Get message history
Function overview
This document describes how to query the message history and specific messages of one-to-one, group, and in-room chats with the ZIM SDK.
This feature allows you to get historical messages of types other than command messages and pop-up messages.
Get the full message history
After logging in to ZIM SDK, users can use the queryHistoryMessage method to retrieve the message history of one-to-one chats
, group chats
, and rooms chats
by providing the parameters conversationID and config.
Taking the example of client A retrieving the conversation history with client B in a one-on-one chat:
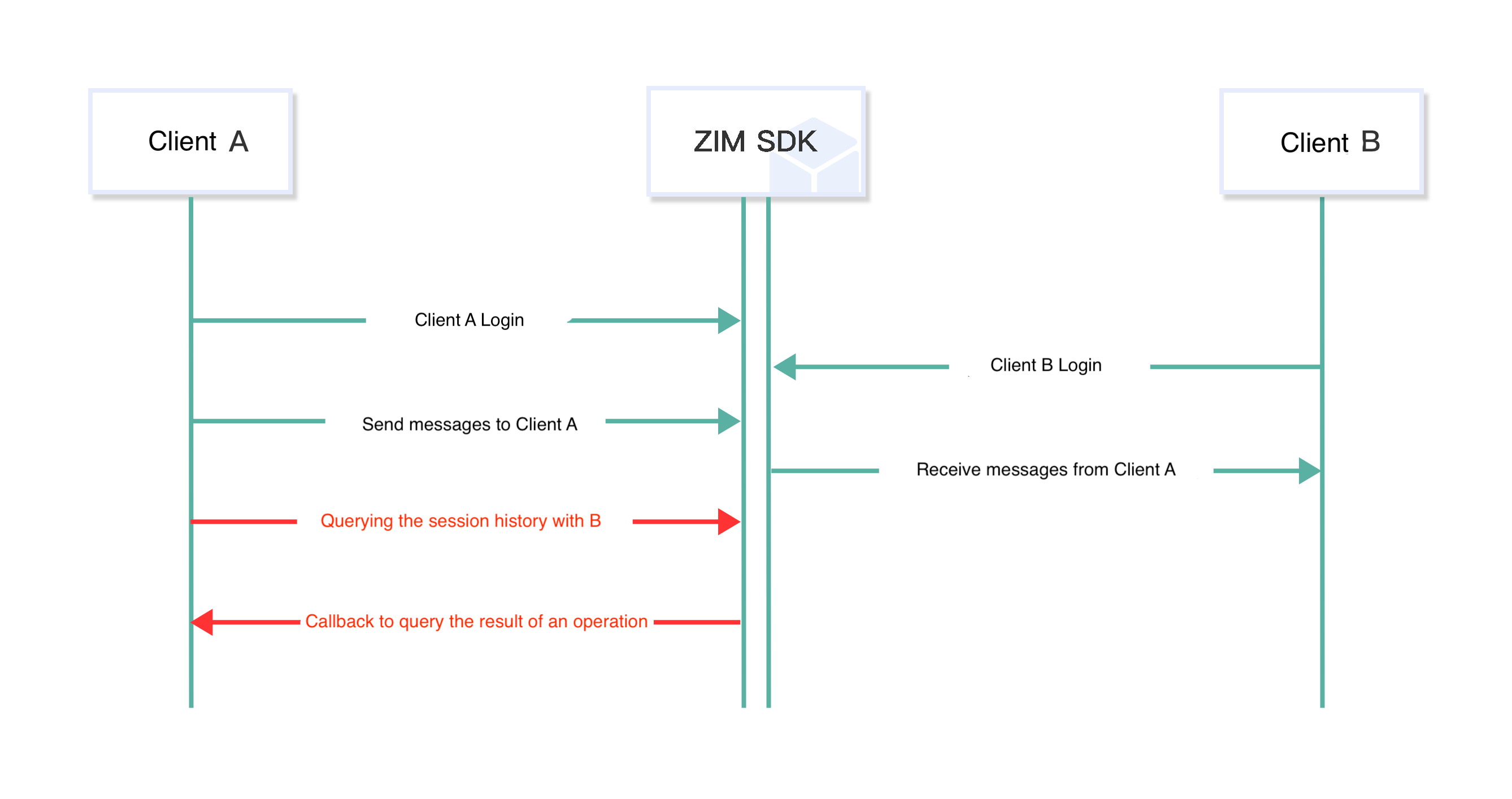
- Client A and B log in to the ZIM SDK and send/receive one-on-one chat messages to each other.
- When client A needs to retrieve the conversation records with B:
- Client A first logs in to the ZIM SDK.
- Client A calls the queryHistoryMessage interface and passes the conversationID and config parameters to start retrieving.
- The retrieved results will be notified to client A through the ZIMMessageQueriedResult callback interface.
// Retrieve historical messages for one-on-one chats
var curMessageList = [];
var conversationID = '';
var conversationType = 0;
// Retrieve 30 messages each time, starting from the latest message
var config = {
nextMessage: null, // Set nextMessage to null for the first retrieval
count: 30,
reverse: true
}
function queryMessageCallback({ messageList }) {
curMessageList.push(...messageList);
// When scrolling down to the topmost message on the screen, retrieve earlier messages
if (fetchMore && messageList.length > 0) {
// For subsequent pagination, set nextMessage to the first message in the current retrieved message list
config.nextMessage = messageList[0];
zim.queryHistoryMessage(conversationID, conversationType, config).then(queryMessageCallback);
}
}
zim.queryHistoryMessage(conversationID, conversationType, config).then(queryMessageCallback);
Get specific messages
ZIM supports querying specific messages in a one-to-one or group conversation based on messageSeq
(the sequence number of the message in the conversation) list (up to a maximum of 10) by calling queryMessages.
This interface is used when you only know the messageSeq
of a message and do not know the complete structure of the message. For example, if a message in a conversation replies to a historical message, members of the conversation can use the repliedInfo.messageSeq
of the reply to obtain the messageSeq
of the historical message. At this time, you can call this interface to obtain the complete structure of the historical message.
var messageSeqs = []; // The maximum length is 10
var conversationID = '';
var conversationType = 0; // Conversation type: one-to-one: 0, group: 2
zim.queryMessages(messageSeqs, conversationID, conversationType)
.then(({ messageList }) => {
// Query successful
})
.catch((err) => {
// Query failed
});