces dependencies on subsystem components.
- Memento: Developers can utilize it to capture and externalize an object’s internal state without violating encapsulation.
- Decorator: Using this pattern, developers can add behavior to individual objects dynamically without affecting the behavior of other objects from the same class.
- Adapter: This pattern allows objects with incompatible interfaces to work together by acting as a bridge between them.
Question 10. How can you differentiate between “ViewDidLoad” and “ViewDidAppear” in the iPhone app building?
To test your knowledge about iOS app development, interviewers can ask advanced iOS interview questions. So, you need to explain the significant differences by explaining that developers call “viewDidLoad” once per instance of the view controller when its view hierarchy loads into memory.
On the other hand, they make use of “viewDidAppear” every time the view appears on the screen. In addition, programmers utilize “viewDidLoad” for initial setup and “viewDidAppear” for tasks that need to be performed each time the view becomes visible or active.
iOS Interview Questions for Advanced Candidates
As you move towards higher positions, interviewers will expect you to know everything related to iPhone app development. To test your knowledge, they will ask problem-solving questions to see how you will manage different problems during the application life cycle. So, go through these advanced iOS interview questions to take your knowledge to the next level.
Question 1. How will you achieve concurrency in iOS apps?
Appearing as an experienced developer, you should know about different methods and techniques to perform this task. Among these methods, a senior developer must mention the following while answering this iOS interview question:
- Grand Central Dispatch: GCD is a low-level API for managing concurrent operations. Other than that, it provides a thread pool, work queues, and dispatch mechanisms to efficiently execute tasks concurrently.
- Operation Queues: Built on top of GCD, this method manages and executes instances of Operation objects, which encapsulate the code and data needed for a task.
- Async/Await: Introduced in Swift 5.5, this technique simplifies asynchronous code by allowing developers to write functions that look like synchronous code.
- Thread Programming: Though less common due to complexity and overhead, developers can directly create and manage threads using APIs like Thread.
Question 2. Name some of the methods you can utilize to reduce the size of the iOS app
By asking just the names of the techniques in this iOS coding interview question, interviewers will try to assess the number of methods you know about. Irrespective of your knowledge about their workings, you can name these methods to impress the interviewer:
- Asset Optimization
- App Thinning
- Code Optimization
- Resource Bundling
- Dynamic Linking
- Network Resource Management
Question 3. Talk about the Raw Strings in Swift for iOS app development
When answering this advanced iOS interview question, you can explain that raw strings simplify writing regular expressions by reducing the need to escape backslashes. Other than that, users can use them to write file paths or URLs that contain backslashes or other special characters.
So, they increase code readability by eliminating the need for multiple escape characters and reducing the likelihood of errors related to incorrect escaping.
Question 4. Can you explain the best Swift’s pattern-matching techniques?
As a senior developer, you should answer this advanced iOS interview question by detailing each method, as explained below:
- Switch Statement: Using this technique in Switch, developers can match against various data types and conditions.
- Tuple Matching: You can also utilize pattern matching with tuples to destructure and match multiple values.
- Pattern Matching in “for” Loops: Advanced developers can make use of pattern matching in “for” loops to iterate over collections conditionally.
- Value Binding: Other than that, the switch statement can bind matched values to constants or variables.
Question 5. How can you handle memory management within iOS apps?
While answering this iOS coding interview question, you should explain that Automatic Reference Counting handles memory management in iOS apps. Additionally, careful use of memory-intensive resources, implementing lazy loading, and releasing unused objects help manage memory efficiently. Other than that, tools like Xcode’s Instruments can profile and debug memory usage to identify and resolve memory-related issues.
Boost iOS Development with ZEGOCLOUD for Real-Time Communication
Developers who want to boost their communication app development process can get help from the UIKits offered by ZEGOCLOUD. After you ace the interview with the help of the previously discussed iOS interview questions, these APIs and SDKs will help you get better and more productive at your new job. While creating iOS applications, you can utilize these communication APIs to integrate advanced features into your apps.
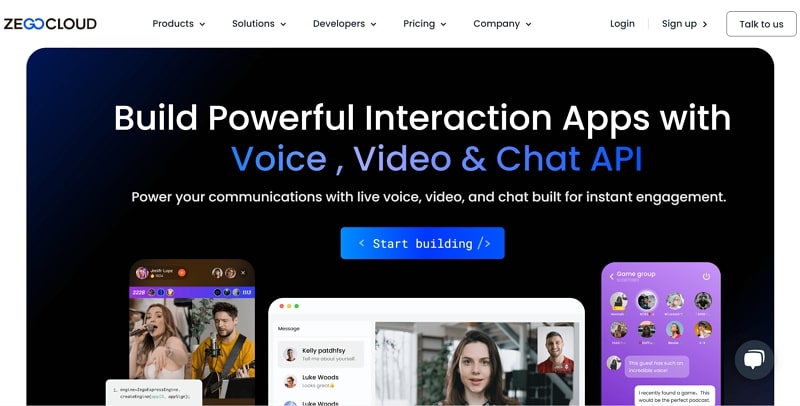
Other than that, ZEGOCLOUD will help you integrate audio calling and video calling features into your iPhone applications to build an all-in-one platform. Senior developers who want to experiment with live broadcasting and gaming applications will be able to find relevant APIs within this platform. Using the ultra-low latency SDKs of ZEGOCLOUD, you can provide an optimal streaming and calling experience to iOS app users.
Conclusion
Throughout this article, we have explained the perfect answer to iOS developer interview questions to help you get your dream job. No matter if you are a fresher or senior app developer, this article has some questions that will help you prepare for your interview better than competitors. Moreover, you can get better at iOS application development with the help of APIs and SDKs offered by ZEGOCLOUD.
Read more:
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!