Quick start (with call invitation)
You can refer to this document to understand the effects of the offline call invitation (system-calling UI) and complete the basic integration.
-
If your project needs Firebase integration or customization of features like ringtone and UI, complete the basic integration first and then refer to Customize the call and Enhance the call for further configuration.
-
Offline call invitation configuration is complex. If you only require online call invitations, please skip the steps related to firebase console and apple certificate.
UI Implementation Effects
Recorded on Xiaomi and iPhone, the outcome may differ on different devices.
Online call | online call (Android App background) | offline call (Android App killed) | offline call (iOS Background/Killed) |
---|---|---|---|
![]() | ![]() | ![]() | ![]() |
Integration Guide for Common Components
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Add dependencies.
- Run the following code in your project root directory, to install
@zegocloud/zego-uikit-prebuilt-call-rn
.
yarn add @zegocloud/zego-uikit-prebuilt-call-rn
npm install @zegocloud/zego-uikit-prebuilt-call-rn
-
If you are using Expo, please note that we only support Expo bare workflow. (We have only done basic testing on expo. If possible, it is recommended to integrate call kit with a standard react native project.)
-
call invitation feature is not compatible with "expo-notifications" and cannot be used together. If you are using "expo-notifications", it is recommended to remove this dependency or migrate to "@react-native-firebase/messaging".
- Add other dependencies.
Run the following command to install other dependencies for making sure the @zegocloud/zego-uikit-prebuilt-call-rn
can work properly.
yarn add @zegocloud/zego-uikit-rn react-delegate-component @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-sound react-native-encrypted-storage zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.16.0 zego-zpns-react-native@2.6.6 zego-callkit-react-native@1.0.6 react-native-keep-awake@4.0.0 react-native-device-info
npm install @zegocloud/zego-uikit-rn react-delegate-component @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-sound react-native-encrypted-storage zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.16.0 zego-zpns-react-native@2.6.6 zego-callkit-react-native@1.0.6 react-native-keep-awake@4.0.0 react-native-device-info
Config React Navigation
To let users transition between multiple screens, you will need to install a navigation library. In React Native, there are several different types of navigation libraries you can use to create a navigation structure. For now, we use the React navigation.
- Place the
ZegoCallInvitationDialog
component on the top level of theNavigationContainer
.
<ZegoCallInvitationDialog />
- Add two routes (
ZegoUIKitPrebuiltCallWaitingScreen
andZegoUIKitPrebuiltCallInCallScreen
) to your stack navigator.
<Stack.Screen
options={{ headerShown: false }}
// DO NOT change the name
name="ZegoUIKitPrebuiltCallWaitingScreen"
component={ZegoUIKitPrebuiltCallWaitingScreen}
/>
<Stack.Screen
options={{ headerShown: false }}
// DO NOT change the name
name="ZegoUIKitPrebuiltCallInCallScreen"
component={ZegoUIKitPrebuiltCallInCallScreen}
/>
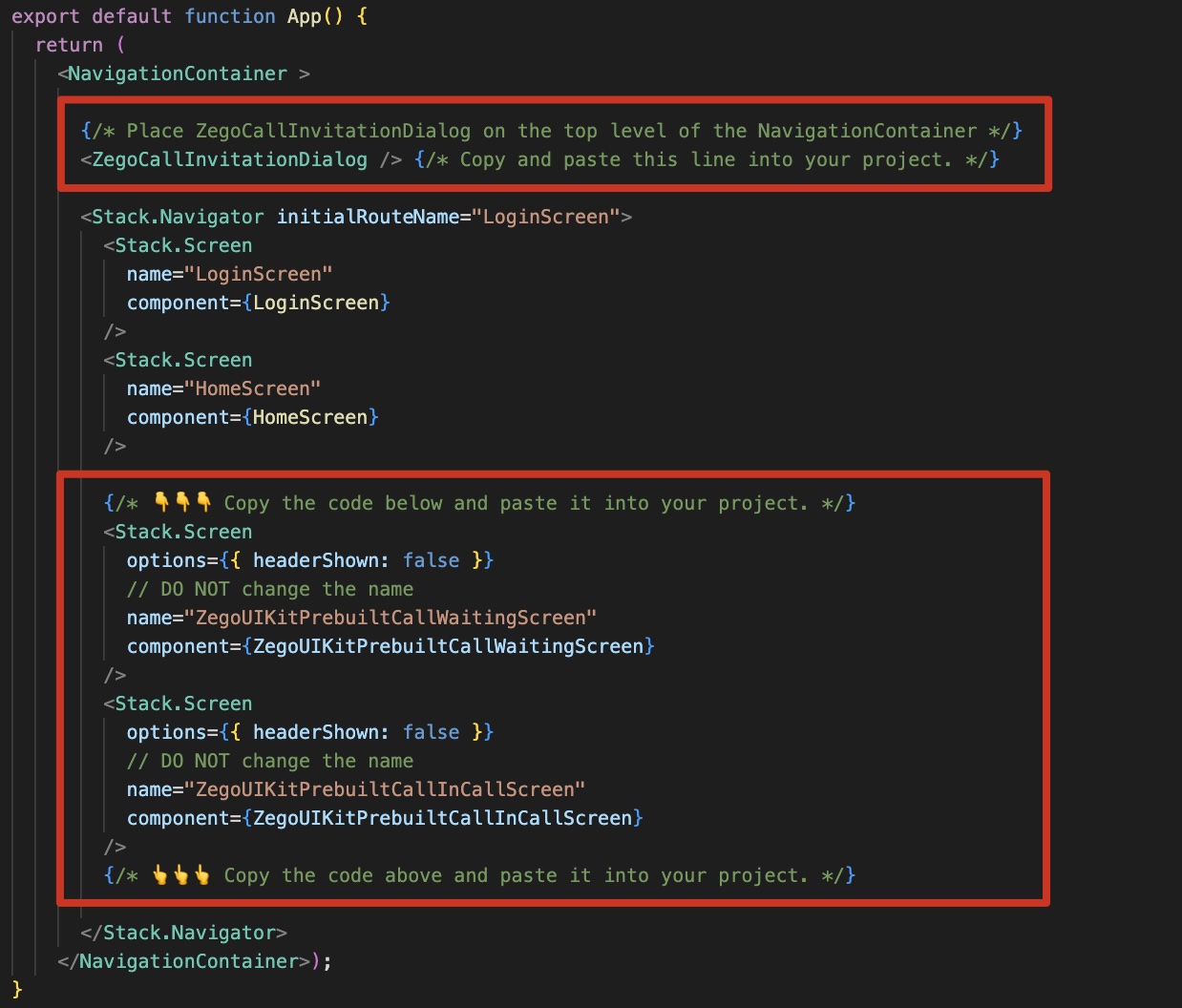
Initialize the call invitation service
- Call the
useSystemCallingUI
method in theindex.js
file.
import {AppRegistry} from 'react-native';
import App from './App';
import {name as appName} from './app.json';
// Add these lines \/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/
import ZegoUIKitPrebuiltCallService from '@zegocloud/zego-uikit-prebuilt-call-rn'
import * as ZIM from 'zego-zim-react-native';
import * as ZPNs from 'zego-zpns-react-native';
ZegoUIKitPrebuiltCallService.useSystemCallingUI([ZIM, ZPNs]);
// Add these lines /\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\/\
AppRegistry.registerComponent(appName, () => App);
- Call the
ZegoUIKitPrebuiltCallService.init
method.
We recommend calling this method immediately after the user logs into your app.
-
After the user logs in, it is necessary to Initialize the ZegoUIKitPrebuiltCallInvitationService to ensure that it is initialized only once, avoiding errors caused by repeated initialization.
-
When the user logs out, it is important to perform Deinitialize to clear the previous login records, preventing any impact on the next login.
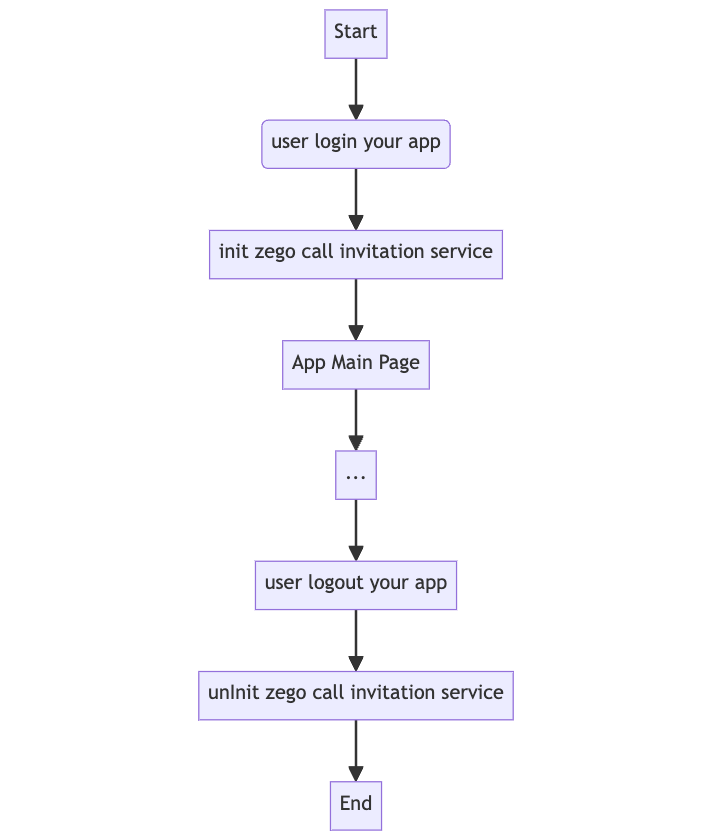
import ZegoUIKitPrebuiltCallService from '@zegocloud/zego-uikit-prebuilt-call-rn'
import * as ZIM from 'zego-zim-react-native';
import * as ZPNs from 'zego-zpns-react-native';
const onUserLogin = async (userID, userName, props) => {
return ZegoUIKitPrebuiltCallService.init(
yourAppID, // You can get it from ZEGOCLOUD's console
yourAppSign, // You can get it from ZEGOCLOUD's console
userID, // It can be any valid characters, but we recommend using a phone number.
userName,
[ZIM, ZPNs],
{
ringtoneConfig: {
incomingCallFileName: 'zego_incoming.mp3',
outgoingCallFileName: 'zego_outgoing.mp3',
},
androidNotificationConfig: {
channelID: "ZegoUIKit",
channelName: "ZegoUIKit",
},
});
}
const onUserLogout = async () => {
return ZegoUIKitPrebuiltCallService.uninit()
}
Add a call invitation button
Configure the "ZegoSendCallInvitationButton" to enable making calls.
<ZegoSendCallInvitationButton
invitees={[{userID: varUserID, userName: varUserName}]}
isVideoCall={true}
resourceID={"zego_call"} // Please fill in the resource ID name that has been configured in the ZEGOCLOUD's console here.
/>
<ZegoSendCallInvitationButton
invitees={invitees.map((inviteeID) => {
return { userID: inviteeID, userName: 'user_' + inviteeID };
})}
isVideoCall={true}
resourceID={"zego_call"} // Please fill in the resource ID name that has been configured in the ZEGOCLOUD's console here.
/>
For more parameters, go to Custom prebuilt UI.
Configure your project
Run & Test
If your device is not performing well or you found a UI stuttering, run in Release mode for a smoother experience.
- Run on an iOS device:
yarn ios
npm run ios
- Run on an Android device:
yarn android
npm run android
FAQ
Related guide
Refer this doc to make further customization.
Resources
Click here to get the complete sample code.