Quick start
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Integrate the SDK
Add ZegoUIKitPrebuiltLiveAudioRoom as dependencies
Run the following code in your project's root directory:
Untitled
flutter pub add zego_uikit_prebuilt_live_audio_room
1
Import the SDK
Now in your Dart code, import the Live Audio Room Kit SDK.
Untitled
import 'package:zego_uikit_prebuilt_live_audio_room/zego_uikit_prebuilt_live_audio_room.dart';
1
Using the Live Audio Room Kit
- Specify the
userID
anduserName
for connecting the Live Audio Room Kit service. - Create a
roomID
that represents the live audio room you want to create.
Note
userID
,userName
, androomID
can only contain numbers, letters, and underlines (_).- Using the same
roomID
will enter the same live audio room.
Warning
With the same roomID
, only one user can enter the live audio room as host. Other users need to enter the live audio room as the audience.
Untitled
class LivePage extends StatelessWidget {
final String roomID;
final bool isHost;
const LivePage({Key? key, required this.roomID, this.isHost = false}) : super(key: key);
@override
Widget build(BuildContext context) {
return SafeArea(
child: ZegoUIKitPrebuiltLiveAudioRoom(
appID: yourAppID, // Fill in the appID that you get from ZEGOCLOUD Admin Console.
appSign: yourAppSign, // Fill in the appSign that you get from ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
roomID: roomID,
config: isHost
? ZegoUIKitPrebuiltLiveAudioRoomConfig.host()
: ZegoUIKitPrebuiltLiveAudioRoomConfig.audience(),
),
);
}
}
1
Then, you can create a live audio room. And the audience can join the live audio room by entering the roomID
.
Config your project
Android
iOS
Run & Test
Now you can simply click the Run or Debug button to run and test your App on the device.
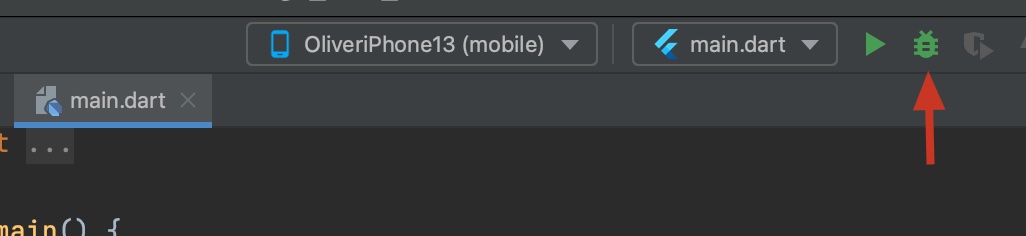
Related guide
Resources
Sample code
Click here to get the complete sample code.
API reference
Click here for detailed explanations of all APIs.