Quick start
This doc will guide you to integrate the In-app Chat Kit and start a chat quickly.
Prerequisites
- Go to ZEGOCLOUD Admin Console and do the following:
- Create a project, and get the
AppID
andAppSign
of your project. - Activate the In-app Chat service.
- Create a project, and get the
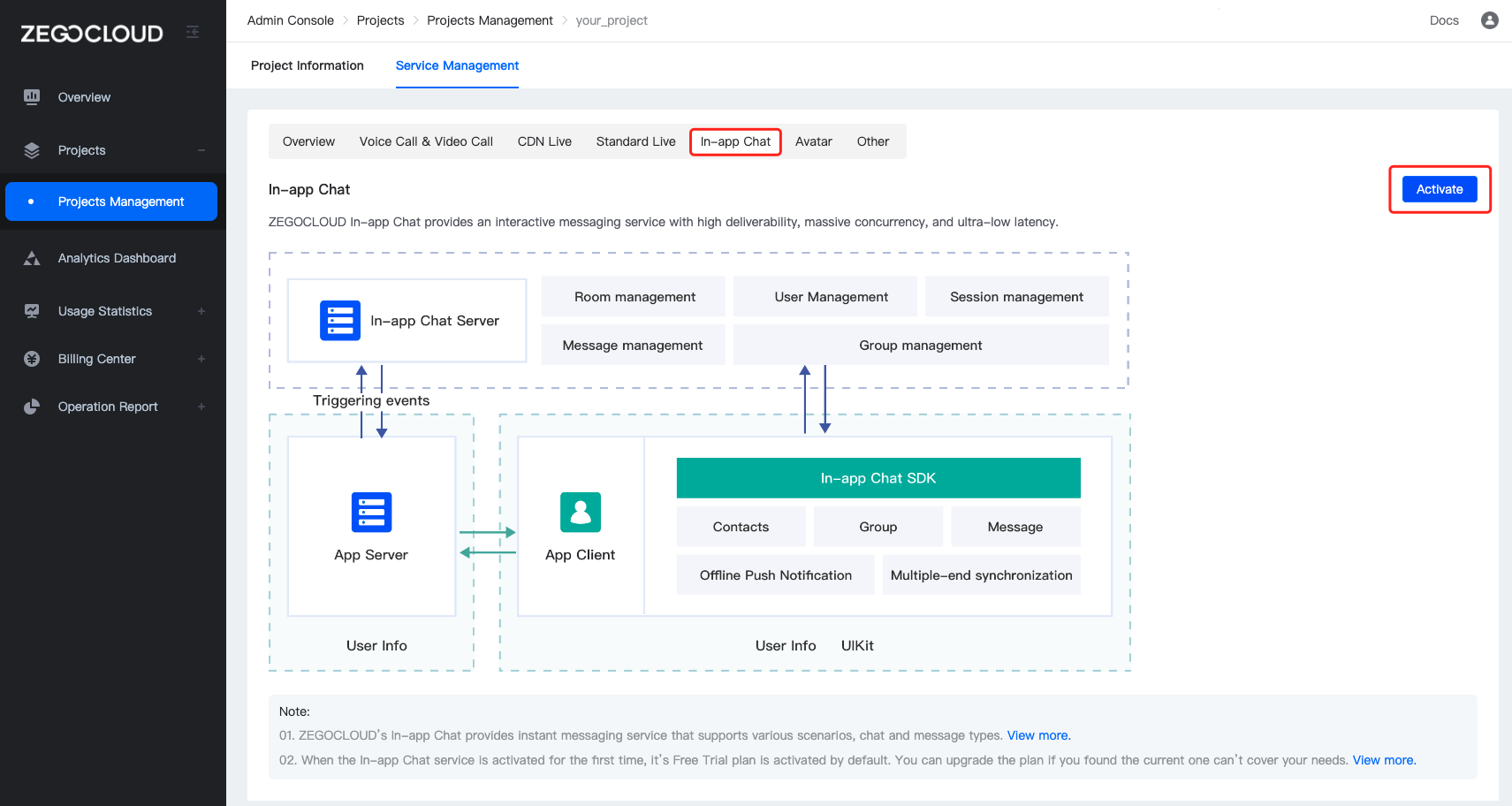
- Environment-specific requirements:
- Android Studio Arctic Fox (2020.3.1) or later
- Android SDK Packages: Android SDK 30, Android SDK Platform - Tools 30
- An Android device or Simulator that is running on Android 5.0 or later and supports audio and video. We recommend you use a real device.
- Android device and your computer are connected to the internet.
Integrate the SDK
1
Add com.github.ZEGOCLOUD:zego_inapp_chat_uikit_android as dependencies
- Add the
jitpack
configuration
- Modify your app-level
build.gradle
file
Untitled
dependencies {
...
implementation 'com.github.ZEGOCLOUD:zego_inapp_chat_uikit_android:+' // add this line in your module-level build.gradle file's dependencies, usually named [app].
}
1
2
Call the init method to initialize the In-app Chat Kit
MyApplication.java
import android.app.Application;
import com.zegocloud.zimkit.services.ZIMKit;
public class MyApplication extends Application {
public static MyApplication sInstance;
@Override
public void onCreate() {
super.onCreate();
sInstance = this;
Long appId = ; // The AppID you get from ZEGOCLOUD Admin Console.
String appSign = ; // The App Sign you get from ZEGOCLOUD Admin Console.
ZIMKit.initWith(this,appId,appSign);
// Online notification for the initialization (use the following code if this is needed).
ZIMKit.initNotifications();
}
}
1
3
Call the connectUser method on the login page to log in to the In-app Chat Kit.
Warning
You can customize rules to generate the user ID and user name. We recommend that you set a meaningful user ID. You can associate the user ID with your business account system.
MyZIMKitActivity.java
import android.content.Intent;
import android.os.Bundle;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import im.zego.zim.enums.ZIMErrorCode;
import com.zegocloud.zimkit.services.ZIMKit;
public class MyZIMKitActivity extends AppCompatActivity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
public void buttonClick() {
// userId and userName: 1 to 32 characters, can only contain digits, letters, and the following special characters: '~', '!', '@', '#', '$', '%', '^', '&', '*', '(', ')', '_', '+', '=', '-', '`', ';', '’', ',', '.', '<', '>', '/', '\'
String userId = ; // Your ID as a user.
String userName = ; // You name as a user.
String userAvatar = ; // The image you set as the user avatar must be network image. e.g., https://storage.zego.im/IMKit/avatar/avatar-0.png
connectUser(userId, userName,userAvatar);
}
public void connectUser(String userId, String userName,String userAvatar) {
// Logs in.
ZIMKit.connectUser(userId,userName,userAvatar, errorInfo -> {
if (errorInfo.code == ZIMErrorCode.SUCCESS) {
// Operation after successful login. You will be redirected to other modules only after successful login. In this sample code, you will be redirected to the conversation module.
toConversationActivity();
} else {
}
});
}
// Integrate the conversation list into your Activity as a Fragment
private void toConversationActivity() {
// Redirect to the conversation list (Activity) you created.
Intent intent = new Intent(this,ConversationActivity.class);
startActivity(intent);
}
}
1
4
Display the conversation component of the In-app Chat Kit
The layout of the ConversationActivity
is specified in activity_conversation.xml
:
ConversationActivity.java
activity_conversation.xml
public class ConversationActivity extends AppCompatActivity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_conversation);
}
}
1
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<fragment
android:id="@+id/frag_conversation_list"
android:name="com.zegocloud.zimkit.components.conversation.ui.ZIMKitConversationFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
1
Ideally, by this point, your app will look like this:
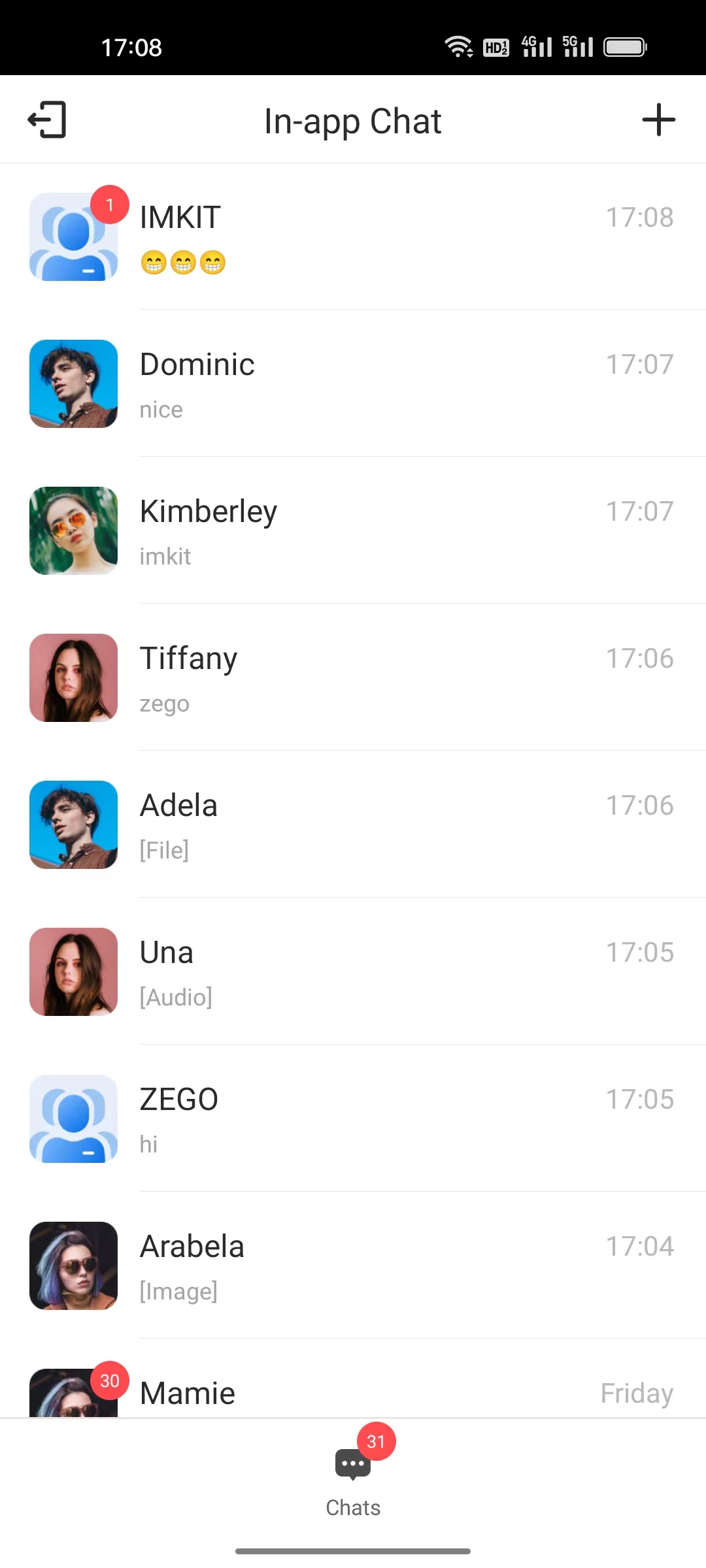
Start a chat
The In-app Chat Kit supports the following features:
Related guides
Component overview
Click here to explore more UI components.
Run the sample code
A quick guide to help you run the sample code.
Get support
Need help or want to raise your questions? Click the button below to join our Discord community to get quick responses.