Authentication
Overview
To avoid unauthorized service access or operations, ZEGOCLOUD uses digital Tokens to control and validate users' login privileges.
The validation process
Before you log in to a room, your app clients request Tokens from your app server and provide the Token for privilege validation when logging in to a room.
The following diagram shows the process of room login privilege validation:
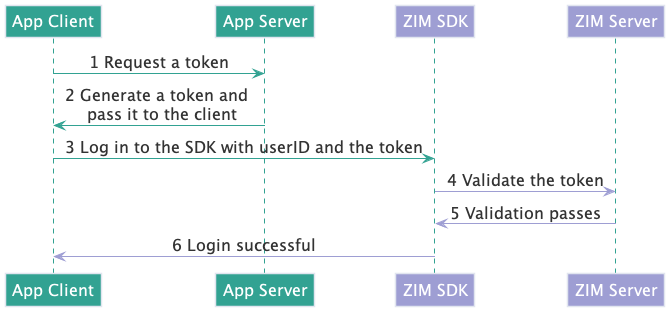
Generate a Token
For business security, you must generate Tokens on your app server.
-
Go to ZEGOCLOUD Admin Console, and do the following:
- Create a project, get the AppID and AppSign.
- Subscribe to the In-app Chat service.
-
Use the
token generator
plug-in provided by ZEGOCLOUD to generate Tokens on your app server.
Language | Supported version | Core function | Code base | Sample code | |
---|---|---|---|---|---|
User identity Token | User privilege Token | ||||
Go | Go 1.14.15 or later | GenerateToken04 | |||
C++ | C++ 11 or later | GenerateToken04 | |||
Java | Java 1.8 or later | generateToken04 | |||
Python | Python 3.6.8 or later | generate_token04 | |||
PHP | PHP 7.0 or later | generateToken04 | |||
.NET | .NET Framework 3.5 or later | GenerateToken04 | |||
Node.js | Node.js 8 or later | generateToken04 |
Take Go language as an example, you can do the following steps to generate a Token:
- go get github.com/ZEGOCLOUD/zego_server_assistant
- import "github.com/ZEGOCLOUD/zego_server_assistant/token/go/src/token04"
- Call the
GenerateToken04
method to generate a Token.
The following code shows how to generate a user identity Token:
var appId uint32 = <Your AppId> // type: uint32
userId := <Your userID> // type: string
secret := <ServerSecret> // type: 32 byte length string
var effectiveTimeInSeconds int64 = <Your token effectiveTime> //type: int64; unit: s
token, err := zsa.GenerateToken04(appId, userId, secret, effectiveTimeInSeconds)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(token)
Generating Token on the Developer's Browser
The following example code is for reference only. The token validity period cannot exceed 24 days. To ensure security, ZEGOCLOUD strongly recommends that you generate tokens on your own server.
Please refer to the following code example for generating tokens on the browser:
import CryptoJS from 'crypto-js';
const appConfig = {
appID: 0, // Fill in the AppID you applied for
serverSecret: '', // Fill in the ServerSecret you applied for
};
/**
* Generate token
*
* Token = "04" + Base64.encode(expire_time + IV.length + IV + binary_ciphertext.length + binary_ciphertext)
* Algorithm: AES<ServerSecret, IV>(token_json_str), using mode: CBC/PKCS5Padding
*
* This code example only provides a client-side token generation example. Please make sure to generate tokens on your own server to avoid leaking your ServerSecret.
*/
export function generateToken(userID, seconds) {
if (!userID) return '';
// Construct encrypted data
const time = (Date.now() / 1000) | 0;
const body = {
app_id: appConfig.appID,
user_id: userID,
nonce: (Math.random() * 2147483647) | 0,
ctime: time,
expire: time + (seconds || 7200), // The validity period should not exceed 24 days
};
// Encrypt the body
const key = CryptoJS.enc.Utf8.parse(appConfig.serverSecret);
let iv = Math.random().toString().substring(2, 18);
if (iv.length < 16) iv += iv.substring(0, 16 - iv.length);
const ciphertext = CryptoJS.AES.encrypt(JSON.stringify(body), key, { iv: CryptoJS.enc.Utf8.parse(iv) }).toString();
const ciphert = new Uint8Array(Array.from(atob(ciphertext)).map((val) => val.charCodeAt(0)));
const len_ciphert = ciphert.length;
// Assemble token data
const uint8 = new Uint8Array(8 + 2 + 16 + 2 + len_ciphert);
// expire: 8
uint8.set([0, 0, 0, 0]);
uint8.set(new Uint8Array(new Int32Array([body.expire]).buffer).reverse(), 4);
// iv length: 2
uint8[8] = iv.length >> 8;
uint8[9] = iv.length - (uint8[8] << 8);
// iv: 16
uint8.set(new Uint8Array(Array.from(iv).map((val) => val.charCodeAt(0))), 10);
// ciphertext length: 2
uint8[26] = len_ciphert >> 8;
uint8[27] = len_ciphert - (uint8[26] << 8);
// ciphertext
uint8.set(ciphert, 28);
const token = `04${btoa(String.fromCharCode(...Array.from(uint8)))}`;
console.log('generateToken', iv.length, body, token);
return token;
}
Setting Token
The token validity period cannot exceed 24 days. To ensure security, ZEGOCLOUD strongly recommends that you generate tokens on your own server.
When a user logs in, they should pass the corresponding token for authentication.
var useID = 'xxxx';
var config = {
userName: 'xxxx',
token: '',// The validity period should not exceed 24 days. Request from the developer's server to obtain the token
}
zim.login(useID, config)
.then(function() {
// Login successful
})
.catch(function(err) {
// Login failed
});
Handling Token Expiration
30 seconds before the token expires, the SDK will send a notification through the tokenWillExpire callback. If the token expires within 30 seconds after a successful login, the callback will be triggered immediately.
Upon receiving this callback, developers need to obtain a new valid token from their own server and use the renewToken interface provided by the SDK to update the token.
When the token expires and is not updated, the user will be disconnected and receive the connectionStateChanged callback, where the event is TokenExpired
and the state is Disconnected
.
// Register the callback for "Token Will Expire"
zim.on('tokenWillExpire', function(zim, second) {
var token = ''; // The validity period should not exceed 24 days. Request a new token from the developer's server
zim.renewToken(token)
.then(function({ token }) {
// Token updated successfully
})
.catch(function(err) {
// Token update failed
});
})
API Reference
Method | Description |
---|---|
login | Log in to the ZIM service. |
renewToken | Update the authentication token. |
tokenWillExpire | Token expiration callback. |