Quick start (with call invitation)
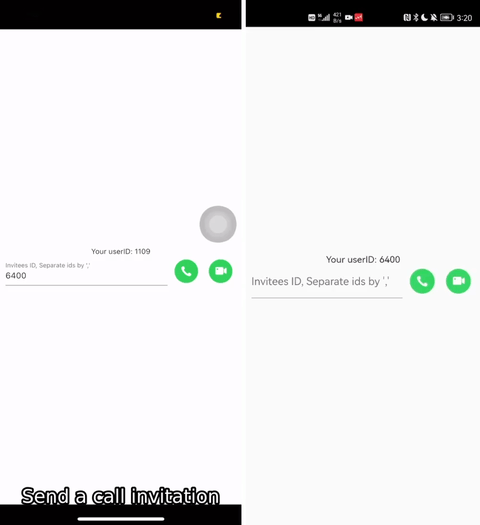
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Prepare the environment
Before you attempt to integrate the SDK, make sure that the development environment meets the following requirements:
- Xcode 15.0 or higher version.
- iOS 12.0 or higher version and iOS devices that support audio and video.
- The iOS device is connected to the Internet.
Add ZegoUIKitPrebuiltCall as dependencies
- Open Terminal, navigate to your project's root directory, and run the following to create a
podfile
:Untitledpod init
1 - Edit the
Podfile
file to add the basic dependencies:Untitledpod 'ZegoUIKitPrebuiltCall' pod 'ZegoUIKitSignalingPlugin' pod 'ZegoUIKitAppleCallKitPlugin'
1 - In Terminal, run the following to download all required dependencies and SDK with Cocoapods:
Untitled
pod install
1
Integrate the SDK with the call invitation feature
Initialize the call invitation service
Call the init
method on the App startup, and specify the userID
and userName
for connecting the Call Kit service.
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Get your AppID and AppSign from ZEGOCLOUD's Console
// userID can only contain numbers, letters, and '_'.
let config = ZegoUIKitPrebuiltCallInvitationConfig(notifyWhenAppRunningInBackgroundOrQuit: true, isSandboxEnvironment: false)
ZegoUIKitPrebuiltCallInvitationService.shared.initWithAppID(YOUR_APPID, appSign: YOUR_APP_SIGN, userID:YOUR_USER_ID, userName:YOUR_USER_NAME, config: config)
}
}
#import "ViewController.h"
@import ZegoUIKit;
@import ZegoUIKitPrebuiltCall;
@import ZegoUIKitSignalingPlugin;
@import ZegoPluginAdapter;
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Get your AppID and AppSign from ZEGOCLOUD's Console
// userID can only contain numbers, letters, and '_'.
// certificateIndex: indicates the signaling formation certificate verification method
ZegoUIKitPrebuiltCallInvitationConfig *config = [[ZegoUIKitPrebuiltCallInvitationConfig alloc] initWithNotifyWhenAppRunningInBackgroundOrQuit:YES isSandboxEnvironment:YES certificateIndex:ZegoSignalingPluginMultiCertificateFirstCertificate];
[[ZegoUIKitPrebuiltCallInvitationService shared] initWithAppID:YOUR_APPID appSign:YOUR_APP_SIGN userID:YOUR_USER_ID userName:YOUR_USER_NAME config:config];
}
Parameters of ZegoUIKitPrebuiltCallInvitationConfig class
Parameter | Type | Required | Description |
---|---|---|---|
incomingCallRingtone | String | No | Set the ringtone for incoming call invitations. |
outgoingCallRingtone | String | No | Set the ringtone for outgoing call invitations. |
showDeclineButton | Bool | No | Set the display or hide for the decline button. |
notifyWhenAppRunningInBackgroundOrQuit | Bool | No | Change notifyWhenAppRunningInBackgroundOrQuit to false if you don't need to receive a call invitation notification when your app is running in the background or the user has quitted the app. |
isSandboxEnvironment | Bool | No | To publish your app to TestFlight or App Store, set the isSandboxEnvironment to false before starting building. To debug locally, set it to true. Ignore this when the notifyWhenAppRunningInBackgroundOrQuit is false. |
certificateIndex | Enum | No | To configure different certificates for different bunldle ID applications. The certificateIndex can be set as firstCertificate or secondCertificate , defaults to firstCertificate . |
translationText | ZegotranslationText | No | To modify the UI text, use this property. For more details, see Custom prebuilt UI. |
videoConfig | ZegoPrebuiltCallVideoConfig | .PRESET_360P | This parameter is used to configure the push-pull flow resolution. |
Parameters of ZegoUIKitPrebuiltCallInvitationService class
Parameter | Type | Required | Description |
---|---|---|---|
appID | String | Yes | The App ID you get from ZEGOCLOUD Admin Console. |
appSign | String | Yes | The App Sign you get from ZEGOCLOUD Admin Console. |
userID | String | Yes | userID can be something like a phone number or the user ID on your own user system. userID can only contain numbers, letters, and underlines (_). |
userName | String | Yes | userName can be any character or the user name on your own user system. |
config | ZegoUIKitPrebuiltCallInvitationConfig | Yes | This can be used to set up call invitation related configurations. |
Add the button for making call invitation
You can customize the position of the ZegoSendCallInvitationButton
accordingly, pass in the ID of the user you want to call.
- Set up a button for making a voice call.
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Create Voice Call invitation Button
let callTargetUser: ZegoUIKitUser = ZegoUIKitUser.init("TargetUserID", "TargetUserName")
let sendVoiceCallButton: ZegoSendCallInvitationButton = ZegoSendCallInvitationButton(ZegoInvitationType.voiceCall.rawValue)
sendVoiceCallButton.text = "voice"
sendVoiceCallButton.setTitleColor(UIColor.blue, for: .normal)
sendVoiceCallButton.inviteeList.append(callTargetUser)
sendVoiceCallButton.resourceID = "zegouikit_call" // For offline call notification
sendVoiceCallButton.frame = CGRect.init(x: 100, y: 100, width: 100, height: 30)
// Add the button to your view
self.view.addSubview(sendVoiceCallButton)
}
}
- (void)viewDidLoad {
[super viewDidLoad];
// Create Voice Call invitation Button
ZegoUIKitUser *callTargetUser = [[ZegoUIKitUser alloc] initWithUserID:@"TargetUserID" userName:@"TargetUserName" isCameraOn:NO isMicrophoneOn:YES];
ZegoSendCallInvitationButton *sendVoiceCallButton = [[ZegoSendCallInvitationButton alloc] initWithInvitationType:ZegoInvitationTypeVoiceCall];
sendVoiceCallButton.frame = CGRectMake(100, 100, 100, 30);
[sendVoiceCallButton setText:@"voice"];
[sendVoiceCallButton setTitleColor:[UIColor blueColor] forState:UIControlStateNormal];
sendVoiceCallButton.resourceID = @"xxx";
sendVoiceCallButton.inviteeList = @[allTargetUser];
// Add the button to your view
[self.view addSubview:sendVoiceCallButton];
}
- Set up a button for making a video call.
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Create Video Call invitation Button
let callTargetUser: ZegoUIKitUser = ZegoUIKitUser.init("TargetUserID", "TargetUserName")
let sendVideoCallButton: ZegoSendCallInvitationButton = ZegoSendCallInvitationButton(ZegoInvitationType.videoCall.rawValue)
sendVideoCallButton.text = "Video"
sendVideoCallButton.setTitleColor(UIColor.blue, for: .normal)
sendVideoCallButton.resourceID = "xxx" // For offline call notification
sendVideoCallButton.frame = CGRect.init(x: 100, y: 100, width: 100, height: 30)
sendVideoCallButton.inviteeList.append(callTargetUser)
// Add the button to your view.
self.view.addSubview(sendVideoCallButton)
}
}
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
ZegoUIKitUser *user = [[ZegoUIKitUser alloc] initWithUserID:@"user id" userName:@"user nickname" isCameraOn:YES isMicrophoneOn:YES];
// Create video call invitation button.
ZegoSendCallInvitationButton * sendVideoCallButton = [[ZegoSendCallInvitationButton alloc] initWithInvitationType:ZegoInvitationTypeVideoCall];
sendVideoCallButton.frame = CGRectMake(100, 100, 100, 30);
[sendVideoCallButton setText:@"Video"];
sendVideoCallButton.resourceID = @"xxx";
sendVideoCallButton.inviteeList = @[user];
// Add the button to your view.
[self.view addSubview:sendVideoCallButton];
}
Parameters of ZegoSendCallInvitationButton class
Parameter | Type | Required | Description |
---|---|---|---|
inviteeList | Array | Yes | The information of the callee. userID and userName are required. For example: [{ userID: inviteeID, userName: inviteeName }] |
type | int | Yes | If the type is set to ZegoInvitationType.videoCall.rawValue , a video call is made when the button is pressed. If the type is set to other values, a voice call is made. |
resourceID | String | No | resourceID can be used to specify the ringtone of an offline call invitation, which must be set to the same value as the Push Resource ID in ZEGOCLOUD Admin Console. This only takes effect when the notifyWhenAppRunningInBackgroundOrQuit is true. |
timeout | UInt32 | No | The timeout duration. It's 60 seconds by default. |
For more parameters, go to Custom prebuilt UI.
Now, you can make call invitations by simply clicking on the buttons you set.
Customer call button
API
Configure your project
1. Apple Developer Center and ZEGOCLOUD Console Configuration
- step1. You need to refer to Create VoIP services certificates to create the VoIP service certificate, and export a .p12 file on your Mac.
- step2. Add the voip service certificate .p12 file. Then, create a resource ID;
In the create resource ID popup dialog, you should switch to the VoIP option for APNs, and switch to Data messages for FCM.
When you have completed the configuration, you will obtain the resourceID
. You can refer to the image below for comparison.
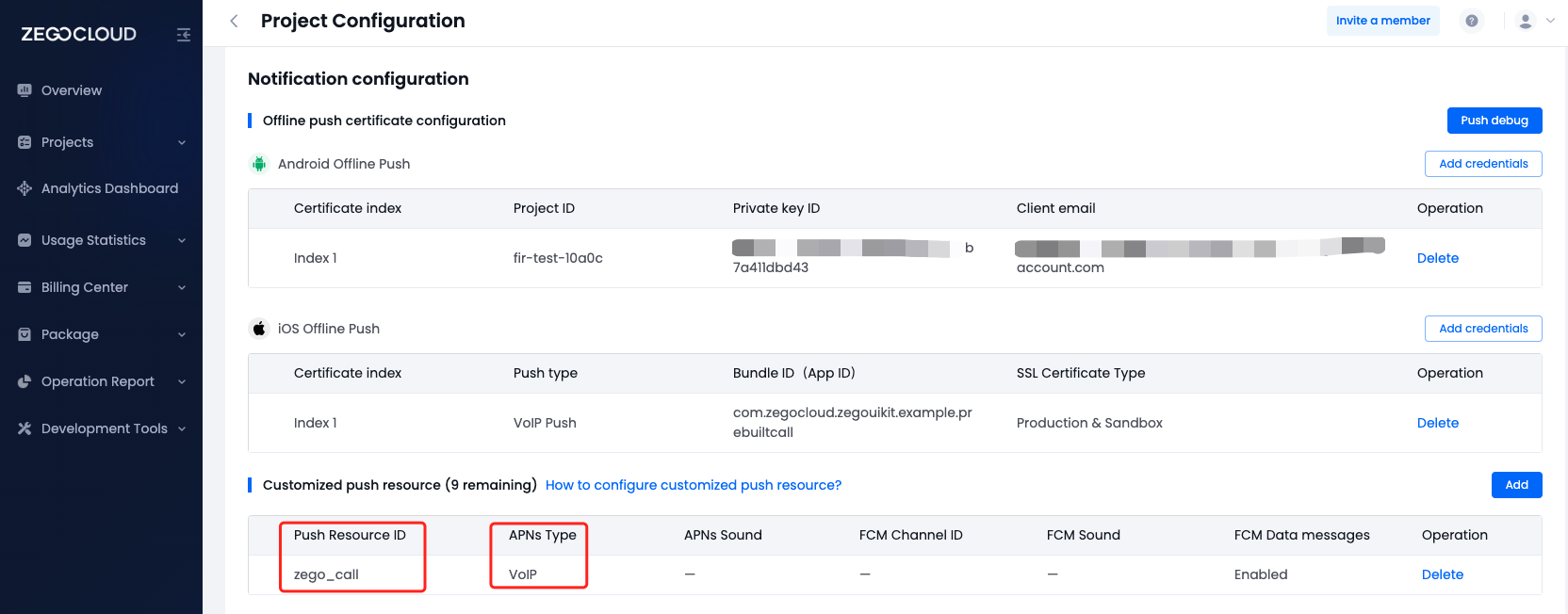
2. Add app permissions
Open the Info.plist
file and add the following:
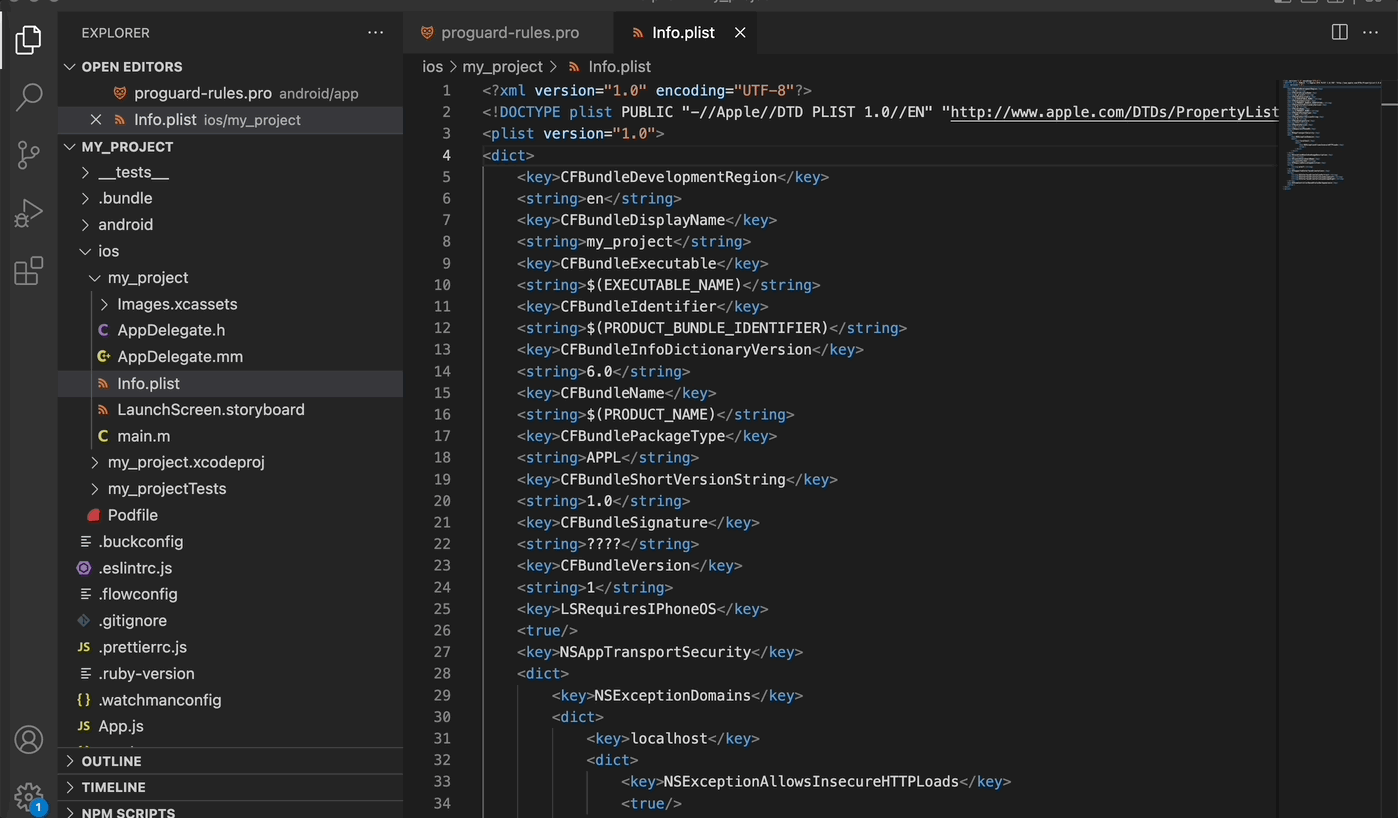
<key>NSCameraUsageDescription</key>
<string>We need to use the camera</string>
<key>NSMicrophoneUsageDescription</key>
<string>We need to use the microphone</string>
3. Get the APNs device token
- step1. In the
AppDelegate.swift
file, implement Apple's register callback for receiving thedeviceToken
:
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data){
}
- step2. In the
didRegisterForRemoteNotificationsWithDeviceToken
callback, call thesetRemoteNotificationsDeviceToken
method to get thedeviceToken
:
import ZegoUIKitPrebuiltCall
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
/// Required - Set the device token
ZegoUIKitPrebuiltCallInvitationService.setRemoteNotificationsDeviceToken(deviceToken)
}
4. Add Push Notifications configuration
Open the project with Xcode, and click the+ Capability
on the Signing & Capabilities
page.
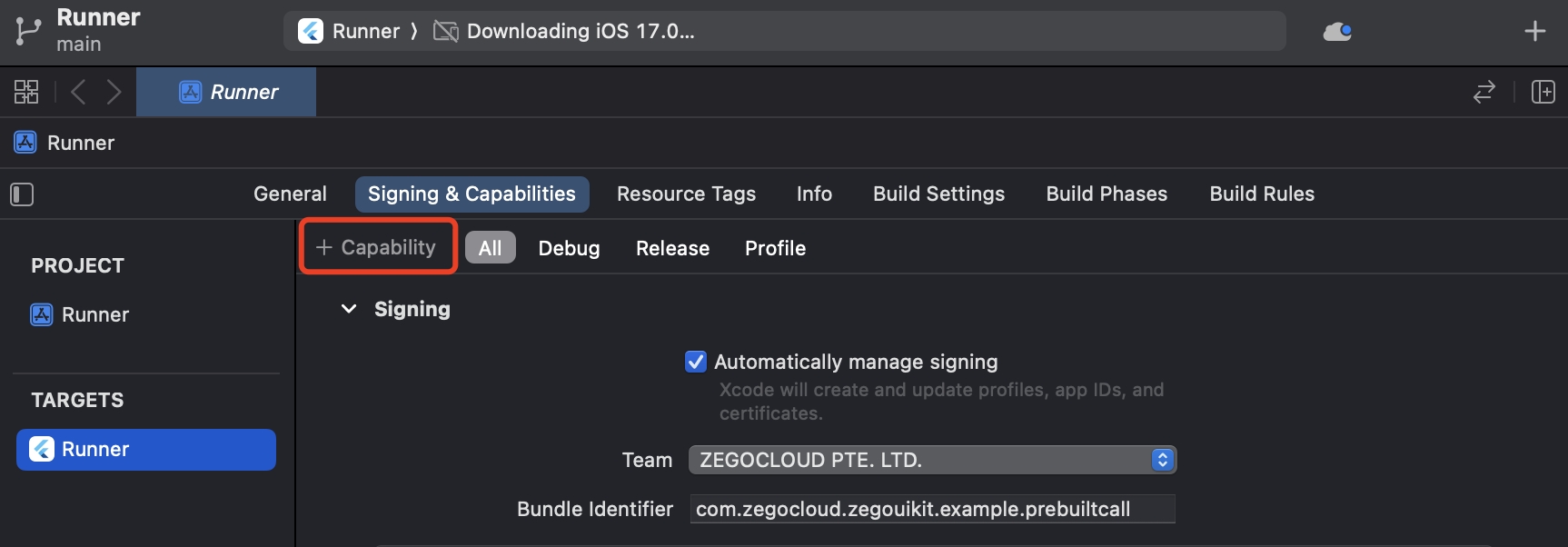
And double-click on Push Notifications
to add this feature.
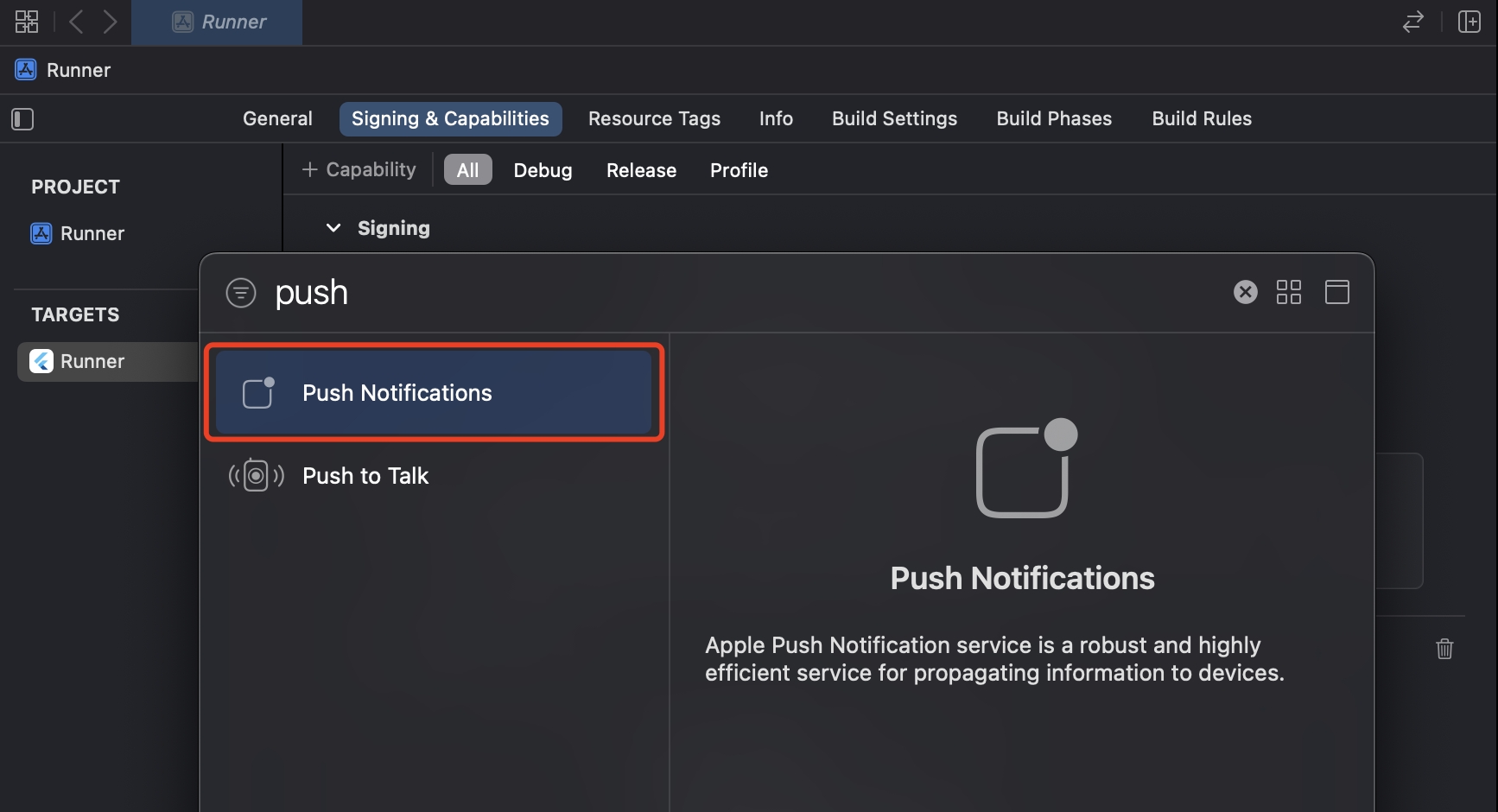
5. Add the Background Modes capability.
Open the project with Xcode, and click the+ Capability
on the Signing & Capabilities
page again.
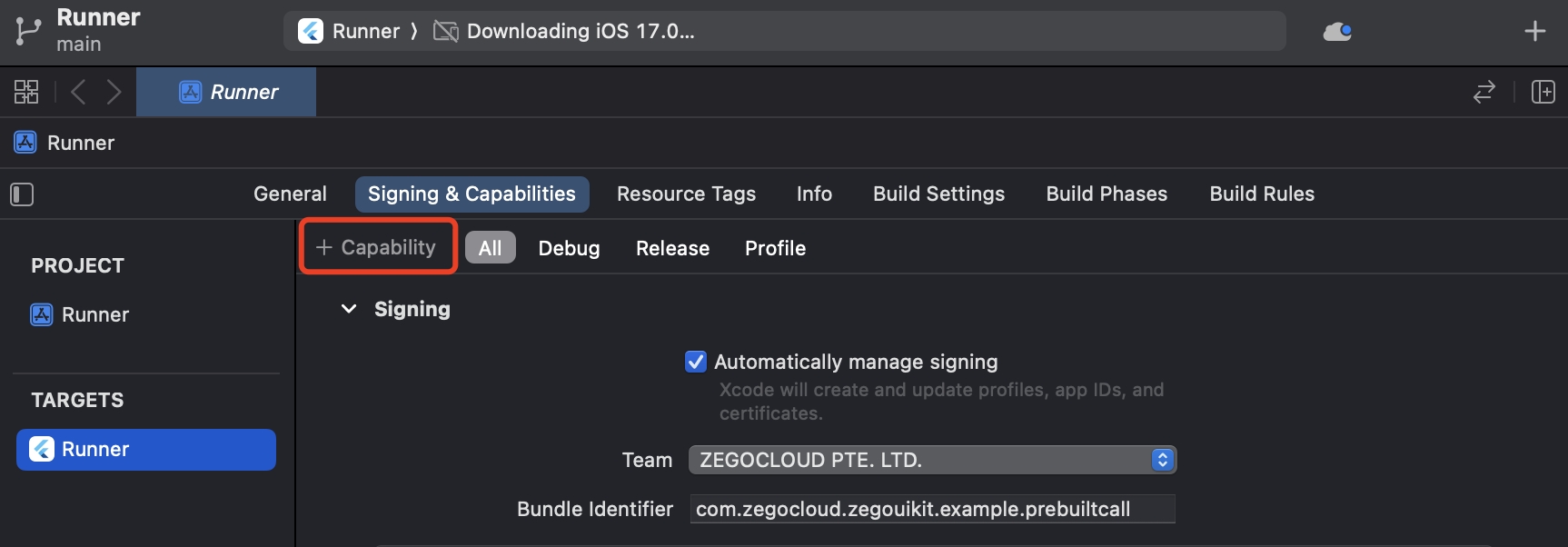
And double-click on Background Modes
in the pop-up window. This will allow you to see the Background Modes
configuration in the Signing & Capabilities
.
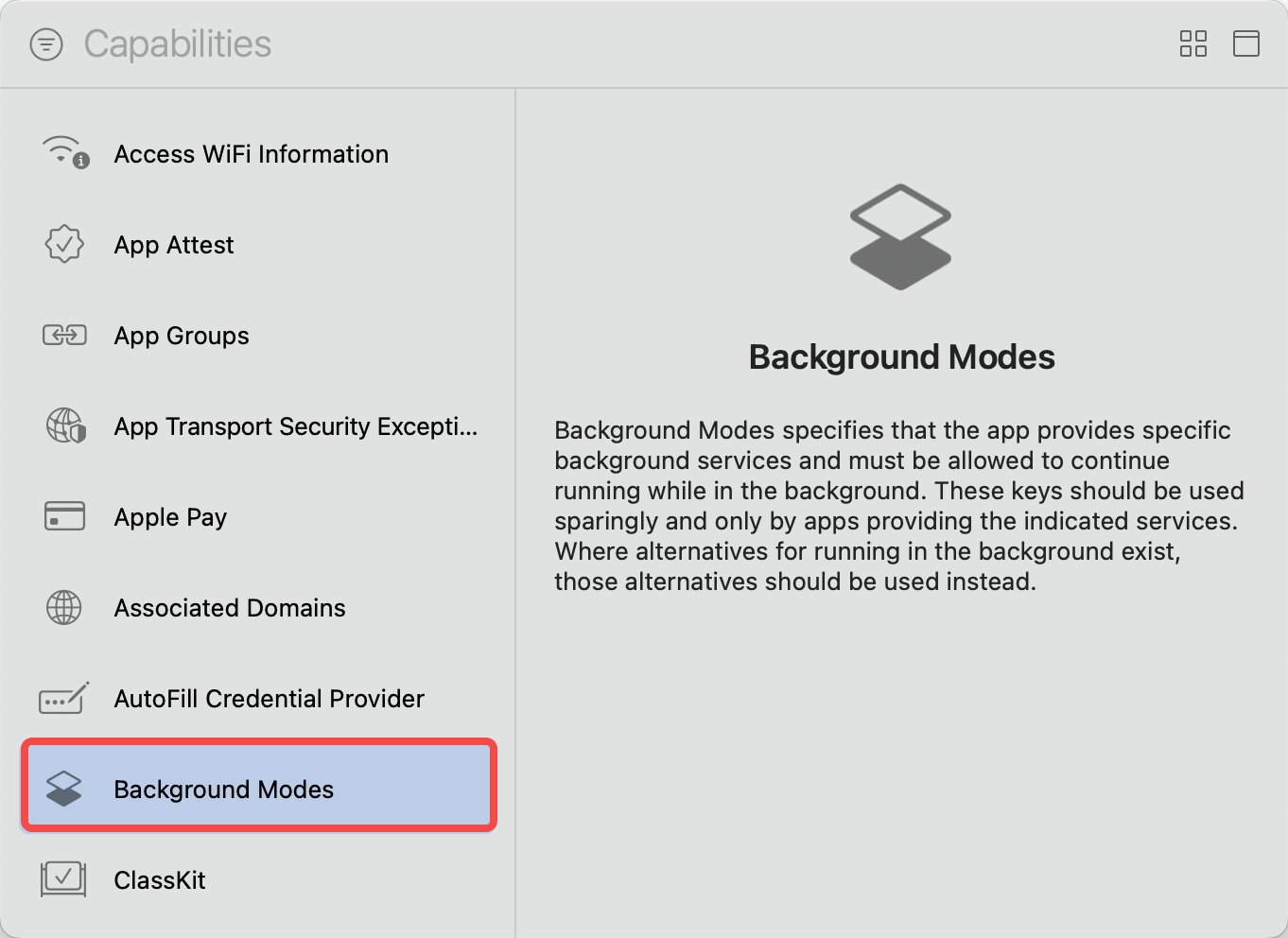
6. Check and Make sure the following features are enabled
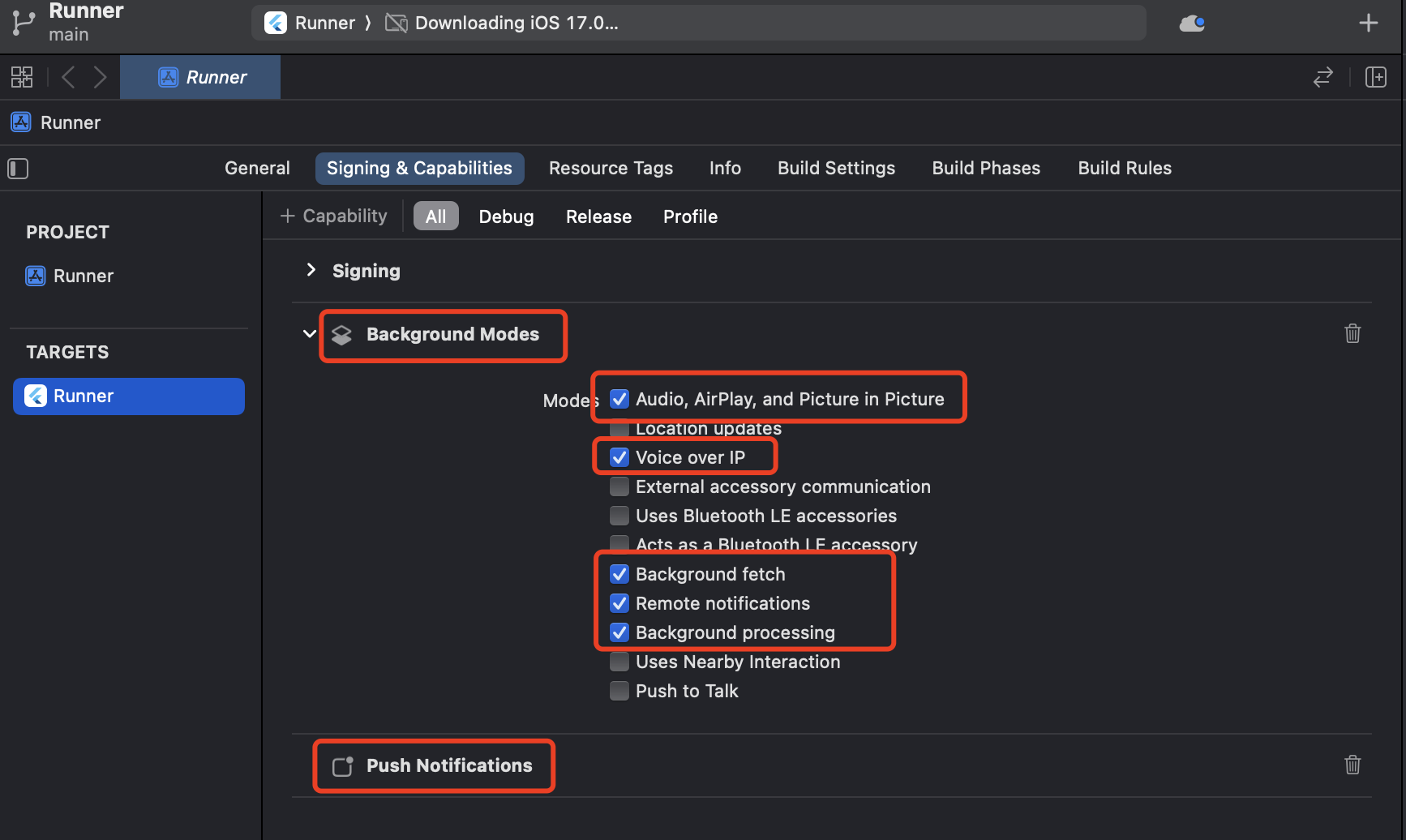
7. Import the PushKit and CallKit libraries.
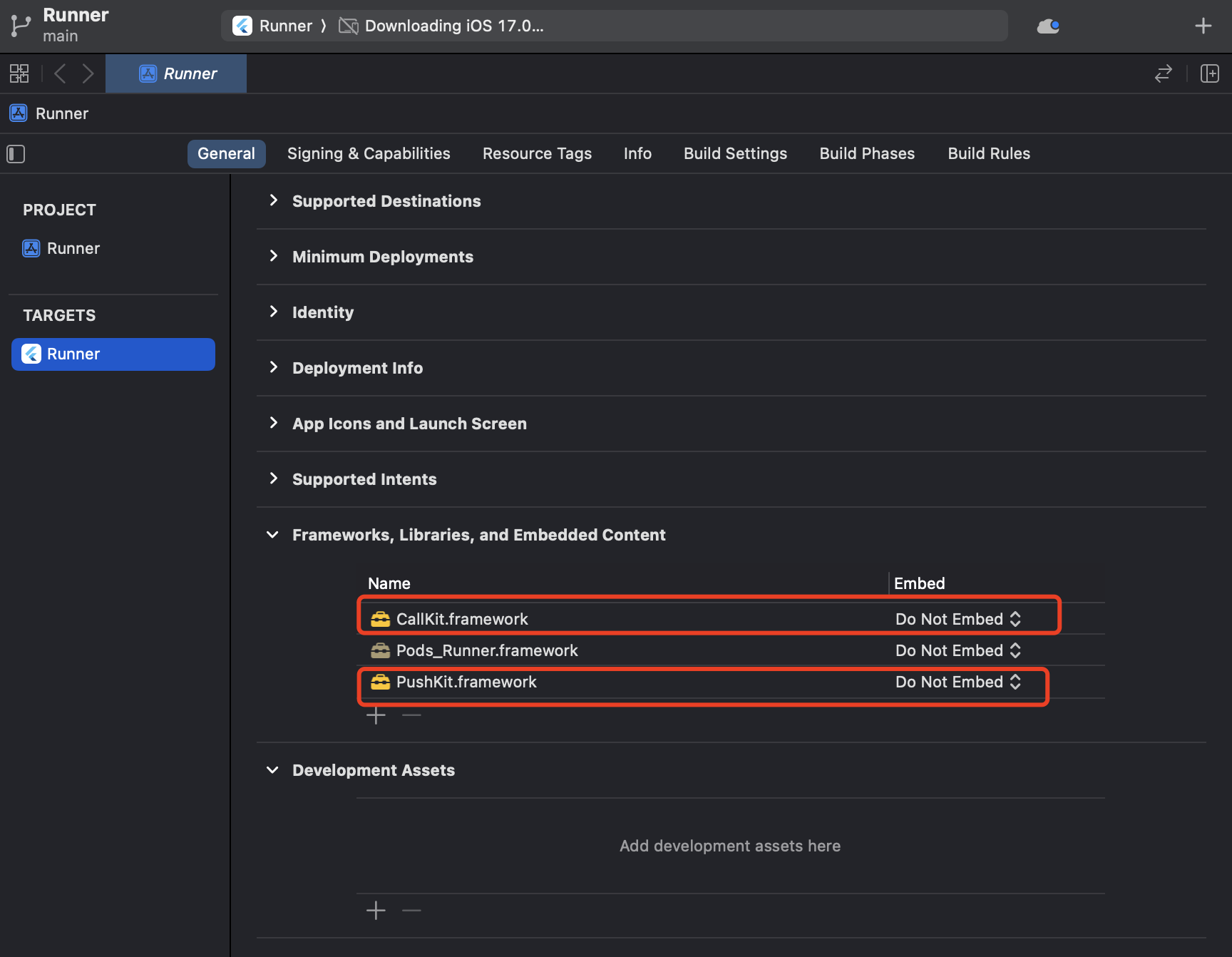
Run & Test
Now you have finished all the steps!
You can simply click the Run in XCode to run and test your App on your device.
Related guides
Content of the Card.
Resources
Click here to get the complete sample code.