Calculate call duration
This doc describes how to calculate the call duration through configuration.
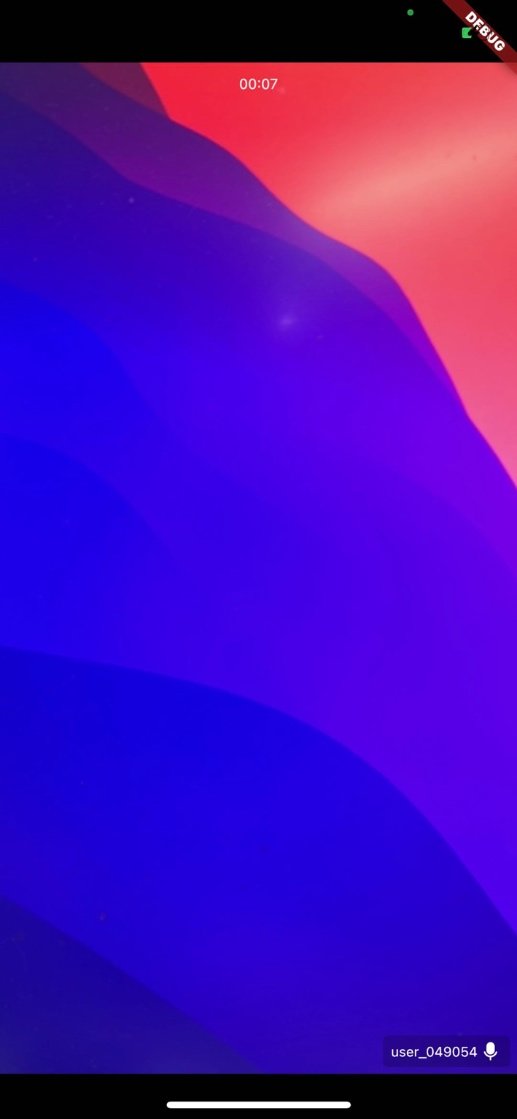
To calculate call duration, do the following:
-
Set the
isVisible
property ofdurationConfig
to display the current call timer. (It is displayed by default) -
Assuming that the call duration is 5 minutes, the call will automatically end when the time is up (refer to the following code). You will be notified of the end of the call duration through
durationConfig.onDurationUpdate
. To end the call, you can call thehangUp
method ofZegoUIKitPrebuiltCallService
orZegoUIKitPrebuiltCall
ref.
With call invitation
Basic call
import ZegoUIKitPrebuiltCallService from '@zegocloud/zego-uikit-prebuilt-call-rn';
import * as ZIM from 'zego-zim-react-native';
import * as ZPNs from 'zego-zpns-react-native';
ZegoUIKitPrebuiltCallService.init(
yourAppID,
yourAppSign,
userID,
userName,
[ZIM, ZPNs],
{
ringtoneConfig: {
incomingCallFileName: 'zego_incoming.mp3',
outgoingCallFileName: 'zego_outgoing.mp3',
},
requireConfig: (data) => {
return {
// \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
timingConfig: {
isDurationVisible: true,
onDurationUpdate: (duration) => {
if (duration === 5 * 60) {
ZegoUIKitPrebuiltCallService.hangUp();
}
},
},
// \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
};
},
notifyWhenAppRunningInBackgroundOrQuit: true,
isIOSSandboxEnvironment: true,
androidNotificationConfig: {
channelID: "ZegoUIKit",
channelName: "ZegoUIKit",
},
},
)
1
import React, { useRef } from 'react';
import { StyleSheet, View } from 'react-native';
import { ZegoUIKitPrebuiltCall, ONE_ON_ONE_VIDEO_CALL_CONFIG } from '@zegocloud/zego-uikit-prebuilt-call-rn';
export default function VideoCallPage(props) {
const prebuiltRef = useRef();
return (
<View style={styles.container}>
<ZegoUIKitPrebuiltCall
ref={prebuiltRef}
appID={yourAppID}
appSign={yourAppSign}
userID={userID} // userID can be something like a phone number or the user id on your own user system.
userName={userName}
callID={callID} // callID can be any unique string.
config={{
// You can also use ONE_ON_ONE_VOICE_CALL_CONFIG/GROUP_VIDEO_CALL_CONFIG/GROUP_VOICE_CALL_CONFIG to make more types of calls.
...ONE_ON_ONE_VIDEO_CALL_CONFIG,
onHangUp: () => { props.navigation.navigate('HomePage') },
// \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
durationConfig: {
isVisible: true,
onDurationUpdate: (duration) => {
if (duration === 5 * 60) {
prebuiltRef.current.hangUp();
}
}
},
// \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
}}
/>
</View>
);
}
1