Building seamless multi-user video calls is a cornerstone of modern communication applications. This guide walks you through the process of implementing a video call system that supports multiple users, allowing them to publish and play video streams simultaneously. Whether you’re designing a collaborative workspace, a virtual event platform, or a social app, this guide provides best practices and practical steps to bring your multi-user video call scenario to life.
Prerequisites
Before you begin, make sure you complete the following steps:
- You have created a project in ZEGOCLOUD Console, and get the valid AppID and AppSign. For details, please refer to Admin Console – How to view project information.
- Your project has integrated ZEGO Express SDK , and your project has implemented basic real-time audio and video features. For details, please refer to Integrate the SDK and Implement a basic video call.
Implementation process of multi-user video calls
The following diagram shows the basic process of the video call for multiple users:
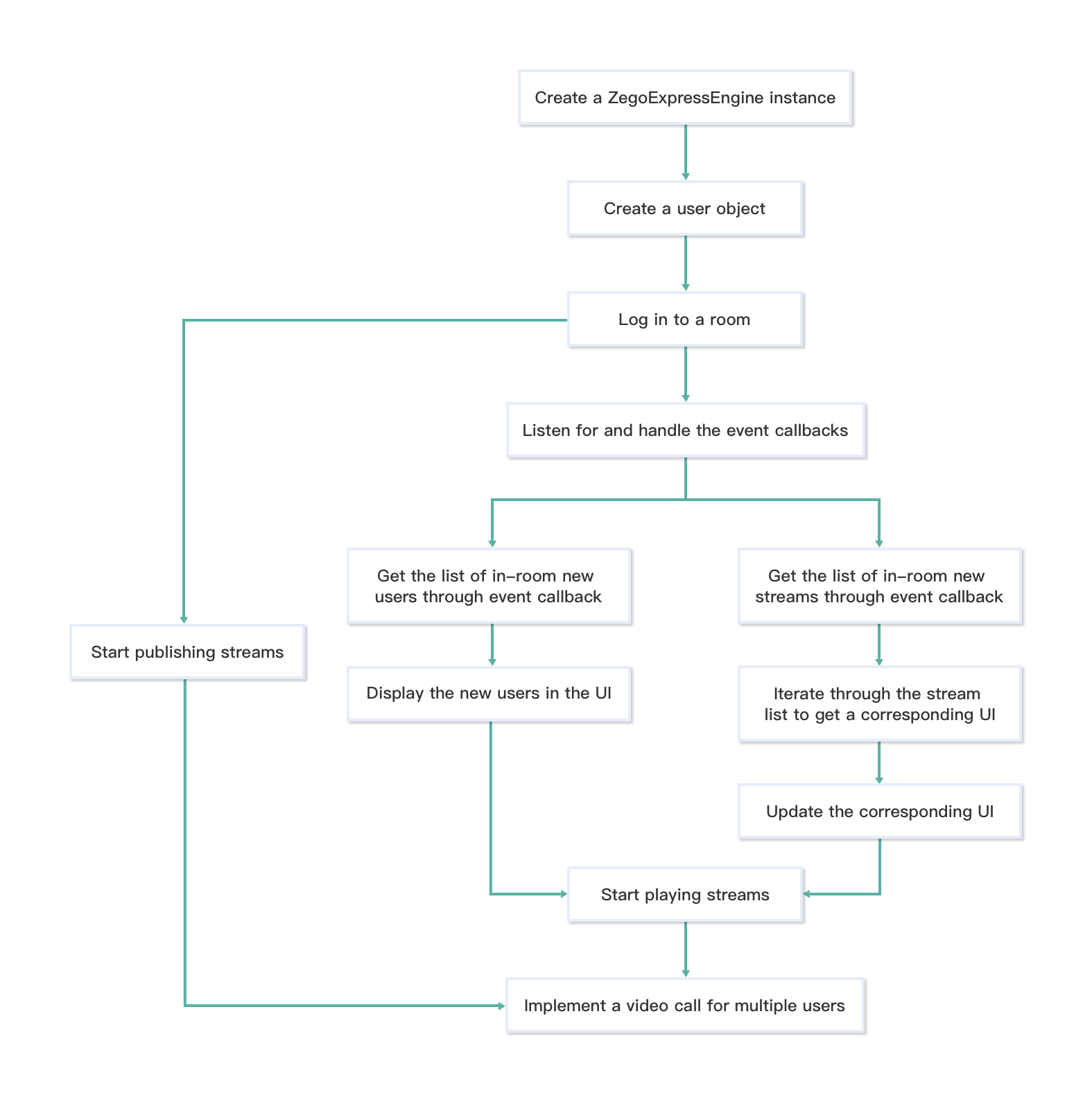
Create a ZegoExpressEngine
instance
To create a singleton instance of the ZegoExpressEngine class, call the createEngine
method with the AppID of your project.
To receive event callbacks, implement an event handler object that conforms to the IZegoEventHandler
protocol, and then pass the implemented event handler object to the createEngine
method as the eventHandler
parameter.
Alternatively, you can pass null
to the createEngine
method as the eventHandler
parameter for now, and then call the method setEventHandler
to set up the event handler after creating the engine.
/** Define a ZegoExpressEngine object */
ZegoExpressEngine engine;
ZegoEngineProfile profile = new ZegoEngineProfile();
/** AppID format: 123456789L */
profile.appID = appID;
/** General scenario */
profile.scenario = ZegoScenario.GENERAL;
/** Set application object of App */
profile.application = getApplication();
/** Create a ZegoExpressEngine instance */
engine = ZegoExpressEngine.createEngine(profile, null);
Enable the event notification
To receive the event notification that other users join or leave the room, you must set the isUserStatusNotify
property of the room configuration parameter ZegoRoomConfig
to true
every time the user logs in to a room.
ZegoRoomConfig RoomConfig = new ZegoRoomConfig();
RoomConfig.isUserStatusNotify = true;
// Log in to a room.
engine.loginRoom(roomID, user, RoomConfig);
Listen for and handle the event callbacks
To implement the multi-user video call feature, you need to listen for event callbacks related to room users and streams. Additionally, you must handle these callbacks to ensure smooth functionality. To listen for and handle the related event callbacks, call the setEventHandler
method.
To receive the event notification for updates on the status of other users in the room, call the onRoomUserUpdate
method. And when other users join or leave the room, the SDK sends out the event notification through this callback.
The updateType
parameter in the callback onRoomUserUpdate
indicates the type of changes, details are listed below:
Change type | Enumerated values | Description |
User joins | ZegoUpdateType.ADD | New users join the room. The userList indicates the list of new users. |
User leaves | ZegoUpdateType.DELETE | Users leave the room. The userList indicates the list of users who have logged out. |
When a user joins a room for the first time, if there are already other users in the room, the new user will receive a user list (the event notification when updateType
is ZegoUpdateType.ADD
) through this callback, which includes the existing users in the room.
- To receive the onRoomUserUpdate callback, you must set the
isUserStatusNotify
property of the room configuration parameter ZegoRoomConfig totrue
when you call the loginRoom method to log in to a room. - This callback turns invalid when there are more than 500 users in the room.
engine.setEventHandler(new IZegoEventHandler() {
//Callback for the updates on the status of other users in the room (other users join or leave the room).
//The callback turns invalid when there are more than 500 users in the room.
@Override
public void onRoomUserUpdate(String roomID, ZegoUpdateType updateType, ArrayList<ZegoUser> userList) {
super.onRoomUserUpdate(roomID, updateType, userList);
// Update the UI or implement other operations here.
}
}
To receive notifications about updates to stream statuses in the room, use the onRoomStreamUpdate
callback. When new streams are published in the room, the SDK triggers this callback to send a notification. Similarly, it notifies you through the same callback when streams are removed.
The updateType
parameter in the callback onRoomStreamUpdate
indicates the type of changes, details are listed below:
Change type | Enumerated values | Description |
New stream publishes | ZegoUpdateType.ADD | New streams published to the room. The streamList indicates the list of newly published streams.| |
Existing stream stops | ZegoUpdateType.DELETE | Existing streams in the room stop. The streamList indicates the list of stopped streams. |
When a user joins a room for the first time, if there is stream publishing ongoing, the new user will receive a stream list (the event notification when updateType
is ZegoUpdateType.ADD
) through this callback, which includes the newly published streams in the room.
engine.setEventHandler(new IZegoEventHandler() {
// Callback for the updates on the status of the streams in the room (new streams published to the room or existing streams in the room stop).
@Override
public void onRoomStreamUpdate(String roomID, ZegoUpdateType updateType, ArrayList<ZegoStream> streamList, JSONObject extendedData) {
super.onRoomStreamUpdate(roomID, updateType, streamList, extendedData);
// Update the UI or implement other operations here.
}
}
Start publishing/playing a stream
To start the stream publishing and playing, see Implement a basic video call
API call sequence diagram
The following diagram shows the API call sequence of the stream publishing and playing process:
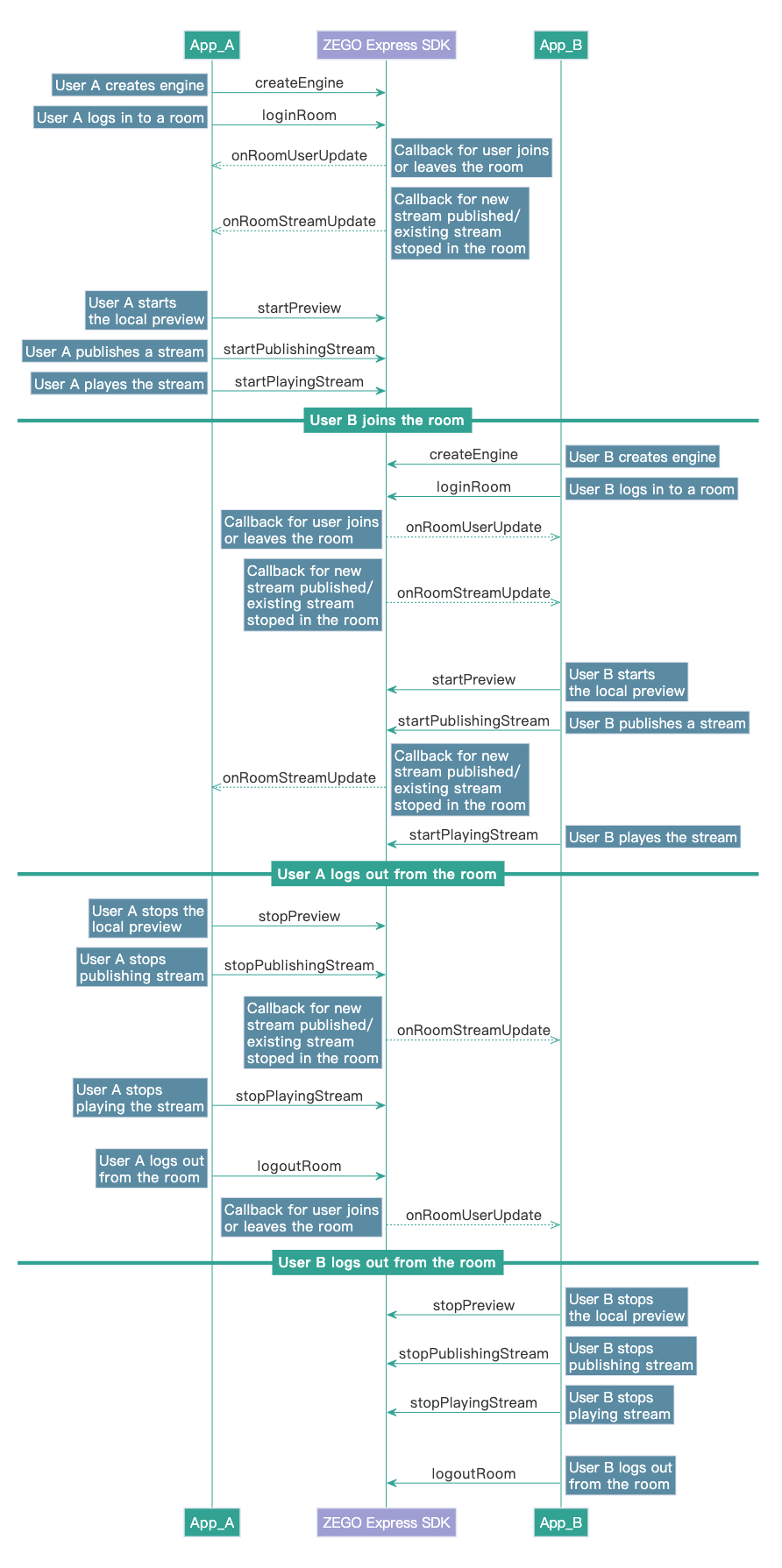
Conclusion
Implementing multi-user video calls is a vital feature for any modern communication app, empowering seamless collaboration and real-time interaction among users. By following this guide, you now have the foundation to set up a scalable and robust video call system based on ZEGOCLOUD Video Call. From initializing the ZegoExpressEngine to handling user and stream updates efficiently, this step-by-step process ensures you’re equipped to deliver high-quality video experiences.
With ZEGOCLOUD’s powerful SDK and intuitive tools, you can build engaging applications that cater to diverse user needs. Start integrating multi-user video calls into your app today and unlock new possibilities for connection and innovation in real-time communication. Sign up today and enjoy 10,000 minutes free of charge.
Let’s Build APP Together
Start building with real-time video, voice & chat SDK for apps today!