Quick start
Prepare the environment
Before you attempt to integrate the SDK, make sure that the development environment meets the following requirements:
- Xcode 15.0 or higher version.
- iOS 12.0 or higher version and iOS devices that support audio and video.
- The iOS device is connected to the Internet.
Integrate the SDK
Add ZegoUIKitPrebuiltCall as dependencies
- Open Terminal, navigate to your project's root directory, and run the following to create a
podfile
:Untitledpod init
1 - Edit the
Podfile
file to add the basic dependency:Untitledpod 'ZegoUIKitPrebuiltCall'
1 - In Terminal, run the following to download all required dependencies and SDK with Cocoapods:
Untitled
pod install
1
Import ZegoUIKit & ZegoUIKitPrebuiltCall to your project
Untitled
import ZegoUIKit
import ZegoUIKitPrebuiltCall
// YourViewController.swift
class ViewController: UIViewController {
//Other code...
}
1
Using the ZegoUIKitPrebuiltCallVC in your project
- Go to ZEGOCLOUD Admin Console, get the
appID
andappSign
of your project. - Specify the
userID
anduserName
for connecting the Call Kit service. - Create a
callID
that represents the call you want to make.
Note
userID
andcallID
can only contain numbers, letters, and underlines (_).- Users that join the call with the same
callID
can talk to each other.
Untitled
// YourViewController.swift
class ViewController: UIViewController {
// Other code...
var userID: String = <#UserID#>
var userName: String = <#UserName#>
var callID: String = <#CallID#>
@IBAction func makeNewCall(_ sender: Any) {
// You can also use groupVideo/groupVoice/oneOnOneVoice to make more types of calls.
let config: ZegoUIKitPrebuiltCallConfig = ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall()
let callVC = ZegoUIKitPrebuiltCallVC.init(yourAppID,
appSign: yourAppSign,
userID: self.userID,
userName: self.userName,
callID: self.callID,
config: config)
callVC.modalPresentationStyle = .fullScreen
self.present(callVC, animated: true, completion: nil)
}
}
1
Then, you can make a new call by presenting the VC
.
Configure your project
Open the Info.plist
, add the following code inside the dict
part:
Info.plist
<key>NSCameraUsageDescription</key>
<string>We require camera access to connect to a call</string>
<key>NSMicrophoneUsageDescription</key>
<string>We require microphone access to connect to a call</string>
1
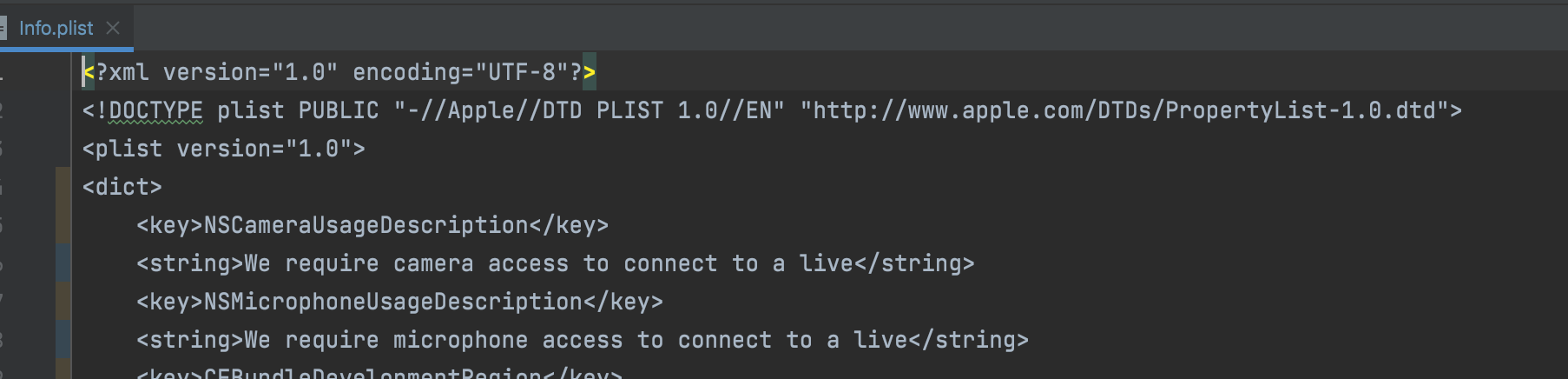
Run & Test
Now you have finished all the steps!
You can simply click the Run in XCode to run and test your App on your device.
Related guide
Resources
Sample code
Click here to get the complete sample code.