Customize the seat-taking logic
Customize the audience's seat-taking logic
The reference code below implements the following:
- Decide whether to show the "apply to take a seat" button.
- Listen to the
ZegoLiveAudioRoomSeatEvents.onClosed
andZegoLiveAudioRoomSeatEvents.onOpened
, you can tell whether the seat is open or closed. If open, the button shows. If closed, the button shall be hidden.
- How to know whether a seat-taking request is sent?
- Listen to the
ZegoLiveAudioRoomSeatAudienceEvents.onTakingRequestFailed
andZegoLiveAudioRoomSeatAudienceEvents.onTakingRequestRejected
callbacks, and you can tell when the request is sent successfully via these callbacks. - Listen to the
takenSeats
in the callbackZegoLiveAudioRoomSeatEvents.onChanged
, you can tell when the audience takes a seat successfully.
- How to apply to take a seat and cancel the seat-taking request?
- To send a seat-taking request, use the
applyToTake
method inZegoUIKitPrebuiltLiveAudioRoomController().seat.audience
. - To cancel the seat-taking request after applying, use the
cancelTakingRequest
method inZegoUIKitPrebuiltLiveAudioRoomController().seat.audience
. - And sure, these methods will return a result, you can do further logic customization based on the returned results.
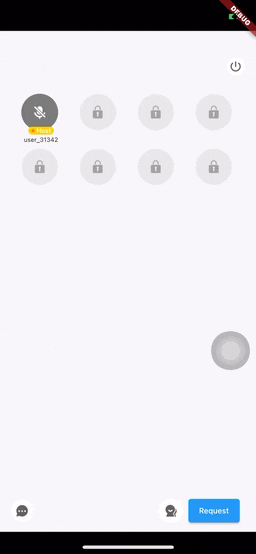
class LivePage extends StatefulWidget {
const LivePage({
Key? key,
required this.roomID,
this.isHost = false,
}) : super(key: key);
final String roomID;
final bool isHost;
@override
State<LivePage> createState() => _LivePageState();
}
class _LivePageState extends State<LivePage> {
final isSeatClosedNotifier = ValueNotifier<bool>(false);
final isRequestingNotifier = ValueNotifier<bool>(false);
@override
Widget build(BuildContext context) {
return SafeArea(
child: ZegoUIKitPrebuiltLiveAudioRoom(
appID: YourSecret.appID,
appSign: YourSecret.appSign,
userID: userID,
userName: 'user_$userID',
roomID: widget.roomID,
events: ZegoUIKitPrebuiltLiveAudioRoomEvents(
seat: ZegoLiveAudioRoomSeatEvents(
onClosed: () {
isSeatClosedNotifier.value = true;
},
onOpened: () {
isSeatClosedNotifier.value = false;
},
onChanged: (
Map<int, ZegoUIKitUser> takenSeats,
List<int> untakenSeats,
) {
if (isRequestingNotifier.value) {
if (takenSeats.values
.map((e) => e.id)
.toList()
.contains(userID)) {
/// on the seat now
isRequestingNotifier.value = false;
}
}
},
host: ZegoLiveAudioRoomSeatHostEvents(),
audience: ZegoLiveAudioRoomSeatAudienceEvents(
onTakingRequestFailed: () {
isRequestingNotifier.value = false;
},
onTakingRequestRejected: () {
isRequestingNotifier.value = false;
},
),
),
),
config: (widget.isHost
? ZegoUIKitPrebuiltLiveAudioRoomConfig.host()
: ZegoUIKitPrebuiltLiveAudioRoomConfig.audience())
/// Remove the button that is used for the audience to apply to take a seat.
..bottomMenuBar.audienceButtons = const [
ZegoLiveAudioRoomMenuBarButtonName.showMemberListButton,
]
/// Add custom button.
..bottomMenuBar.audienceExtendButtons = [
connectButton(),
]),
);
}
Widget connectButton() {
return ValueListenableBuilder<bool>(
valueListenable: isSeatClosedNotifier,
builder: (context, isSeatClosed, _) {
return isSeatClosed
? ValueListenableBuilder<bool>(
valueListenable: isRequestingNotifier,
builder: (context, isRequesting, _) {
return isRequesting
? ElevatedButton(
onPressed: () {
ZegoUIKitPrebuiltLiveAudioRoomController()
.seat
.audience
.cancelTakingRequest()
.then((result) {
isRequestingNotifier.value = false;
});
},
child: const Text('Cancel'),
)
: ElevatedButton(
onPressed: () {
ZegoUIKitPrebuiltLiveAudioRoomController()
.seat
.audience
.applyToTake()
.then((result) {
isRequestingNotifier.value = result;
});
},
child: const Text('Request'),
);
},
)
: Container();
},
);
}
}
Customize your own business logic
You can use API, config, and callback to achieve more detailed control to fit your business logic.
API
speaker
The following functions will only take effect when the user role performing the operation is a speaker.
leave
Leave the seat voluntarily.
A pop-up confirmation prompt will show when the showDialog
is true
.
Future<bool> leave({bool showDialog = true}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.speaker.leave(showDialog: true)
host
The following functions will only take effect when the user role performing the operation is a host.
removeSpeaker
Remove the user with the ID userID
from the seat.
Future<void> removeSpeaker(String userID, {bool showDialogConfirm = true,}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.removeSpeaker(userID)
close
Close(lock) the seat(s).
You can use the close()
method to close all seats or specify only to close a certain seat by using the targetIndex
.
Once all seats are closed, audiences can only be invited by the host or request a seat by sending a seat-taking request themselves.
Future<bool> close({
int targetIndex = -1,
}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.close(targetIndex: 2)
open
When the current user is the host, Open(unlock) the seat(s).
You can use open()
to open all seats or open the seat specified by targetIndex
.
Once the seat is opened, the audience can then take the seat by clicking on the seat.
Future<bool> open({
int targetIndex = -1,
}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.close(targetIndex: 2)
acceptTakingRequest
When the current user is the host, accept seat-taking requests for the audience with the ID audienceUserID
.
Future<bool> acceptTakingRequest(String audienceUserID) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.acceptTakingRequest(audienceUserID)
rejectTakingRequest
When the current user is the host, reject seat-taking requests for the audience with the ID audienceUserID
.
Future<bool> rejectTakingRequest(String audienceUserID) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.rejectTakingRequest(audienceUserID)
inviteToTake
When the current user is the host, invite the audience with the ID audienceUserID
to take the speaker seat.
Future<bool> inviteToTake(String audienceUserID) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.inviteToTake(audienceUserID)
mute
When the current user is the host, set whether the speaker at the seat position targetIndex
is muted based on the muted
value.
After the host initiates the mute operation, if the value of muted
is false, the speaker at the position of targetIndex
will receive the ZegoLiveAudioRoomAudioVideoEvents.onMicrophoneTurnOnByOthersConfirmation
callback. The speaker needs to return true
in this callback to agree to the host opening their microphone.
Future<bool> mute({
int targetIndex = -1,
bool muted = true,
}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.mute(targetIndex: 1, muted: true)
muteByUserID
When the current user is the host, set whether the speaker with id targetUserID
is muted based on the muted
value.
After the host initiates the mute operation, if the value of muted
is false, the speaker with id targetUserID
will receive the ZegoLiveAudioRoomAudioVideoEvents.onMicrophoneTurnOnByOthersConfirmation
callback. The speaker needs to return true
in this callback to agree to the host opening their microphone.
Future<bool> muteByUserID(
String userID, {
bool muted = true,
}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.host.mute(targetUserID, muted: true)
audience
The following functions will only take effect when the user role performing the operation is a speaker.
applyToTake
Applies to take a speaker seat.
Future<bool> applyToTake() async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.audience.applyToTake()
cancelTakingRequest
Cancels his seat-taking request.
Future<bool> cancelTakingRequest() async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.audience.cancelTakingRequest()
acceptTakingInvitation
Accepts the seat-taking invite from the host.
Future<bool> acceptTakingInvitation({
required BuildContext context,
bool rootNavigator = false,
}) async
ZegoUIKitPrebuiltLiveAudioRoomController().seat.audience.acceptTakingInvitation()
config
-
ZegoLiveAudioRoomLayoutConfig
layout
:The default layout of the audio chat room supports free layout with multiple rows and columns of seats.
You can use this parameter to control the specific style of each row and column.
-
int
takeIndexWhenJoining
:Specifies the seat to occupy when joining the live audio room.
This is only valid when the role is set to host or speaker.
-
int Function(ZegoUIKitUser user)?
takeIndexWhenAudienceRequesting
:When the audience take on seat, do you want specify a seat? If so, return to the seat you want to specify
-
bool
closeWhenJoining
:Specifies whether to lock the seat automatically after entering the room.
It only takes effect when set by the host, the default value is
true
. -
List<int>
hostIndexes
:Specifies the list of seats occupied by the host.
Once specified, these seats can only be used by the host and cannot be occupied by speakers or audience members.
The default value is
[0]
.
event callbacks
The ZegoLiveAudioRoomSeatEvents.onClicked
and ZegoLiveAudioRoomMemberListEvents.onMoreButtonPressed
overrides Live Audio Room Kit's prebuilt logic, meaning that when you customize these events, the prebuilt events are no longer executed.
- ZegoLiveAudioRoomMemberListEvents
- Function(ZegoUIKitUser user)?
onMoreButtonPressed
: This callback will be triggered when the more button in the member list is pressed.
- Function(ZegoUIKitUser user)?
- ZegoLiveAudioRoomSeatEvents
- Function(int index, ZegoUIKitUser? user)
onClicked
: This callback will be triggered when a seat is clicked. - Function()
onClosed
: This callback will be triggered when a speaker seat is closed. - Function()
onOpened
: This callback will be triggered when a closed speaker seat is opened. - Function(Map<int/seat index/, ZegoUIKitUser> takenSeats, List<int> untakenSeats)
onChanged
: This callback will be triggered when someone gets on/gets off/switches seat. - ZegoLiveAudioRoomSeatHostEvents
-
Function(ZegoUIKitUser audience)
onTakingRequested
: The host will receive a notification via this callback when the audience applies to take a seat. -
Function(ZegoUIKitUser audience)
onTakingRequestCanceled
: The host will receive a notification via this callback when the audience cancels his seat-taking request. -
VoidCallback?
onTakingInvitationFailed
: The host will receive a notification via this callback when a seat-taking invite failed. -
void Function(ZegoUIKitUser audience)?
onTakingInvitationRejected
: The host will receive a notification via this callback when the audience rejects the seat-taking invite.
-
- ZegoLiveAudioRoomSeatAudienceEvents
- VoidCallback?
onTakingRequestFailed
: The audience will receive a notification via this callback when his seat-taking request fails. - VoidCallback?
onTakingRequestRejected
: The audience will receive a notification via this callback when his seat-taking request is rejected by the host. - VoidCallback?
onTakingInvitationReceived
: The audience will receive a notification via this callback when the host invites them to take a seat.
- VoidCallback?
- Function(int index, ZegoUIKitUser? user)