Manage group members
ZEGOCLOUD's In-app Chat (the ZIM SDK) provides the capability of group member management, allowing you to query the group member list and group member info, change the group owner, and more.
- Before you use the following features, you must join a group first. To join a group, refer to the Chapter Join a group of Manage groups.
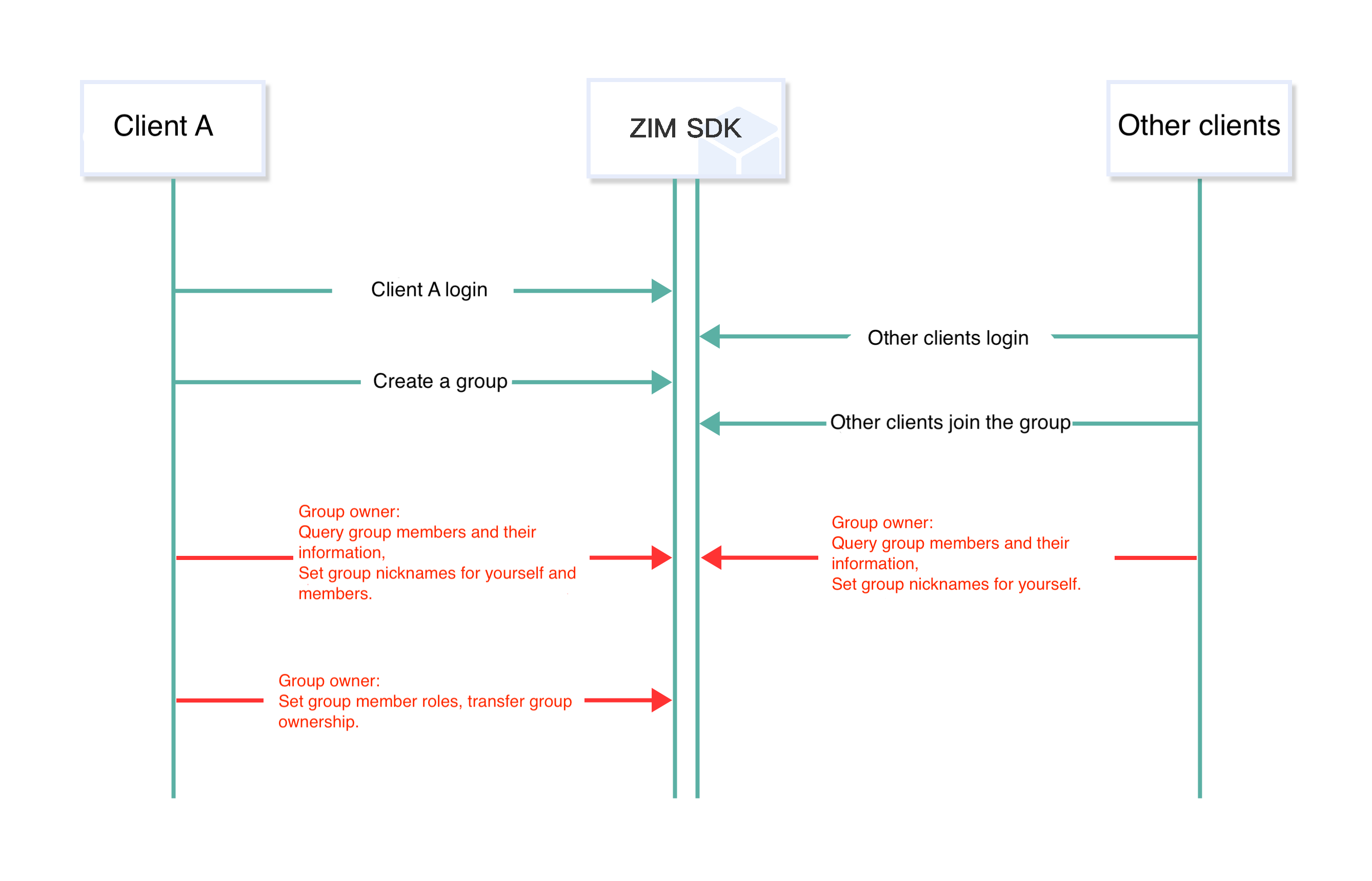
Get group member list
To know who is in the current group and get the group member list after login, call the queryGroupMemberList method.
To know the results of the query, you can check the return value of the class queryGroupMemberList.
Sample code
// Get group member list.
ZIMGroupMemberQueryConfig groupMemberQueryConfig =
ZIMGroupMemberQueryConfig();
groupMemberQueryConfig.count = 100;
ZIM
.getInstance()
.queryGroupMemberList('groupID', groupMemberQueryConfig)
.then((value) {
//This will be triggered when operation successful.
})
.catchError((onError) {
//This will be triggered when operation failed.
});
Get group member info
To know the info of a specified member after joining a group, call the queryGroupMemberInfomethod.
To know the results of the query, you can check the return value of the class ZIMGroupMemberInfoQueriedResult.
Sample code
// Get group member info.
ZIM
.getInstance()
.queryGroupMemberInfo('userID', 'groupID')
.then((value) {
//This will be triggered when operation successful.
})
.catchError((onError) {
//This will be triggered when operation failed.
});
Set a nickname
To set a nickname after joining a group, call the setGroupMemberNickname method.
The group owner can set the nickname of all group members. But the group members can only set their own nicknames.
To know whether the nickname is set successfully, you can check the return value of the class ZIMGroupMemberNicknameUpdatedResult.
Sample code
// Set a nickname. Note: The group owner can set the nickname of all group members. But the group members can only set their own nicknames.
ZIM
.getInstance()
.setGroupMemberNickname('nickname', 'forUserID', 'groupID')
.then((value) {
//This will be triggered when operation successful.
})
.catchError((onError) {
//This will be triggered when operation failed.
});
Set a role for a group member
For a group owner to set a role for a group member, call the setGroupMemberRole method. You can set the group member to a regular member or admin.
After the setting is successful, the group owner can receive the results of the operation through the return value of the class ZIMGroupMemberRoleUpdatedResult.
Group members can receive notifications of user role changes through onGroupMemberInfoUpdated.
Description of group member roles and permissions
The ZIM SDK supports setting users as group owners, administrators, and regular members by default. In a group, the group owner has all client permissions and can perform all group functions. Administrators have most client permissions, while regular members have the fewest client permissions. The specific client permissions for each role are shown in the table below:
Client Permissions | Group Owner (corresponding enum value: 1) | Administrator (corresponding enum value: 2) | Regular Member (corresponding enum value: 3) |
---|---|---|---|
Modify group avatar, group name, group notice | Supported | Supported | Supported |
Modify group attributes | |||
Modify group member nickname | Supported, can be used for all group role users | Supported, can be used for all regular members | Supported, can only be used for oneself |
Recall group member messages | |||
Kick out members | Not supported | ||
Mute individual group members | |||
Mute specific group roles | |||
Set group member roles | Not supported | ||
Transfer group ownership | |||
Dismiss the group | |||
Mute all members |
setGroupMemberRole description
Sample code
// The group owner sets regular users as administrators.
ZIM
.getInstance()
.setGroupMemberRole(2, 'forUserID', 'groupID')
.then((value) {
//This will be triggered when operation successful.
})
.catchError((onError) {
//This will be triggered when operation failed.
});
Transfer the group ownership
For a group owner to transfer the group ownership, call the transferGroupOwner method with the toUserID
(the ID of the member you want to transfer the ownership to).
- Only the group owner has permission to change the group owner.
- The member you want to transfer the ownership to must be in the group; otherwise, the operation fails.
All group members can be told whether the group owner is changed through the return value of the class ZIMGroupOwnerTransferredResult.
Sample code
// Change the group owner.
ZIM
.getInstance()
.transferGroupOwner('toUserID', 'groupID')
.then((value) {
//This will be triggered when operation successful.
})
.catchError((onError) {
//This will be triggered when operation failed.
});
Set Group Member Mute Status
After logging into the ZIM SDK, users can mute or unmute specific members within the groups they manage. By calling the muteGroupMembersinterface, you can batch modify the mute status of up to 100 group members. The mute duration can be permanent or up to a maximum of 604,800 seconds (7 days).
- Group owners can mute all group members, including themselves.
- If you need to increase the number of operations per request or extend the maximum mute duration, please contact ZEGOCLOUD technical support.
After a successful setting, the operating user will receive a notification through ZIMGroupMembersMutedResult.
After muting or unmuting, all group members will receive onGroupMemberInfoUpdated, which informs them of which members are unable to speak or have their mute status lifted in the group.
If you want to mute certain group members , please refer Manage groups - Mute group.
try {
bool isMute = true;
List<String> userIDs = ['user_1','user_2','user_3'];
String groupID = 'group_id';
ZIMGroupMemberMuteConfig config = ZIMGroupMemberMuteConfig();
// Mute duration of 30 seconds.
config.duration = 30;
ZIMGroupMembersMutedResult result = await ZIM.getInstance()!.muteGroupMembers(isMute, userIDs, groupID, config);
// Developers can obtain mute result-related information from the parameters in the result.
} on PlatformException catch (onError){
onError.code;//Handle according to the error code table.
onError.message;//Error message.
}
Query the list of muted members in a group
After logging into the ZIM SDK, if group members want to know the list of members muted in the group, they can call the queryGroupMemberMutedList interface to query.
After a successful query, the operating user can retrieve specific information using ZIMGroupMemberMutedListQueriedResult.
try {
// Query the list of members muted in the group by group members.
ZIMGroupMemberMutedListQueryConfig config = ZIMGroupMemberMutedListQueryConfig();
//Get up to 100 members in one request
config.count = 100;
config.nextFlag = 0;
ZIMGroupMemberMutedListQueriedResult result = await ZIM.getInstance()!.queryGroupMemberMutedList('gorupID', config);
} on PlatformException catch (onError){
onError.code; // Handle according to the error code table.
onError.message; // Error message.
}