Quick start (with cohosting)
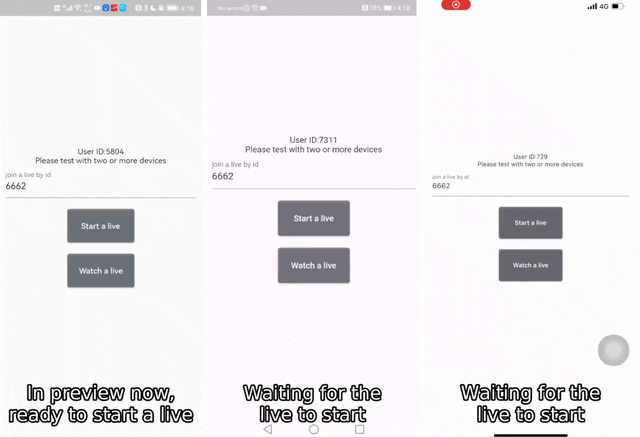
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Prepare the environment
Before you attempt to integrate the SDK, make sure that the development environment meets the following requirements:
- Xcode 15.0 or higher version.
- iOS 12.0 or higher version and iOS devices that support audio and video.
- The iOS device is connected to the Internet.
Integrate the SDK
Add dependencies
Do the following to add the ZegoUIKitPrebuiltLiveStream
and ZegoUIKitSignalingPlugin
dependencies:
-
Open Terminal, navigate to your project's root directory, and run the following to create a
podfile
:Untitledpod init
1 -
Edit the
Podfile
file to add the basic dependency:Untitledpod 'ZegoUIKitPrebuiltLiveStreaming' pod 'ZegoUIKitSignalingPlugin'
1 -
In Terminal, run the following to download all required dependencies and SDK with Cocoapods:
Untitledpod install
1
Import SDKs & plug-in
Import the ZegoUIKit
, ZegoUIKitPrebuiltLiveStreaming
, and ZegoUIKitSignalingPlugin
into your project:
import ZegoUIKit
import ZegoUIKitSignalingPlugin
import ZegoUIKitPrebuiltLiveStreaming
// YourViewController.swift
class ViewController: UIViewController {
//Other code...
}
Using the Live Streaming Kit
- Specify the
userID
anduserName
for connecting the Live Streaming Kit service. - Create a
liveID
that represents the live you want to start.
userID
andliveID
can only contain numbers, letters, and underlines (_).- Using the same
liveID
will enter the same live room.
With the same liveID
, only one user can enter the live stream as host. Other users need to enter the live stream as the audience.
class ViewController: UIViewController {
let appID: UInt32 = <#AppID#>
let appSign: String = <#AppSign#>
var userID: String = <#UserID#>
var userName: String = <#UserName#>
var liveID: String = <#LiveID#>
@IBAction func startLive(_ sender: Any) {
let config: ZegoUIKitPrebuiltLiveStreamingConfig = ZegoUIKitPrebuiltLiveStreamingConfig.host(enableSignalingPlugin: true)
config.enableCoHosting = true
let liveVC: ZegoUIKitPrebuiltLiveStreamingVC = ZegoUIKitPrebuiltLiveStreamingVC(appID, appSign: appSign, userID: userID, userName: userName, liveID: liveID, config: config)
liveVC.modalPresentationStyle = .fullScreen
self.present(liveVC, animated: true, completion: nil)
}
@IBAction func watchLive(_ sender: Any) {
let config: ZegoUIKitPrebuiltLiveStreamingConfig = ZegoUIKitPrebuiltLiveStreamingConfig.audience(enableSignalingPlugin: true)
config.enableCoHosting = true
let liveVC: ZegoUIKitPrebuiltLiveStreamingVC = ZegoUIKitPrebuiltLiveStreamingVC(appID, appSign: appSign, userID: userID, userName: userName, liveID: liveID, config: config)
liveVC.modalPresentationStyle = .fullScreen
self.present(liveVC, animated: true, completion: nil)
}
}
Then, you can start a live by presenting the VC
.
Configure your project
Open the Info.plist
, add the following code inside the dict
part:
<key>NSCameraUsageDescription</key>
<string>We require camera access to connect to a live</string>
<key>NSMicrophoneUsageDescription</key>
<string>We require microphone access to connect to a live</string>
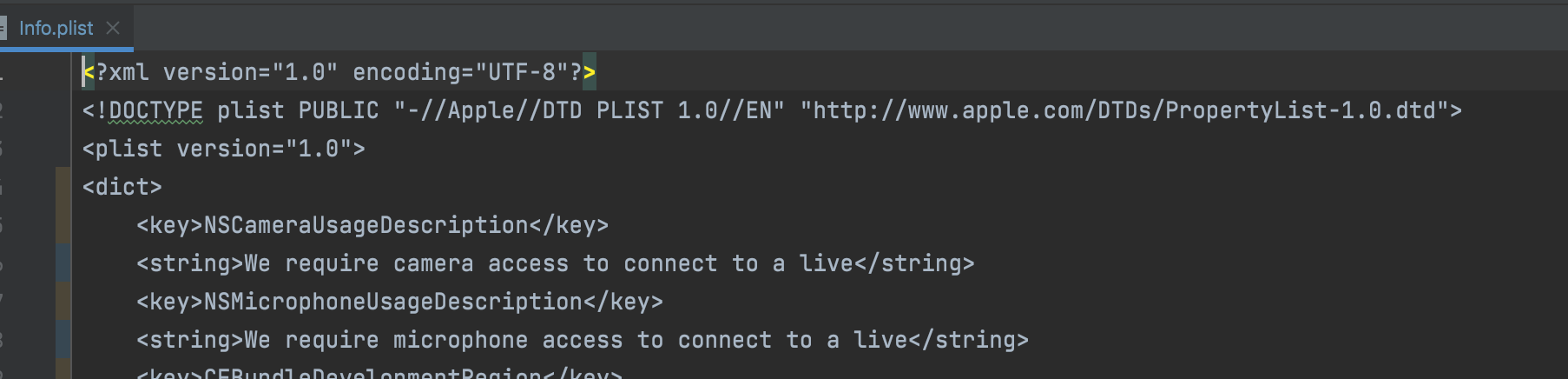
Run & Test
Now you have finished all the steps!
You can simply click the Run in XCode to run and test your App on your device.
Related guides
Resources
Click here to get the complete sample code.