Send and receive messages
This document describes how to use the ZIM SDK (In-app Chat) to send and receive messages.
Prerequisites
Before you begin, make sure:
- Go to ZEGOCLOUD Admin Console, and do the following:
- Create a project, get the AppID and AppSign.
- Activate the In-app Chat service (as shown in the following figure).
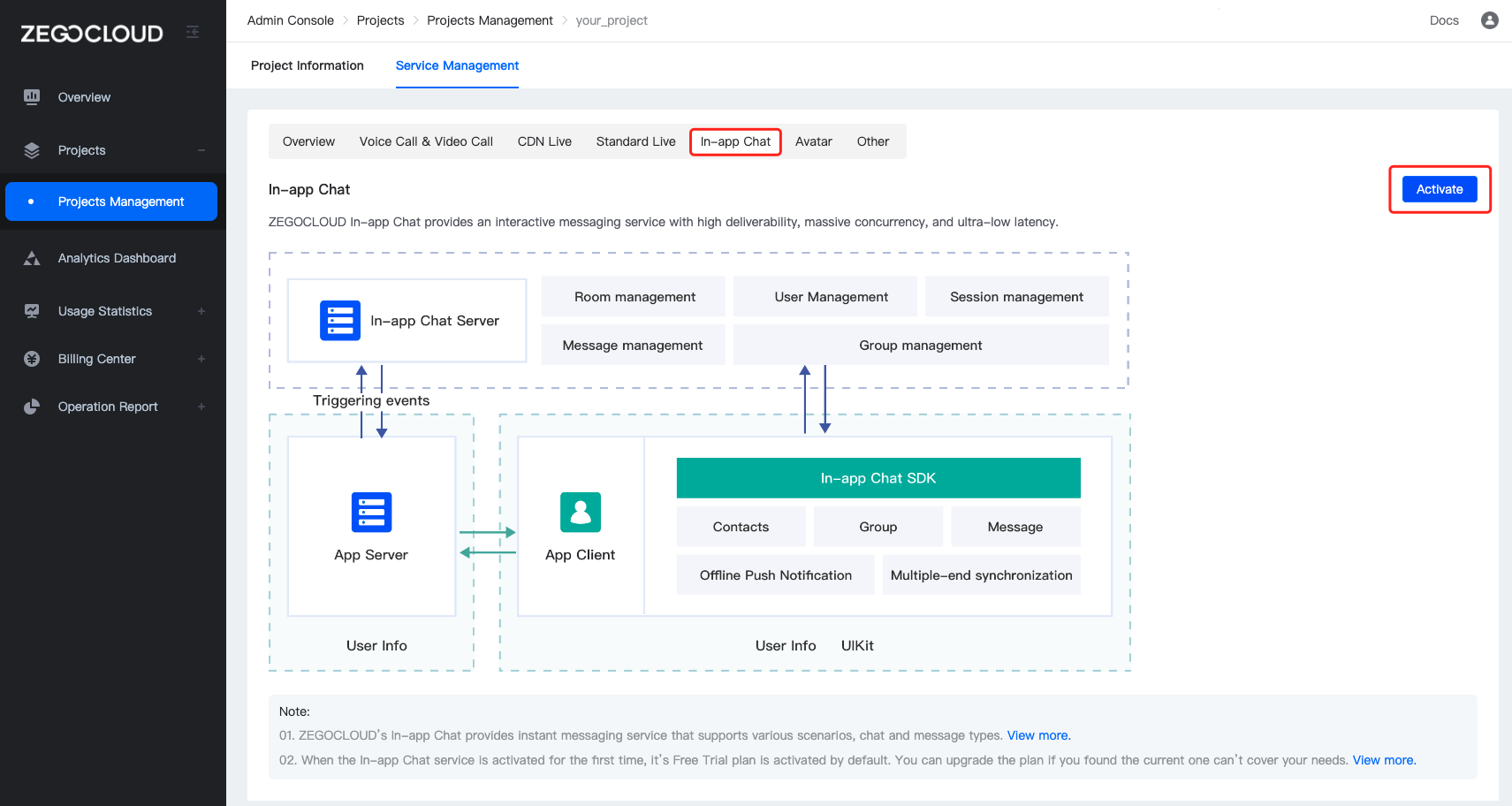
- Platform-specific requirements:
- React Native 0.60.0 or later
- A real iOS device that is running on iOS 11.0 or later and supports audio and video.
- An Android device or Simulator that is running on Android 4.0.3 or later and supports audio and video. We recommend you use a real device (enable the "USB Debugging" option).
- Your device is connected to the internet.
- Configure Visual Studio Code, search for the "React Native Tools" extension, and install it.
Integrate the SDK
Optional: Create a new project
Skip to this step if a project already exists.
Import the SDK
The following shows how to integrate the SDK using NPM:
-
Execute the
npm i zego-zim-react-native
oryarn add zego-zim-react-native
command to install the dependencies.NoteWe recommend you use the NPM package that supports the TypeScript language.
-
Import the SDK.
Untitledimport ZIM from 'zego-zim-react-native';
1 -
Go to the iOS root directory, run the
pod install
command to install the dependencies.
After completing the above steps, you can use the zego-zim-react-native
SDK in your project using JavaScript or TypeScript (recommended).
Implementation steps
Get the sample code
Get the sample code from Sample app.
Import the SDK file
Refer to the Integrate the SDK using NPM to import the SDK package file.
Create a ZIM SDK instance
Creating a ZIM instance is the very first step, an instance corresponds to a user logging into the system as a client.
So, let's suppose we have two clients now, client A and client B.
To send and receive messages to each other, both of them will need to call the create method with the AppID in the previous Prerequisites steps to create a ZIM SDK instance of their own:
// Since In-app Chat 2.3.0, the SDK uses the AppSign authentication mode by default. To change the authentication mode:
// 1. For SDK 2.3.3 or later, you can change the authentication mode by yourself. 2. For SDK 2.3.0 or later, if you want to change your authentication mode back to using the Token, contact Technical Support.
// Use a static synchronized method to create a ZIM SDK object and pass the AppID and AppSign.
// The create method creates a ZIM instance only the first time it is called. Any subsequent use will return null.
ZIM.create({ appID: 0, appSign: '' });
// Please get a single instance via the getInstance method to avoid hot updates that return null multiple times using the create method.
const zim = ZIM.getInstance();
Set event callbacks
Before a client user's login, you will need to call the on method to customize the event callbacks, such as you can receive callback notifications when SDK errors occur or receive message-related callback notifications.
// Set up and listen for the callback for receiving error codes.
zim.on('error', function (zim, errorInfo) {
console.log('error', errorInfo.code, errorInfo.message);
});
// Set up and listen for the callback for connection status changes.
zim.on('connectionStateChanged', function (zim, { state, event, extendedData }) {
console.log('connectionStateChanged', state, event, extendedData);
});
// Set up and listen for the callback for receiving one-to-one messages.
zim.on('peerMessageReceived', function (zim, { messageList, info, fromConversationID }) {
console.log('peerMessageReceived', messageList, info, fromConversationID);
});
// Register a callback to listen for "Token is about to expire"
zim.on('tokenWillExpire', function (zim, { second }) {
console.log('tokenWillExpire', second);
// You can call the renewToken interface here to update the token
// For new token generation, please refer to the above
zim.renewToken(token)
.then(function({ token }){
// Update successful
})
.catch(function(err){
// Update failed
})
});
For a detailed introduction to the interfaces, please refer to connectionStateChanged, peerMessageReceived, tokenWillExpire.
Log in to the ZIM SDK
For client A and client B to send, receive messages, and renew the token after creating the ZIM SDK instance, they will need to log in to the ZIM SDK.
To log in, Clients A and B both need to do the following:
- Call the ZIMLoginConfig method to create a user object.
- Then, call the [login](@ login__2) method with their own user information and the Token they get in the previous Prerequisites steps.
-
You can custom the userID and userName, and we recommend you set the userID to a meaningful value and associate them with the account system of your application.
-
For SDK 2.3.0 or later: The SDK uses the AppSign authentication mode by default. You only need to pass the [ZIMUserInfo`](@-ZIMUserInfo) when logging in.
-
If you use the Token-based authentication mode, please refer to the [Authentication](./Guides/Users/Authentication.mdx) to get the Token first, and pass it when logging in.
// userID must be within 32 characters, and can only contain letters, numbers, and the following special characters: '~', '!', '@', '#', '$', '%', '^', '&', '*', '(', ')', '_', '+', '=', '-', '`', ';', '’', ',', '.', '<', '>', '/', '\'.
// userName A string of up to 256 bytes, with no restrictions on special characters.
const userInfo = { userID: 'xxxx', userName: 'xxxx' };
const userID = 'xxxx';
const config: ZIMLoginConfig = {
userName: 'xxxx',
token: '',
customStatus: '',
isOfflineLogin: false,
}
// When logging in:
// If you use the Token-based authentication, pass the Token you get by referring to the [Guides - Authentication] document.
// If you use the AppSign authentication mode (the default auth mode by SDK 2.3.0), you need to leave the Token field empty.
zim.login(userID, config)
.then(function () {
// Login successful.
})
.catch(function (err) {
// Login failed.
});
Send messages
Client A can send messages to client B after logging in successfully.
The message types currently supported by ZIM are as follows:
Message Type | Description | Feature and Scenario |
---|---|---|
ZIMCommandMessage(2) | The signaling message whose content can be customized. A signaling message cannot exceed 5 KB in size, and up to 10 signaling messages can be sent per second per client. | Signaling messages, unable to be stored, are applicable to signaling transmission (for example, co-hosting, virtual gifting, and course materials sending) in scenarios with a higher concurrency, such as chat rooms and online classrooms. Higher concurrency is supported, but it's unreliable: it does not ensure message delivery and order. API: sendMessage |
ZIMBarrageMessage(20) | On-screen comments in a chat room. An on-screen comment cannot exceed 5 KB in size, and there is no number limit on comments that can be sent per second per client. | On-screen comments, unable to be stored are usually unreliable messages that are sent at a high frequency and can be discarded. A high concurrency is supported, but it's unreliable: it does not ensure message delivery. API: sendMessage |
ZIMTextMessage(1) | The text message. The default upper limit of message size is 2 KB. If necessary, please contact ZEGOCLOUD technical support for configuration, with a maximum size of up to 32 KB. Up to 10 text messages can be sent per second per client. | Text messages are reliable, in order, and able to be stored as historical messages. (For the storage duration, please refer to Pricing - Plan Fee - Plan Differences).
API: sendMessage、replyMessage |
ZIMMultipleMessage(10) | Multi-item message, a message that can include multiple texts, up to 10 images, 1 file, 1 audio, 1 video, and 1 custom message. Note
| |
ZIMImageMessage(11) | Image message. Applicable formats includes JPG, PNG, BMP, TIFF, GIF, and WebP. The maximum size is 10 MB. Up to 10 image messages can be sent per second per client. | |
ZIMFileMessage(12) | File Message. A file message contains a file of any format and cannot exceed 100 MB in size. Up to 10 file messages can be sent per second per client. | |
ZIMAudioMessage(13) | Audio message. An audio message contains an MP3 or M4A audio of up to 300 seconds and cannot exceed 6 MB in size. Up to 10 audio messages can be sent per second per client. | |
ZIMVideoMessage(14) | A video message contains an MP4 or MOV video and cannot exceed 100 MB in size. Up to 10 video messages can be sent per second per client. Note To retrieve the width and height of the first video frame after a video is successfully sent, the video must be encoded in H.264 or H.265. | |
ZIMCombineMessage(100) | For combined messages, there is no limit on message size, and the sending frequency of a single client is limited to 10 times/second. | |
ZIMCustomMessage(200) | You can customize the message type and parse the message without using the ZIM SDK. The default upper limit of message size is 2 KB. If necessary, please contact ZEGOCLOUD technical support for configuration, with a maximum size of up to 32 KB. |
To send one-to-one messages, for example, if client A wants to send a message to client B, then client A needs to call the sendMessage method with client B's userID, message content, and message type (conversationType).
-
ZIMMessageSentResult: Callback for the results that whether the message is sent successfully.
-
onMessageAttached callback: The callback on the message not sent yet. Before the message is sent, you can get a temporary ZIMMessage message for you to implement your business logic as needed. For example, you can get the ID of the message before sending it. Or when sending a message with large content, such as a video, you can get the localMessageID of the message before the message is uploaded to implement a Loading UI effect.
// Send one-to-one text messages.
const toConversationID = ''; // The other user's userID
const conversationType = 0; // Conversation type, 0: One-on-one chat, 1: Chat room, 2: Group chat.
const config: ZIMMessageSendConfig = {
priority: 1, // Set message priority, 1: Low (by default), 2: Medium, 3: High.
};
const messageTextObj: ZIMMessage = { type: 1, message: 'xxxx' };
const notification: ZIMMessageSendNotification = {
onMessageAttached: function(message) {
// todo: Loading
}
}
zim.sendMessage(messageTextObj, toConversationID, conversationType, config, notification)
.then(function ({ message }) {
// Message sent successfully.
})
.catch(function (err) {
// Failed to send the message.
});
Receive messages
After client B logs in, he will receive client A's message through the callback on which is already set in the peerMessageReceived method.
// Set up and listen for the callback for receiving the one-to-one messages.
zim.on('receivePeerMessage', function (zim, { messageList, fromConversationID }) {
console.log('receivePeerMessage', messageList, fromConversationID);
});
Log out
For a client to log out, call the logout method.
zim.logout();
Destroy the ZIM SDK instance
To destroy the ZIM SDK instance, call the destroy method.
zim.destroy();