Use with Call Kit
This doc will introduce how to use In-app Chat Kit with Call Kit.
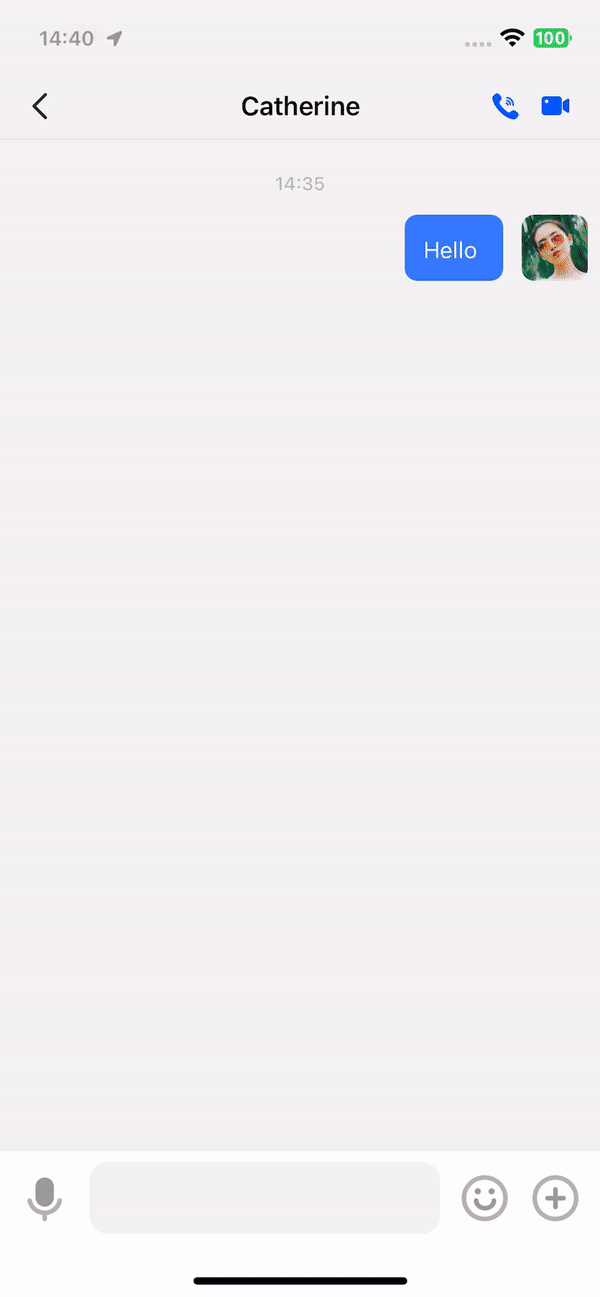
Prerequisites
Integrate the In-app Chat Kit SDK into your project. For more information, see Quick start.
Integrate the Call Kit
Add Call Kit dependency
- Add @zegocloud/zego-uikit-prebuilt-call-rn as dependencies.
yarn add @zegocloud/zego-uikit-prebuilt-call-rn
npm install @zegocloud/zego-uikit-prebuilt-call-rn
- Add other dependencies.
Run the following command to install other dependencies for making sure the @zegocloud/zego-uikit-prebuilt-call-rn
can work properly.
yarn add @zegocloud/zego-uikit-rn react-delegate-component @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-sound react-native-encrypted-storage zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.12.1 zego-zpns-react-native@2.5.0-alpha react-native-keep-awake@4.0.0
npm install @zegocloud/zego-uikit-rn react-delegate-component @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-sound react-native-encrypted-storage zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.12.1 zego-zpns-react-native@2.5.0-alpha react-native-keep-awake@4.0.0
Initialize the Call Kit
To receive the call invites from others and let the calling notification show on the top bar when receiving it, you will need to initialize the call invitation.
- Config React Navigation
- Place the
ZegoCallInvitationDialog
component on the top level of theNavigationContainer
.
// App.js
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import AppNavigation from './AppNavigation';
// Add this line \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
import { ZegoCallInvitationDialog } from '@zegocloud/zego-uikit-prebuilt-call-rn';
// Add this line \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
export default function App() {
return (
<NavigationContainer>
// Add this line \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
<ZegoCallInvitationDialog />
// Add this line \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
<AppNavigation />
</NavigationContainer>
);
}
- Add two routes (
ZegoUIKitPrebuiltCallWaitingScreen
andZegoUIKitPrebuiltCallInCallScreen
) to your stack navigator.
// AppNavigation.js
import { createNativeStackNavigator } from '@react-navigation/native-stack';
import { MessageListPage } from '@zegocloud/zimkit-rn';
import LoginPage from './LoginPage.js'; // Import the login page you created.
import HomePage from './HomePage.js'; // Import the In-app Chat home page you created.
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
import {
ZegoUIKitPrebuiltCallWaitingScreen,
ZegoUIKitPrebuiltCallInCallScreen,
} from '@zegocloud/zego-uikit-prebuilt-call-rn';
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
const Stack = createNativeStackNavigator();
export default function AppNavigation() {
return (
<Stack.Navigator initialRouteName="LoginPage">
<Stack.Screen
name="LoginPage" // Specify a page route for the login page.
component={LoginPage} // Fill in the imported login page.
/>
<Stack.Screen
name="HomePage" // Specify a page route for the In-app Chat home page.
component={HomePage} // Fill in the imported In-app Chat home page.
/>
<Stack.Screen
name="MessageListPage" // Specify a page route for the message list page.
component={MessageListPage} // Fill in the imported message list component.
/>
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
<Stack.Screen
options={{ headerShown: false }}
// DO NOT change the name
name="ZegoUIKitPrebuiltCallWaitingScreen"
component={ZegoUIKitPrebuiltCallWaitingScreen}
/>
<Stack.Screen
options={{ headerShown: false }}
// DO NOT change the name
name="ZegoUIKitPrebuiltCallInCallScreen"
component={ZegoUIKitPrebuiltCallInCallScreen}
/>
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
... // Other page info.
</Stack.Navigator>
);
}
- Initialize/Deinitialize the call invitation service.
Initialize the service when your app users logged in successfully or re-logged in after an exit.
// LoginPage.js
import { useEffect } from 'react';
import { useNavigation } from '@react-navigation/native';
import { ZIMKit } from '@zegocloud/zimkit-rn';
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
import * as ZIM from 'zego-zim-react-native';
import ZegoUIKitPrebuiltCallService from "@zegocloud/zego-uikit-prebuilt-call-rn";
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
export default function LoginPage() {
const navigation = useNavigation(); // Use the React Navigation for page routing.
const appConfig = {
appID: 0, // The AppID you get from ZEGOCLOUD Admin Console.
appSign: '', // The AppSign you get from ZEGOCLOUD Admin Console.
};
useEffect(() => {
ZIMKit.init(appConfig.appID, appConfig.appSign);
ZIMKit.connectUser({
userID: '', // Your ID as a user.
userName: '' // Your name as a user.
}, '')
.then(() => {
// Implement your event handling logic after logging in successfully.
// initialized ZegoUIKitPrebuiltCallInvitationService.
// when app's user is logged in or re-logged in.
// We recommend calling this method as soon as the user logs in to your app.
ZegoUIKitPrebuiltCallService.init(
appConfig.appID, // The AppID you get from ZEGOCLOUD Admin Console.
appConfig.appSign, // The AppSign you get from ZEGOCLOUD Admin Console.
userID, // Your ID as a user.
userName, // Your name as a user.
[ZIM],
);
// Navigate to the In-app Chat Kit page.
navigation.navigate('HomePage');
});
}, []);
}
Add a call button
Add a call button to the appBar
using the appBarActions
parameter of jump to the MessageListPage
:
// HomePage.js
import { useNavigation } from '@react-navigation/native';
import { SafeAreaView } from 'react-native';
import { ConversationList } from '@zegocloud/zimkit-rn';
export default function HomePage() {
const navigation = useNavigation(); // Use the React Navigation for page routing.
const goToMessageListPage = props => {
// Rount to the message list page via the React Navigation. For more, refer to: https://reactnavigation.org/
navigation.navigate('MessageListPage', {
...props,
// The callback method for the top button on the message list page.
// Add call button for peer chat
appBarActions: props.conversationType === 0 ? [
{
icon: 'goBack',
onPressed: () => {
navigation.goBack();
},
},
// Voice call button
() => <ZegoSendCallInvitationButton
invitees={[{userID: props.conversationID, userName: props.conversationName }]}
/>,
// Video call button
() => <ZegoSendCallInvitationButton
isVideoCall={true}
invitees={[{userID: props.conversationID, userName: props.conversationName }]}
/>
] : [{
icon: 'goBack',
onPressed: () => {
navigation.goBack();
},
}],
});
};
const onPressed = (props) => {
goToMessageListPage(props);
};
return (
<SafeAreaView style={{flex: 1}}>
<ConversationList onPressed={onPressed}></ConversationList>
</SafeAreaView>
);
}
So far, you have successfully integrated Call Kit in the In-app Chat Kit. You can now run and experience it.
More resources
The above content only introduces the basic configuration required for integrating Call Kit. If you need to further customize the configuration of the call, refer to the following documents:
Steps in this doc helps you to make a call quickly.
This article guides you on how to further customize the features and UI of the call.