Quick start
This doc will guide you to integrate the In-app Chat Kit and start a chat quickly.
Prerequisites
- Go to ZEGOCLOUD Admin Console and do the following:
- Create a project, and get the
AppID
andAppSign
of your project. - Activate the In-app Chat service.
- Create a project, and get the
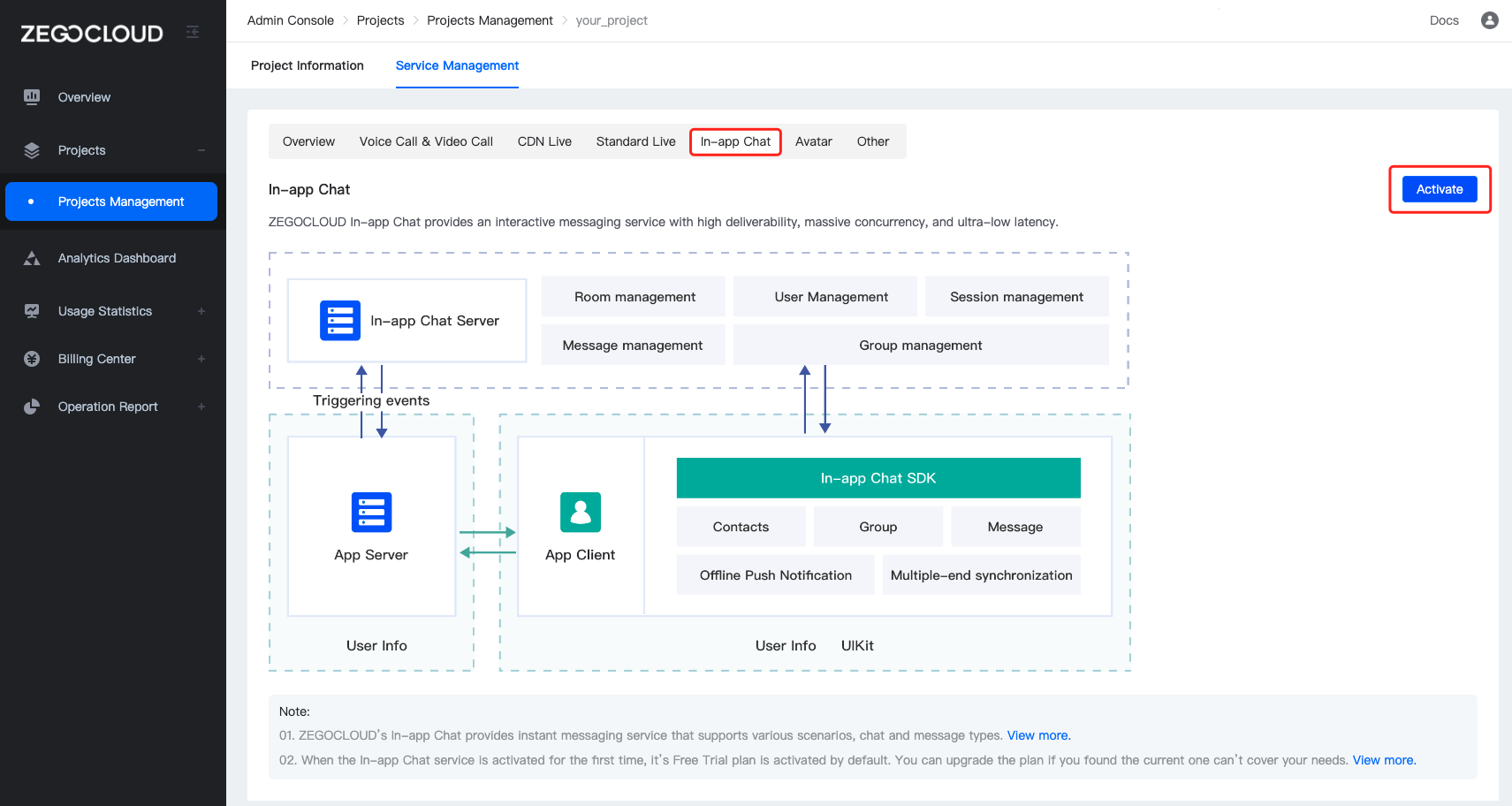
- Environment-specific requirements:
- Xcode 13.0 or later
- An iOS device that is running on iOS 12.0 or later
- The device is connected to the internet.
Integrate the SDK
The CocoaPods version needs to be 1.10.0 or later.
Open Podfile
and add pod 'ZIMKit'
target 'MyProject' do
use_frameworks!
pod 'ZIMKit'
end
Execute the command pod repo update
to update the local index and ensure that you can install the latest version of the SDK.
pod repo update
Execute pod install
to install the SDK.
pod install
Open the Info.plist
file and add the following:
<key>NSMicrophoneUsageDescription</key>
<string>We require microphone access to use zimkit.</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>We require photo access to use zimkit.</string>
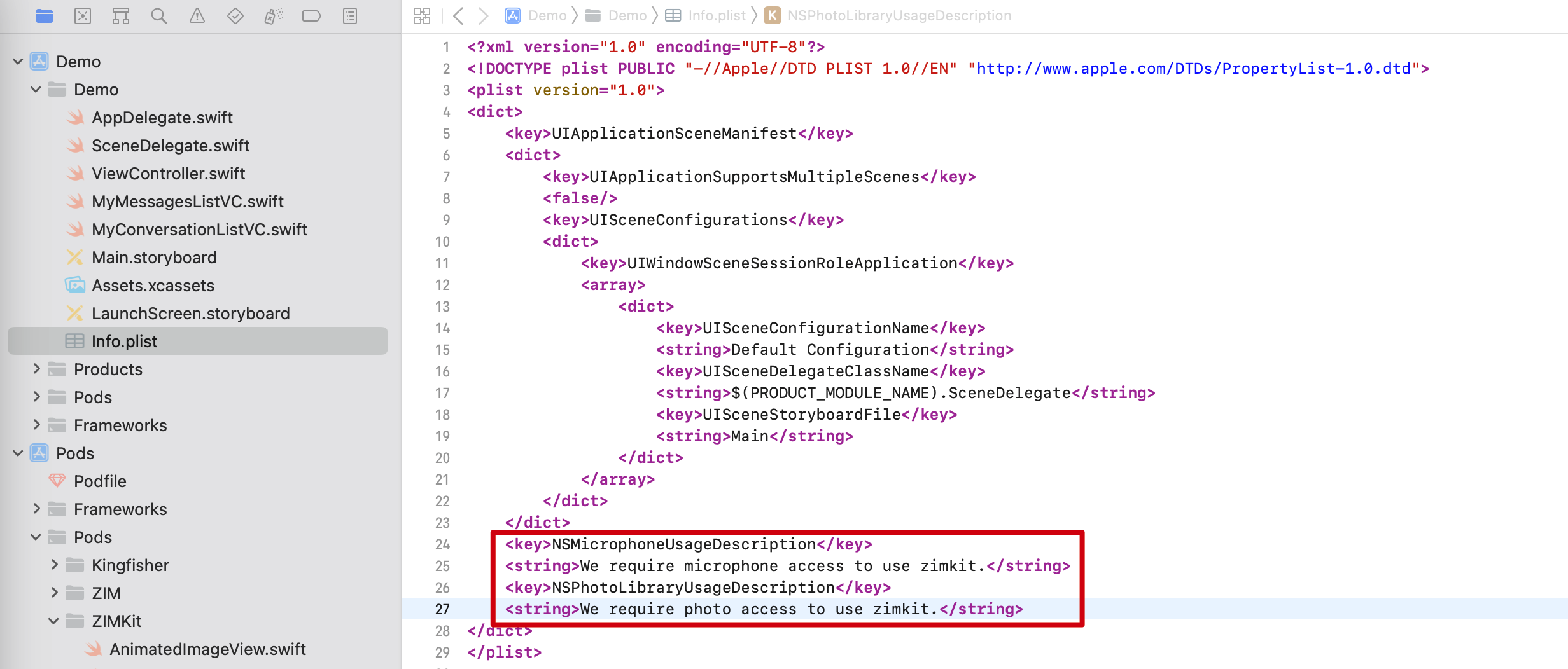
import UIKit
import ZIMKit
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
let appID: UInt32 = <#your appID#> // The AppID you get from ZEGOCLOUD Admin Console.
let appSign: String = <#your appSign#> // The AppSign you get from ZEGOCLOUD Admin Console.
ZIMKit.initWith(appID: appID, appSign: appSign)
}
}
You can customize rules to generate the user ID and user name. We recommend that you set a meaningful user ID. You can associate the user ID with your business account system.
import UIKit
import ZIMKit
/// your viewController.swift
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let connectUserButton = UIButton(type: .custom)
connectUserButton.setTitle("Connect User And Show", for: .normal)
connectUserButton.frame = .init(x: 100, y: 100, width: 250, height: 50)
connectUserButton.addTarget(self, action: #selector(connectUserAction), for: .touchUpInside)
connectUserButton.backgroundColor = .orange
view.addSubview(connectUserButton)
}
@objc func connectUserAction(_ sender: Any) {
// Your ID as a user.
let userID: String = "<#your userID#>"
// Your name as a user.
let userName: String = "<#your userName#>"
// The image you set as the user avatar must be network image. e.g., https://storage.zego.im/IMKit/avatar/avatar-0.png
let userAvatarUrl: String = "<#your userAvatarUrl#>"
ZIMKit.connectUser(userID: userID, userName: userName, avatarUrl: userAvatarUrl) { error in
// Display the UI views after connecting the user successfully.
if error.code == .success {
self?.showConversationListVC()
}
}
}
}
/// your viewController.swift
func showConversationListVC() {
let conversationVC = ZIMKitConversationListVC()
let nav = UINavigationController(rootViewController: conversationVC)
nav.modalPresentationStyle = .fullScreen
self.present(nav, animated: true)
}
Ideally, by this point, your app will look like this:
Start a chat
The In-app Chat Kit supports the following features:
Related guides
Click here to explore more UI components.
A quick guide to help you run the sample code.
Get support
Need help or want to raise your questions? Click the button below to join our Discord community to get quick responses.