To develop a successful karaoke app, it’s crucial to combine robust audio technology with an engaging user experience. Karaoke app development has gained immense popularity, enabling users to sing along to their favorite songs, record performances, and share them with others. In this article, we will explore the key steps and considerations involved in karaoke app development, from selecting the right technologies to creating a user-friendly interface that offers a captivating singing experience.
What is a Karaoke App?
A karaoke app is a mobile or web-based application that allows users to sing along to popular songs by displaying synchronized lyrics on the screen. These apps typically provide a library of instrumental tracks or karaoke versions of songs, enabling users to record their performances and often add special audio effects like reverb or pitch correction.
Many karaoke apps also feature social sharing options, allowing users to share their recordings with friends or a broader community. Karaoke apps have gained popularity for their ability to offer a fun, interactive, and social music experience on the go.
Must-Have Features for Karaoke Apps
When developing a karaoke app, certain must-have features are essential to create an engaging and enjoyable experience for users. Here are some of the key features that should be included:
- Vast Song Library: A large collection of songs from various genres and languages is crucial to appeal to a broad user base.
- Lyric Synchronization: The app should display lyrics in sync with the music, making it easy for users to follow along and sing in time.
- Voice Recording & Playback: Users should be able to record their performances and play them back to hear how they sound.
- Audio Effects: Include features like pitch correction, reverb, and echo effects to enhance users’ singing and make it sound more professional.
- Duet Mode: Allows users to sing along with friends or other users in real time, either remotely or in the same space.
- Social Sharing: Users should be able to share their recordings easily on social media or within the app’s community to showcase their performances.
- Customizable Avatars or Videos: Some apps let users create fun avatars or add video effects during their performances to personalize their experience.
- Live Performances: Live streaming capabilities for users to broadcast their karaoke sessions to friends or a wider audience.
- Offline Mode: Allow users to download songs and perform offline, which enhances accessibility when an internet connection is unavailable.
- Rating and Feedback System: Enabling users to rate each other’s performances or provide feedback fosters community engagement and growth within the app.
How Does the Karaoke App Work
A karaoke app allows users to sing along to music tracks, record their performances, and sometimes share or stream their performances with others. Here’s how it typically works:
1. Song Library and Lyrics Display
The app offers a wide selection of songs, allowing users to browse by category, search by keywords, or explore recommendations. Once a song is selected, the app plays a backing track and displays synchronized lyrics in karaoke style, enabling users to sing along easily.
2. Recording and Playback
The app uses the device’s microphone to capture the user’s voice while playing the backing track. Advanced features often let users adjust the pitch, tempo, or even remove the original vocals entirely for a purely instrumental experience.
3. Real-Time Scoring and Feedback
Some karaoke apps provide real-time feedback by analyzing the user’s pitch, rhythm, and overall performance using AI algorithms. This adds an element of gamification, encouraging users to improve their singing.
4. Recording and Sharing
Users can record their performances and save them locally or to the cloud for replay and editing. Many apps also support video recording, enabling users to create music videos while singing. After recording, users can share their creations on social media or within the app’s community.
5. Social and Interactive Features
Most karaoke apps include social elements, such as inviting friends for duets, joining group singing sessions, or watching others perform live. Some apps feature virtual gifting, where viewers can send performers virtual items as a token of appreciation.
6. Premium Features and Subscriptions
Karaoke apps often use a freemium model, offering basic features for free while locking advanced functionalities, premium songs, or ad-free experiences behind a subscription or in-app purchases.
What Challenges of Online Karaoke Platforms
Online karaoke platforms have seen significant growth in recent years, offering users the ability to sing and share their performances with others from the comfort of their homes. However, there are several challenges these platforms face in order to remain competitive, user-friendly, and scalable. Here are some of the key challenges:
1. Audio and Video Quality
- Latency and Lag: One of the biggest challenges for online karaoke platforms is minimizing latency and lag, which can disrupt the timing between the user’s singing and the music or lyrics. This is particularly important in live karaoke sessions or virtual karaoke parties.
- Audio Clarity: Ensuring high-quality, distortion-free audio in real-time is essential for a great user experience. This can be difficult, especially when the user’s environment includes background noise or if they are using low-quality microphones.
- Video Resolution: In video-based karaoke platforms, providing smooth, high-definition video streaming is crucial. Poor video quality can lead to a negative user experience, especially in group settings.
2. Noise Cancellation and Background Interference
- Background Noise: Many users may sing in noisy environments (like crowded homes, public spaces, or open-air locations), which can interfere with the audio experience. Platforms need to implement robust AI noise reduction solutions to eliminate unwanted background noise.
- Voice Clarity: Karaoke platforms often rely on real-time audio processing, which requires balancing the suppression of external noise without affecting the user’s vocal clarity. Achieving this balance is a challenge, particularly when users have varying audio setups and environments.
3. User Engagement and Retention
- Personalization: Offering personalized song recommendations and creating tailored karaoke experiences can help retain users, but this requires sophisticated algorithms and understanding of user preferences.
- Gamification and Competition: To keep users engaged, many karaoke platforms incorporate game-like elements, such as scoring, leaderboards, and challenges. Designing these features in a way that appeals to diverse users can be difficult.
- Social Features: Integrating social features, such as the ability to share performances, invite friends to sing, or host live karaoke parties, is key for maintaining user interest. Balancing these features with privacy and safety concerns is a challenge.
4. Licensing and Copyright Issues
- Song Licensing: Karaoke platforms need to secure licenses for the vast array of songs available on their platform. Navigating the complex legal requirements for music distribution, ensuring they have the rights to stream karaoke tracks, and managing royalty payments can be a significant challenge, especially when expanding to different regions.
- User-Generated Content: As platforms allow users to upload their performances or create custom karaoke tracks, managing copyright infringement becomes more complicated. Platforms need mechanisms to handle unauthorized content and protect the rights of original artists.
5. Scalability and Infrastructure
- Server Load and Traffic: Online karaoke platforms often experience spikes in traffic, particularly during peak times or special events. Ensuring that the infrastructure can handle large numbers of simultaneous users without compromising performance or causing crashes is a major challenge.
- Real-Time Interaction: For platforms that allow users to sing live together, maintaining synchronization between participants in different locations is crucial. Ensuring that all users have a seamless experience, without delays or connection issues, requires robust real-time streaming technology and server architecture.
6. Monetization
- Free vs. Paid Content: Many karaoke platforms operate on a freemium model, offering basic services for free and premium features for paid subscribers. Balancing the offerings between free and paid users, while ensuring that premium content adds enough value, can be a tricky task.
- Ad Integration: For free users, advertising can be a significant revenue stream. However, ads can disrupt the user experience if not implemented carefully. Finding the right balance between monetization through ads and user experience is essential.
- Virtual Goods and Tips: Some platforms enable users to purchase virtual goods or tip performers, but designing a system that feels rewarding for both performers and viewers can be complex and requires careful thought.
7. User Interface and Experience
- Intuitive Design: Karaoke platforms must offer a simple, user-friendly interface that enables users to quickly find songs, start singing, and navigate through various features without frustration.
- Mobile Optimization: With many users accessing karaoke platforms through mobile devices, ensuring the platform is optimized for smaller screens and touch controls is important for providing a smooth experience.
- Song Library Searchability: A large song catalog can overwhelm users. Having an intuitive and efficient search function that allows users to easily find songs by title, genre, artist, or mood is essential for user satisfaction.
8. Global Reach and Localization
- Cultural Preferences: Karaoke is a global activity, but song preferences can vary significantly by region. Platforms must consider offering localized content to meet the needs of users in different countries, including a diverse selection of languages, genres, and regional hits.
- Language Barriers: In order to serve international audiences, platforms need to provide accurate translations of song lyrics and UI elements, which can be a significant challenge, especially for live events or real-time collaboration.
9. User Safety and Privacy
- Protecting Personal Information: As online karaoke platforms involve social interaction and sometimes video sharing, user privacy and data protection are important concerns. Ensuring compliance with privacy regulations (such as GDPR) and providing users with control over their data are critical.
- Moderation of Content: Platforms need to implement robust content moderation systems to prevent inappropriate behavior, hate speech, or offensive content during live karaoke sessions or in user-uploaded videos.
10. Technical Support and Troubleshooting
- Device Compatibility: Users may access karaoke platforms from a variety of devices (e.g., mobile phones, tablets, desktops, smart TVs), each with its own technical requirements and limitations. Providing technical support to ensure the platform runs smoothly across all devices can be a challenge.
- Troubleshooting User Issues: Users may face issues such as connectivity problems, audio delays, or poor-quality video. Offering responsive customer support and self-help options is essential to keeping users satisfied.
ZEGOCLOUD Online Karaoke Solution
ZEGOCLOUD offers a comprehensive solution for building online karaoke features, enabling you to integrate song ordering, solo singing, sing-in-turns, and real-time chorus functionalities into your app. With access to over 2 million songs, all licensed and updated regularly, you can deliver a rich, engaging karaoke experience with guaranteed copyright compliance.
Key Features of ZEGOCLOUD’s Karaoke Solution
1. Diverse Karaoke Use Cases
ZEGOCLOUD supports various karaoke formats, allowing you to cater to different user preferences:
- Solo Karaoke: A single singer performs a whole song.
- Sing-in-Turns: Multiple users take turns singing parts of the song.
- Real-time Chorus: Users sing together in real-time, creating a shared musical experience.
These use cases can be easily incorporated into platforms like:
- Voice chat rooms: Users pay for hosts to sing through virtual gifts.
- Dating platforms: Users can sing together to break the ice.
- Live streaming: Hosts perform in turns, while the audience interacts via virtual gifts and feedback.
2. Extensive Music Library with 2+ Million Songs
ZEGOCLOUD provides access to a vast collection of 2 million+ songs and accompaniment tracks, updated regularly to keep up with trends. Key features include:
- Timely song updates: Always access the latest hits, including popular tracks from mainstream platforms and short-video apps.
- Flexible Licensing: Pay based on the number of users or songs accessed, making it more cost-effective than traditional bulk licensing.
- Copyright Compliance: The entire song library is licensed through a partnership with Tencent Music Entertainment Group, ensuring legal protection.
3. Customizable Functional Modules
ZEGOCLOUD offers a variety of ready-to-use modules that allow for customized user experiences:
- Scoring: Automatically scores singing performances to enhance user engagement.
- Synchronized Lyrics: Display lyrics word-by-word or line-by-line in real-time, synchronized with the music.
- Culmination-Only Singing: Users can opt to sing only the key parts of a song.
How to Do Online Karaoke using ZEGOCLOUD SDK
Transform your audio experience with ZEGOCLOUD’s comprehensive UIKits. Designed for developers, UIKIts is a powerful toolkit to create real-time audio applications like online music concerts and meetings. It boasts a range of features including multiple audio stream support, effective echo cancellation, and easy integration with other systems, making audio room management a breeze.
If you are looking for how to do online karaoke, ZEGOCLOUD UIkits would allow you to finish app-building in low code. It includes Live Audio Room Kit SDK that is special for karaoke online. Unlock a world of musical possibilities with the Live Audio Room Kit SDK for online karaoke. Here’s a sneak peek of what you can expect:
1. Music and lyrics customization
With the Live Audio Room Kit SDK, you have the ability to add background music to your karaoke performances and customize the lyrics display to suit your personal style. This means you can create karaoke videos that truly reflect your musical preferences.
2. User-friendly interface
The SDK has a simple and intuitive interface that makes it easy for users to create and customize their karaoke videos. With its straightforward design, you don’t have to be a tech-savvy person to use it effectively.
3. Personalized customization
The Live Audio Room Kit SDK allows you to tailor your karaoke videos to your individual preferences. This means you can experiment with different styles and make your videos truly unique.
4. High-quality output
The videos you create with this tool will have excellent sound and visual quality, making it suitable for sharing online or watching on any device. Whether you’re watching on your TV or on a computer, you can expect a top-notch viewing experience.
Steps to Create Online Karaoke App Free
Preparation
- Get a developer account with ZEGOCLOUD.
- Configure Flutter on your computer.
- Use an Android or iOS device with audio and internet support.
- Go to ZEGOCLOUD Admin Console.
- Create a new project and record the AppID and AppSign.
- Enable the In-app Chat service.
To make online karaoke with the Live Audio Room Kit SDK:
- In the project’s root directory, execute the code below to add
ZegoUIKitPrebuiltLiveAudioRoom
:
flutter pub add zego_uikit_prebuilt_live_audio_room
- In your
main.dart
file, import the SDK with this line:
import 'package:zego_uikit_prebuilt_live_audio_room/zego_uikit_prebuilt_live_audio_room.dart';
You’re now ready to create an amazing online karaoke experience!
How to Use the Live Audio Room Kit for Online Karaoke Free
To join a Live Audio Room with the Live Audio Room Kit, you’ll need to have your userID
and userName
handy. The specific live audio room you want to participate in is identified by its roomID
.
Here’s how to get started with the Live Page class in your main.dart
file:
class LivePage extends StatelessWidget {
final String roomID;
final bool isHost;
const LivePage({Key? key, required this.roomID, this.isHost = false}) : super(key: key);
@override
Widget build(BuildContext context) {
return SafeArea(
child: ZegoUIKitPrebuiltLiveAudioRoom(
appID: yourAppID, // Add your appID from the ZEGOCLOUD Admin Console.
appSign: yourAppSign, // Add your appSign from the ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
roomID: roomID,
config: isHost
? ZegoUIKitPrebuiltLiveAudioRoomConfig.host()
: ZegoUIKitPrebuiltLiveAudioRoomConfig.audience(),
),
);
}
}
Get ready to join a live audio room and enjoy singing your heart out!
Android project configuration
Take the following steps below to configure your Android project in Flutter:
- Boost your Android app’s performance by setting the
compileSdkVersion
to33
inyour_project/android/app/build.gradle
. - Ensure your app has the necessary permissions by adding these lines to
app/src/main/AndroidManifest.xml
:
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
- Protect your SDK from obfuscation by creating a file called
proguard-rules.pro
inandroid > app
and adding the line-keep <strong>class</strong> .<strong>zego</strong>. { *; }
. Also, add the following code in the release section ofyour_project/android/app/build.gradle
:
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
iOS project configuration
1. Granting Access: In your_project/ios
directory, open the Podfile and add the following code to the post install section to enable camera and microphone permissions:
# Permission Configuration
target.build_configurations.each do |config|
config.build_settings['GCC_PREPROCESSOR_DEFINITIONS'] ||= [
'$(inherited)',
'PERMISSION_CAMERA=1',
'PERMISSION_MICROPHONE=1',
]
End
This will allow your app to smoothly request access to the camera and microphone when necessary.
2. Providing Information: To give users more clarity on why your app requires camera and microphone access, navigate to the Info.plist
file in the your_project/ios/Runner
directory and add the following code to the dict
section:
<key>NSCameraUsageDescription</key>
<string>This app needs camera access to join a live audio session.</string>
<key>NSMicrophoneUsageDescription</key>
<string>This app needs microphone access to participate in a live audio session.</string>
This will let your users know why the app needs these resources and increase transparency.
3. Enhancing Performance: To improve the app’s performance and efficiency, disable Bitcode by opening the Runner.xcworkspace
file in your_project > iOS
directory, and selecting your target project.
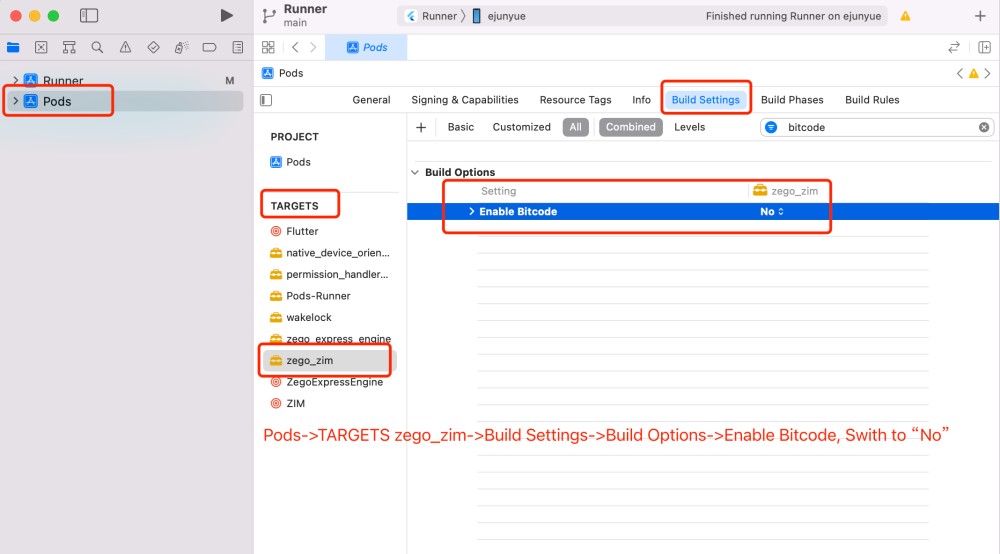
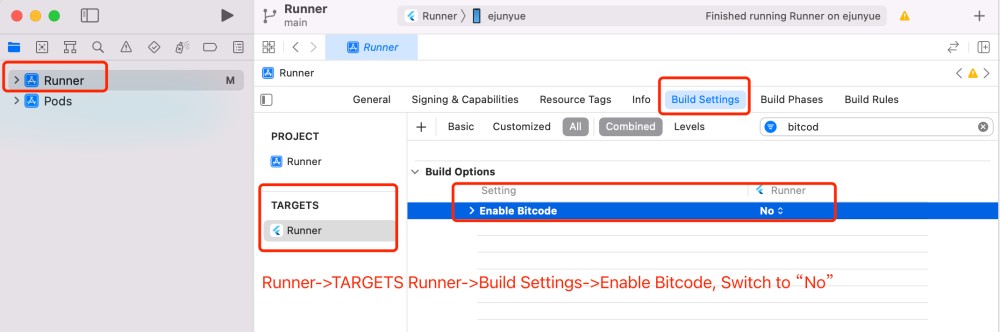
With these three steps, you are all set to join live audio sessions seamlessly.
Run a Demo
With our demo source code, you’ll have everything you need to get started on your project.
ZEGOCLOUD SDK provides an effortless way to enjoy free online karaoke, with its user-friendly interface and advanced features. Sing with friends and family from the comfort of your home.
Whether you’re a seasoned karaoke pro or just starting out, ZEGOCLOUD SDK makes it easy to get started with online karaoke for free. So why wait? Give it a try and experience the fun and excitement of online karaoke for yourself!
How Much It Cost to Develop A Karaoke App
The cost to develop a karaoke app varies widely based on the app’s complexity, desired features, and the location of the development team. For a basic karaoke app with essential features like a song library, recording, and playback, you might expect to spend between $20,000 and $50,000. If you’re looking to add more sophisticated features such as an extensive song library, real-time lyrics, social sharing, and basic audio effects, the cost could rise to between $50,000 and $100,000.
For a highly advanced karaoke app that includes features like live streaming, duets, advanced audio effects, in-app purchases, and integration with third-party music services, the development cost could exceed $100,000, potentially reaching up to $300,000 or more.
Additionally, you should consider ongoing costs for maintenance, updates, and scaling, which typically add 15% to 20% of the initial development cost each year. Marketing and promotion efforts, crucial for user acquisition and growth, will also require a separate budget, the size of which will depend on your overall strategy.
In summary, developing a karaoke app can cost anywhere from $20,000 to over $300,000, depending on the features and complexity, with additional ongoing costs for maintenance and marketing.
How to Monetize with Karaoke Apps
Monetizing karaoke apps involves creating revenue streams that capitalize on the app’s user base and features. Here are several strategies to consider:
- In-App Purchases: Offer users the option to buy premium content, such as exclusive songs, advanced vocal effects, or additional song downloads. This model allows users to customize their experience according to their preferences and willingness to pay.
- Subscription Model: Implement a subscription model that provides access to an expanded library of songs, an ad-free experience, and premium features like high-quality audio or video recording capabilities. Subscriptions encourage ongoing revenue and user engagement.
- Advertisements: Integrate ads into the app, such as banner ads, video ads, or sponsored content. Offering an ad-supported free version can attract a wide user base while providing the option to upgrade to an ad-free version can generate additional revenue.
- Partnerships and Sponsorships: Collaborate with music labels, artists, and brands to feature exclusive content or sponsored playlists. This not only provides users with unique content but also opens up revenue streams through partnerships.
- Merchandising: Sell branded merchandise within the app, such as themed accessories, apparel, or even karaoke equipment. This can be particularly effective if your app has a strong brand identity or a loyal user base.
- Virtual Events and Competitions: Host virtual karaoke competitions or events with entry fees or sponsored prizes. This can increase user engagement and provide an opportunity for additional income through participation fees or sponsorship deals.
- Affiliate Marketing: Use affiliate marketing to recommend products related to music or karaoke, such as microphones, headphones, or music subscriptions, and earn commissions on sales through the app.
Conclusion
In conclusion, karaoke app development requires a careful balance of technology, design, and user experience to create an app that stands out in a competitive market. By focusing on real-time communication, high-quality audio processing, and intuitive features like lyric synchronization and performance sharing, developers can create an engaging platform that appeals to a wide audience. As the demand for interactive entertainment continues to grow, building a well-optimized karaoke app presents a promising opportunity for success in the app industry.
Read more:
FAQ
Q1: How to develop a karaoke app?
Develop a karaoke app by researching the market, planning features like a song library, real-time lyrics, and pitch correction, and securing music licensing. Use technologies such as audio SDKs for streaming and voice effects. After testing for compatibility, launch and market the app effectively.
Q2: What karaoke app has pitch analysis?
Apps like Smule, Perfect Pitch, and Pitchy Ninja offer pitch analysis, providing real-time feedback and vocal scoring to help users improve their singing.
Q3: What karaoke software do bars use?
Bars commonly use software like KaraFun, Pioneer Karaoke, and Kanto Karaoke for their extensive song libraries, offline features, and professional playback capabilities.
Q4: What is the karaoke app that pays people?
Apps like StarMaker, Kumu, and Sessions Live allow singers to earn money through virtual gifts, tips, and live performances.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!