Get message history
Function overview
This document applies to the following platforms: iOS, Android, macOS, Windows and Web.
This document describes how to query the message history and specific messages of one-to-one, group, and in-room chats with the ZIM SDK.
This feature allows you to get historical messages of types other than command messages and pop-up messages.
Get the full message history
After logging in to ZIM SDK, users can use the queryHistoryMessage method to retrieve the message history of one-to-one chats
, group chats
, and rooms chats
by providing the parameters conversationID and config.
Taking the example of client A retrieving the conversation history with client B in a one-on-one chat:
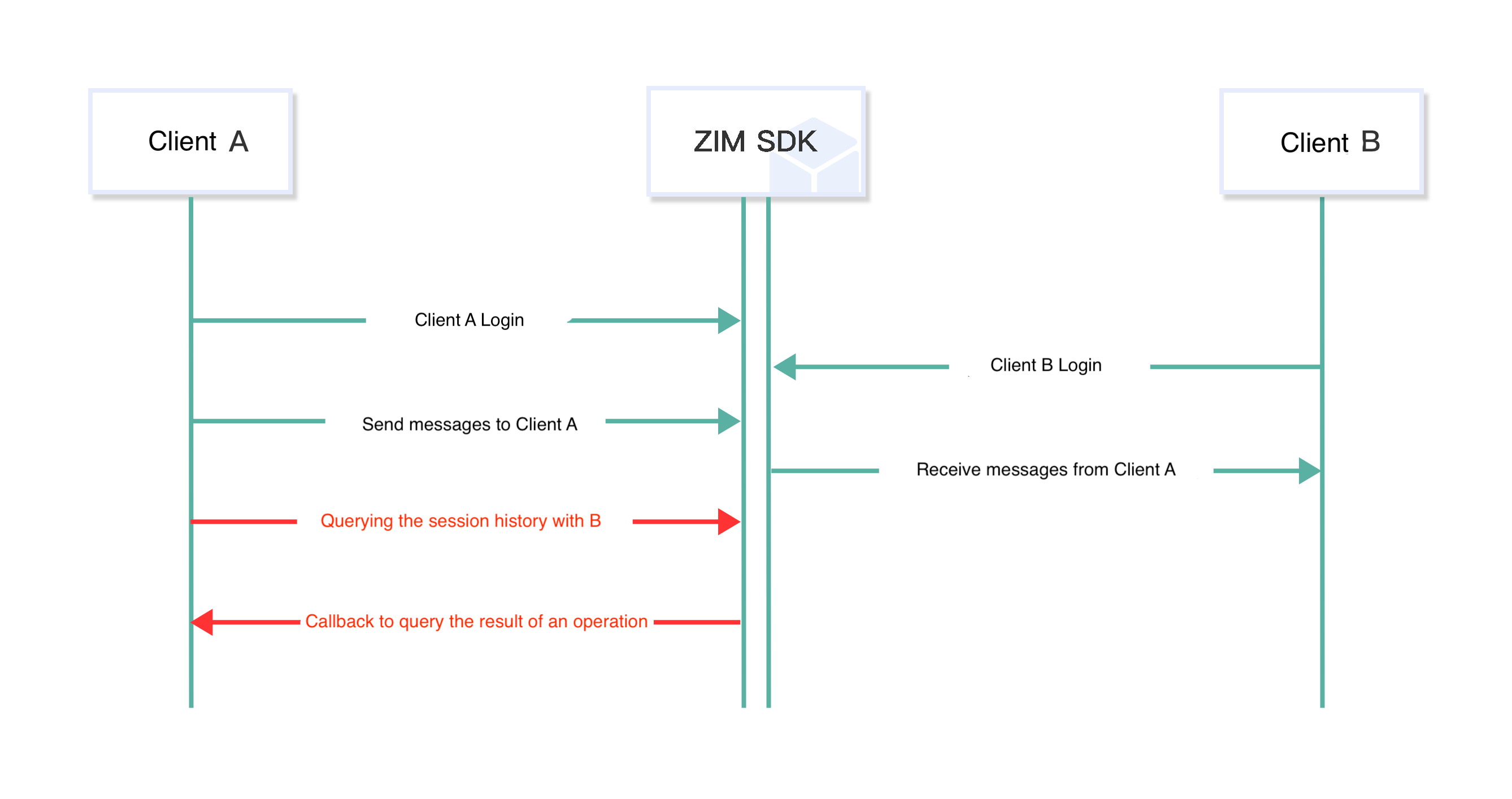
- Client A and B log in to the ZIM SDK and send/receive one-on-one chat messages to each other.
- When client A needs to retrieve the conversation records with B:
- Client A first logs in to the ZIM SDK.
- Client A calls the queryHistoryMessage interface and passes the conversationID and config parameters to start retrieving.
- The retrieved results will be notified to client A through the ZIMMessageQueriedResult callback interface.
// Get the historical messages of a one-on-one chat
// Get the conversation history messages from the back to the front, retrieve 30 messages each time
ZIMMessageQueryConfig config = ZIMMessageQueryConfig();
// For the first retrieval, nextMessage is null
config.nextMessage = null;
config.count = 30;
config.reverse = true;
await ZIM
.getInstance()!
.queryHistoryMessage('conversationID', ZIMConversationType.peer, config)
.then((value) => {
// Triggered when successful
// Developers can listen to this callback to obtain the retrieved message list.
// When the finger scrolls down to the topmost message on the screen, retrieve earlier messages
// Save the frontmost message as the anchor for the messages
if (fetchMore) {
// For subsequent pagination retrieval, nextMessage is the first message in the currently retrieved message list
config.nextMessage = value.messageList[0],
// Recursive retrieval
}
})
.catchError((onError) {
// Triggered when failed
});
Get specific messages
ZIM supports querying specific messages in a one-to-one or group conversation based on messageSeq
(the sequence number of the message in the conversation) list (up to a maximum of 10) by calling queryMessages.
This interface is used when you only know the messageSeq
of a message and do not know the complete structure of the message. For example, if a message in a conversation replies to a historical message, members of the conversation can use the repliedInfo.messageSeq
of the reply to obtain the messageSeq
of the historical message. At this time, you can call this interface to obtain the complete structure of the historical message.
List<int> messageSeqs = []; // The maximum length is 10
String conversationID = "YOUR_CONVERSATION_ID";
ZIMConversationType conversationType = ZIMConversationType.peer; // Conversation type: one-to-one: ZIMConversationTypePeer, group: ZIMConversationTypeGroup
ZIM.getInstance()?.queryMessages(messageSeqs, conversationID, conversationType).then((value) {
// Query successful
}).catchError((onError){
if(onError is PlatformException){
// Query failed
}
});