Edit messages
To use this feature, please subscribe to the enterprise plan.
Function introduction
The ZIM SDK supports users editing messages they have already sent in one-on-one or group chats. The updated content will be synchronized in real-time to all members of the conversation, ensuring that communication information remains consistent and up-to-date.
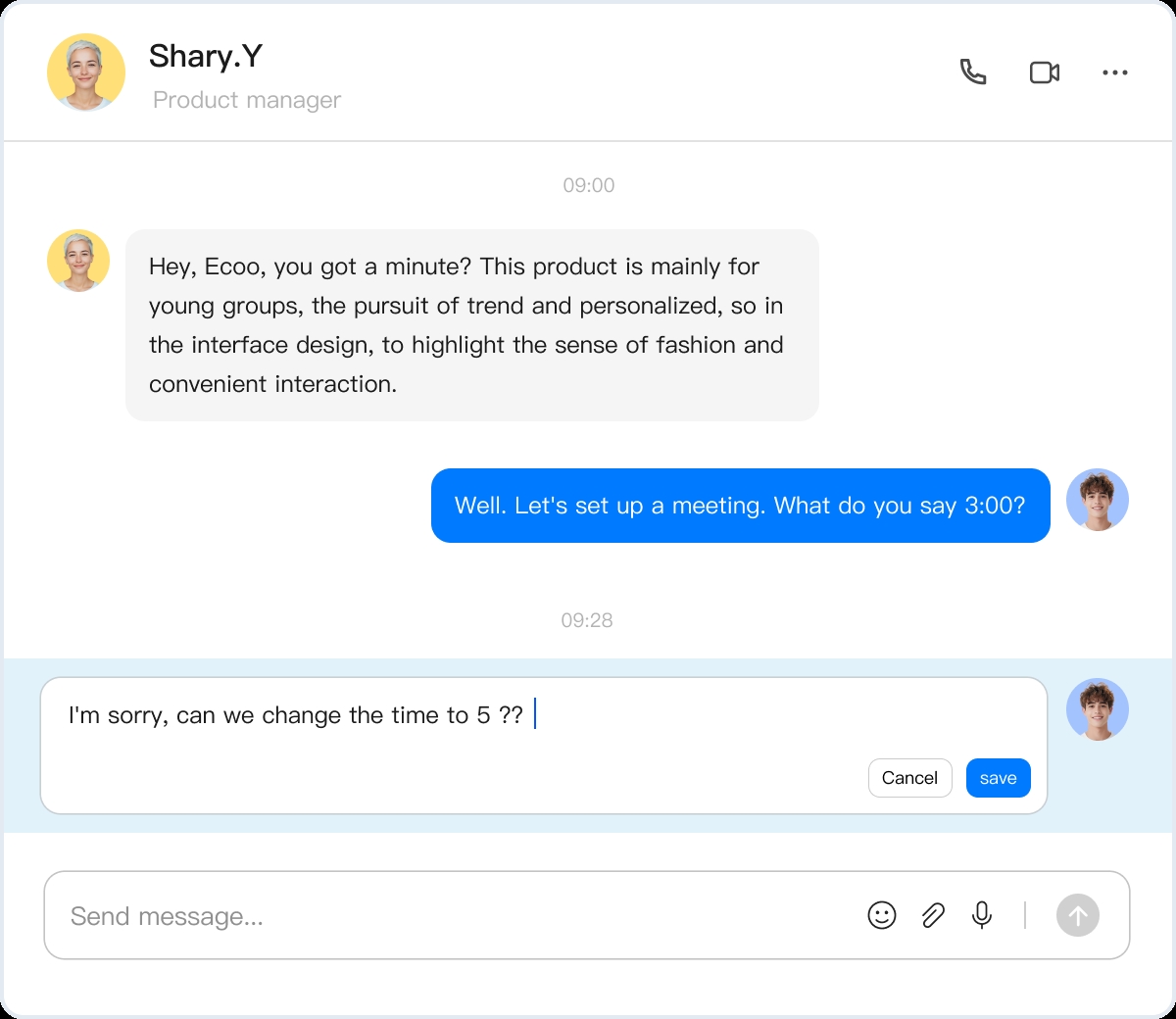
Setting up listener
Participants in a conversation register setEventHandler listeners for onMessageEdited callback related to message edits. When another user edits a message, you can directly obtain relevant information about the edited message, including the edit time and editor.
// Register event
zim.setEventHandler(new ZIMEventHandler() {
@Override
public void onMessageEdited(ZIM zim, ArrayList<ZIMMessage> messageList) {
// Upon receiving the list of edited messages, update the UI according to business needs
}
});
Edit a message
After successfully logging into the ZIM SDK, conversation participants can call the editMessage method to edit messages they have already sent (only supports the following types of messages: ZIMTextMessage, ZIMCustomMessage, ZIMMultipleMessage). The editable attributes are as follows:
- extendedData: Message extension field.
- isMentionAll: Whether to notify all participants (@everyone).
- mentionedUserIDs: List of notified users (@specific user).
- message: Content of ZIMTextMessage or ZIMCustomMessage.
- subType: Subtype of ZIMCustomMessage.
- messageInfoList: Item list of ZIMMultipleMessage.
- searchedContent: Search field of ZIMCustomMessage.
The result of the editing operation will then be known through ZIMMessageSentFullCallback.
- Only supports editing messages within 24 hours. The editing is based on the message's
timestamp
, which will not be updated due to the message editing. - Message type cannot be changed, for Sample: ZIMTextMessage cannot be converted to ZIMCustomMessage or ZIMMultipleMessage.
- Restrictions on each attribute in the edit message method are consistent with the relevant restrictions in the send message method.
- Editing a message triggers the server-side pre-message sending callback and post-message sending callback.
- If you have enabled ZIM content moderation, the edited message content will also be moderated, and the moderation process and limitations are the same as when sending a message.
- When the message content is text (moderation before sending), failing the review will result in editing failure, and the message content will not be updated, remaining as the original content.
- When the message is an image, voice, or video (sending before moderation), failing the review will result in the message being recalled, and it will not revert to the content before editing.
// Edit text message content
ZIMTextMessage messageObj; // Obtain from the queryHistoryMessage method
messageObj.message = "Edited message content";
ZIMMessageEditConfig config = new ZIMMessageEditConfig();
zim.editMessage(messageObj, config, new ZIMMessageSentFullCallback() {
@Override
public void onMessageAttached(ZIMMessage zimMessage) {}
// This callback is triggered when editing a ZIMMultipleMessage with local file uploads
@Override
public void onMultipleMediaUploadingProgress(
ZIMMultipleMessage message,
long currentFileSize,
long totalFileSize,
int messageInfoIndex,
long currentIndexFileSize,
long totalIndexFileSize,
) {}
@Override
public void onMessageSent(ZIMMessage zimMessage, ZIMError errorInfo) {
// Determine if the operation was successful based on errorInfo
}
});