Use in conjunction with Call Kit
This doc will introduce how to use In-app Chat Kit with Call Kit.
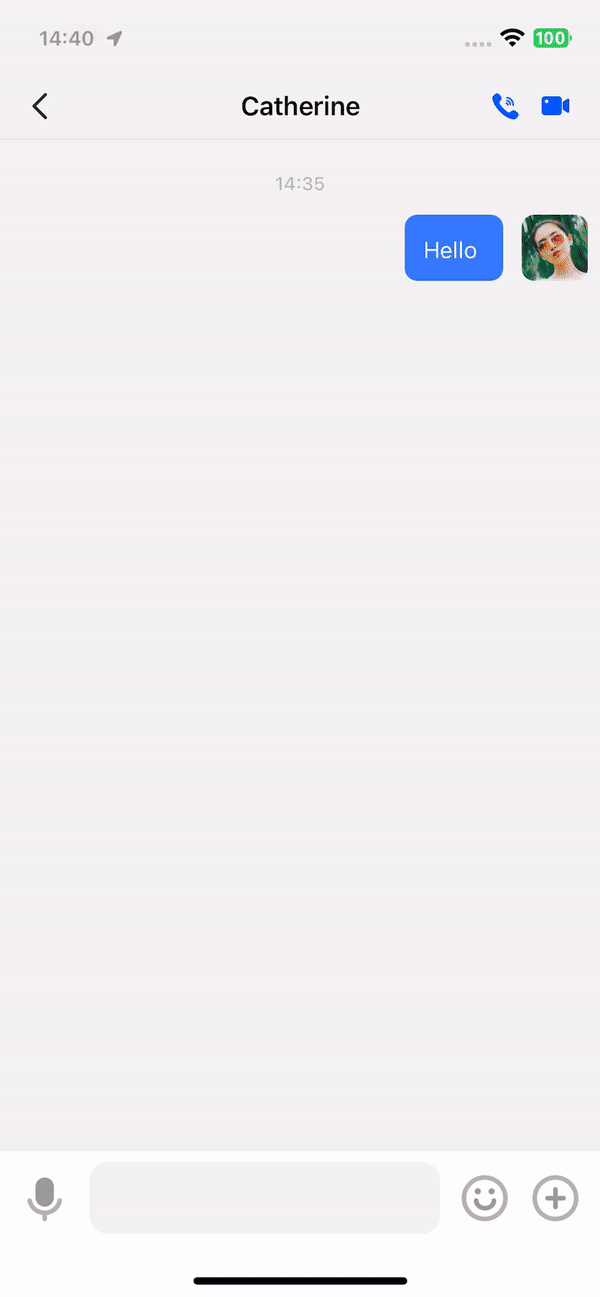
Prerequisites
Integrate the In-app Chat Kit SDK into your project. For more information, see Quick start.
Integrate the Call Kit
Add Call Kit dependency
- Run the following code in your project root directory:
flutter pub add zego_uikit_prebuilt_call
flutter pub add zego_uikit_signaling_plugin
- Run the following code in your project root directory to install all dependencies.
flutter pub get
Initialize the Call Kit
To receive the call invites from others and let the calling notification show on the top bar when receiving it, you will need to initialize the call invitation service (ZegoUIKitPrebuiltCallInvitationService) first.
1. Set up the context.
To make the UI show when receiving a call invite, you will need to get the Context. To do so, do the following 3 steps:
- 1.1 Initialize the
ZIMKit
. - 1.2 Define a
navigatorkey
, then set thenavigatorKey
toZegoUIKitPrebuiltCallInvitationService
. - 1.3 Register the
navigatorKey
toMaterialApp
.
void main() {
/// 1.1 init ZIMKit
ZIMKit().init(
appID: YourSecret.appID, // your appid
appSign: YourSecret.appSign, // your appSign
);
/// 1.2 define a navigator key, then set navigator key to ZegoUIKitPrebuiltCallInvitationService
final navigatorKey = GlobalKey<NavigatorState>();
ZegoUIKitPrebuiltCallInvitationService().setNavigatorKey(navigatorKey);
runApp(ZIMKitDemo(navigatorKey));
}
class ZIMKitDemo extends StatelessWidget {
const ZIMKitDemo(this.navigatorKey, {Key? key}) : super(key: key);
final GlobalKey<NavigatorState>? navigatorKey;
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
/// 1.3: register the navigator key to MaterialApp
navigatorKey: navigatorKey,
debugShowCheckedModeBanner: false,
title: 'ZIMKit Demo',
theme: ThemeData(primarySwatch: Colors.blue),
home: const ZIMKitDemoLoginPage(),
);
}
}
2. Initialize/Deinitialize the call invitation service.
- 2.1 Initialize the service when your app users logged in successfully or re-logged in after an exit.
await ZIMKit().connectUser(id: userID.text, name: userName.text).then((errorCode) {
if (mounted) {
if (errorCode == 0) {
/// 2.1. initialized ZegoUIKitPrebuiltCallInvitationService
/// when app's user is logged in or re-logged in
/// We recommend calling this method as soon as the user logs in to your app.
ZegoUIKitPrebuiltCallInvitationService().init(
appID: yourAppID /*input your AppID*/,
appSign: yourAppSign /*input your AppSign*/,
userID: currentUser.id,
userName: currentUser.name,
plugins: [ZegoUIKitSignalingPlugin()],
);
Navigator.of(context).pushReplacement(
MaterialPageRoute(
builder: (context) => const ZIMKitDemoHomePage(),
),
);
} else {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text('login failed, errorCode: $errorCode'),
),
);
}
}
});
- 2.2 Deinitialize the service after your app users logged out.
/// on App's user logout
void onUserLogout() {
/// 2.2. de-initialization ZegoUIKitPrebuiltCallInvitationService
/// when app's user is logged out
ZegoUIKitPrebuiltCallInvitationService().uninit();
}
Add a call button
Add a call button to the appBar
using the appBarActions
parameter of ZIMKitMessageListPage
:
ZIMKitMessageListPage(
conversationID: conversation.id,
conversationType: conversation.type,
appBarActions: conversation.type == ZIMConversationType.peer
? [
for (final isVideoCall in [true, false])
ZegoSendCallInvitationButton(
iconSize: const Size(40, 40),
buttonSize: const Size(50, 50),
isVideoCall: isVideoCall,
invitees: [ZegoUIKitUser(id: conversation.id, name: conversation.name)],
onPressed: (String code, String message, List<String> errorInvitees) {
onCallInvitationSent(context, code, message, errorInvitees);
},
),
]
: [],
);
void onCallInvitationSent(BuildContext context, String code, String message, List<String> errorInvitees) {
late String log;
if (errorInvitees.isNotEmpty) {
log = "User doesn't exist or is offline: ${errorInvitees[0]}";
if (code.isNotEmpty) {
log += ', code: $code, message:$message';
}
} else if (code.isNotEmpty) {
log = 'code: $code, message:$message';
}
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text(log)),
);
}
Configure your project
After integrating Call Kit, configure the following for your app project
So far, you have successfully integrated Call Kit in the In-app Chat Kit. You can now run and experience it.
More resources
The above content only introduces the basic configuration required for integrating Call Kit. If you need to further customize the configuration of the call, refer to the following documents:
Steps in this doc helps you to make a call quickly.
This article guides you on how to further customize the features and UI of the call.