Advanced beauty effects
What are advanced beauty effects?
In addition to the basic beauty effects provided by the Live Streaming Kit, you can also choose to use advanced beauty effects to enhance your live streaming app. Advanced beauty effects include the following features: face beautification, face shape retouch, makeup, filters, stickers, and background segmentation.
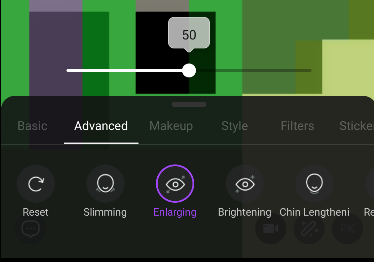
Prerequisites
Before you begin, make sure you complete the following:
- Follow the integration steps by referring to the Quick start or Quick start with co-hosting.
- Contact ZEGOCLOUD Technical Support to activate the advanced beauty effects.
- Download the demo here.
Getting started
Integrate the SDK
Add the dependency
implementation 'com.github.ZEGOCLOUD:zego_uikit_beauty_plugin_android:+'
Add the initialization code
As the initialization code has been added during Quick Start, you only need to add the beauty button in the ZegoUIKitPrebuiltCallConfig.bottomMenuBarConfig
.
//...
ZegoUIKitPrebuiltCallInvitationConfig callInvitationConfig = new ZegoUIKitPrebuiltCallInvitationConfig();
// add `ZegoMenuBarButtonName.BEAUTY_BUTTON` to host
callInvitationConfig.provider = new ZegoUIKitPrebuiltCallConfigProvider() {
@Override
public ZegoUIKitPrebuiltCallConfig requireConfig(ZegoCallInvitationData invitationData) {
ZegoUIKitPrebuiltCallConfig config = null;
boolean isVideoCall = invitationData.type == ZegoInvitationType.VIDEO_CALL.getValue();
boolean isGroupCall = invitationData.invitees.size() > 1;
if (isVideoCall && isGroupCall) {
config = ZegoUIKitPrebuiltCallConfig.groupVideoCall();
} else if (!isVideoCall && isGroupCall) {
config = ZegoUIKitPrebuiltCallConfig.groupVoiceCall();
} else if (!isVideoCall) {
config = ZegoUIKitPrebuiltCallConfig.oneOnOneVoiceCall();
} else {
config = ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall();
}
config.bottomMenuBarConfig.buttons = Arrays.asList(ZegoMenuBarButtonName.TOGGLE_CAMERA_BUTTON,
ZegoMenuBarButtonName.SWITCH_CAMERA_BUTTON, ZegoMenuBarButtonName.HANG_UP_BUTTON,
ZegoMenuBarButtonName.TOGGLE_MICROPHONE_BUTTON, ZegoMenuBarButtonName.BEAUTY_BUTTON);
return config;
}
};
ZegoUIKitPrebuiltCallService.init(getApplication(), appID, appSign, userID, userName,callInvitationConfig);
//...
Add beauty effects resources
Advanced beauty effects require corresponding beauty resources to be effective.
Download resources
Click to download the beauty resources, and extract the resources to your local folder.
- Adding resources to Android:
-
Create an assets folder under the
main
directory of your Android project (no need to create one if the assets folder already exists), like this: xxx/android/app/src/main/assets -
Copy the downloaded
BeautyResources
folder to the assets directory.
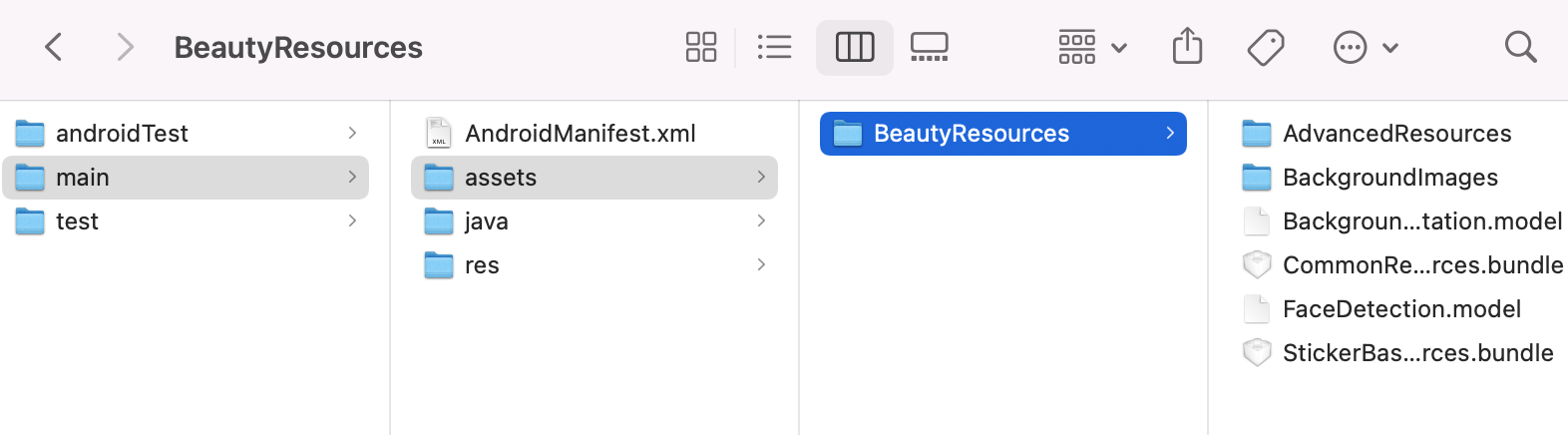
Run & Test
So far, you can simply click the Run or Debug button to run and test your App on the device.
Customize the beauty effects as needed
FYI: Our beauty effects package includes two plans: Basic pan and Pro plan. The Basic plan only includes basic beauty effects, while the Pro plan includes additional features such as face beautification, face shape retouch, facial feature enhancement, makeup looks, stickers, and filters. For more details, refer to AI Effects.
To customize the beauty effects, config the beautyConfig
in ZegoUIKitPrebuiltCallConfig
.
Beauty effects
Beauty effects included the 4 features: basic beauty effects, advanced beauty effects (face shape retouch), facial feature enhancement, and makeup looks. You can enable all four features or choose only a few of them.
The Basic Plan only includes the basic beauty effects. If you require other features, choose the Pro Plan instead.
Example 1: Selecting basic beauty effects and advanced beauty effects
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.addAll(ZegoBeautyPluginConfig.basicTypes());
customTypes.addAll(ZegoBeautyPluginConfig.advancedTypes());
config.beautyConfig.effectsTypes = customTypes;
//...
Here's how it would look like:
![]() | ![]() |
Example 2: Selecting all four features
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.addAll(ZegoBeautyPluginConfig.basicTypes());
customTypes.addAll(ZegoBeautyPluginConfig.advancedTypes());
customTypes.addAll(ZegoBeautyPluginConfig.makeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.styleMakeupTypes());
config.beautyConfig.effectsTypes = customTypes;
//...
Here's how it would look like:
![]() | ![]() |
Filters
The filters include a total of 10 different filter effects, You can use the following code to set them:
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.addAll(ZegoBeautyPluginConfig.basicTypes());
customTypes.addAll(ZegoBeautyPluginConfig.advancedTypes());
customTypes.addAll(ZegoBeautyPluginConfig.makeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.styleMakeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.filterTypes());
config.beautyConfig.effectsTypes = customTypes;
//...
Here's how it would look like:
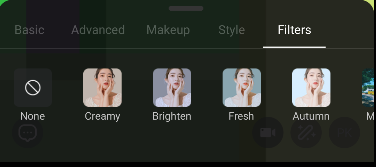
Stickers
The sticker feature requires a relatively large amount of resources. You can choose whether to integrate the sticker feature based on your own needs. If you need to integrate it, you can refer to the following code:
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.addAll(ZegoBeautyPluginConfig.basicTypes());
customTypes.addAll(ZegoBeautyPluginConfig.advancedTypes());
customTypes.addAll(ZegoBeautyPluginConfig.makeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.styleMakeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.filterTypes());
customTypes.addAll(ZegoBeautyPluginConfig.stickerTypes());
config.beautyConfig.effectsTypes = customTypes;
//...
Here's how it would look like:
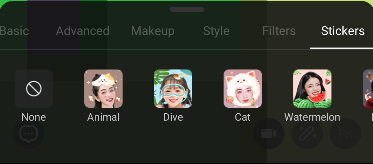
Background segmentation
If you also need the background segmentation feature, refer to the following code to set it up.
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.addAll(ZegoBeautyPluginConfig.basicTypes());
customTypes.addAll(ZegoBeautyPluginConfig.advancedTypes());
customTypes.addAll(ZegoBeautyPluginConfig.makeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.styleMakeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.filterTypes());
customTypes.addAll(ZegoBeautyPluginConfig.stickerTypes());
customTypes.addAll(ZegoBeautyPluginConfig.backgroundTypes());
config.beautyConfig.effectsTypes = customTypes;
//...
For portrait segmentation in background segmentation, you need to provide a background image. You can put the image in the BackgroundImages folder and set it up through the config.
BeautyResources/BackgroundImages/image1.jpg
Configure it through the code like this:
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.addAll(ZegoBeautyPluginConfig.basicTypes());
customTypes.addAll(ZegoBeautyPluginConfig.advancedTypes());
customTypes.addAll(ZegoBeautyPluginConfig.makeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.styleMakeupTypes());
customTypes.addAll(ZegoBeautyPluginConfig.filterTypes());
customTypes.addAll(ZegoBeautyPluginConfig.stickerTypes());
customTypes.addAll(ZegoBeautyPluginConfig.backgroundTypes());
config.beautyConfig.effectsTypes = customTypes;
config.beautyConfig.segmentationBackgroundImageName = "image1.jpg";
//...
Here's how it would look like:
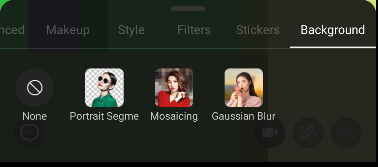
Make further customization
In addition to the aforementioned function customization, you can also pass in a custom effectsTypes
list to determine which beauty effect you want to use.
ZegoUIKitPrebuiltCallConfig config;
// ...
List<ZegoBeautyPluginEffectsType> customTypes = new ArrayList<>();
customTypes.add(ZegoBeautyPluginEffectsType.FILTER_GRAY_FILMLIKE);
customTypes.add(ZegoBeautyPluginEffectsType.FILTER_DREAMY_COZILY);
config.beautyConfig.effectsTypes = customTypes;
//...
Customize UI
The effectsBottomBar that pops up at the bottom supports custom UI. The captions indicate the customization options for each section, and each option will be explained in detail later on.
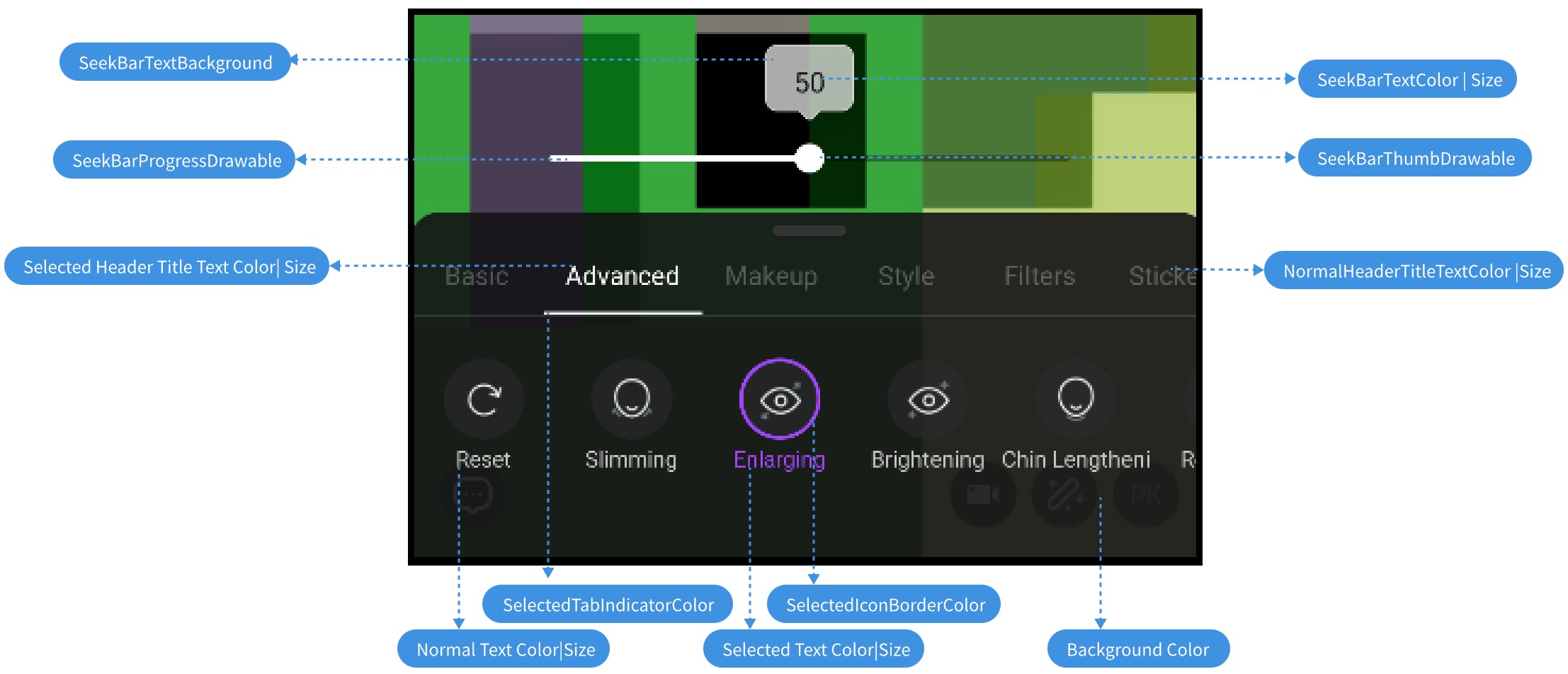
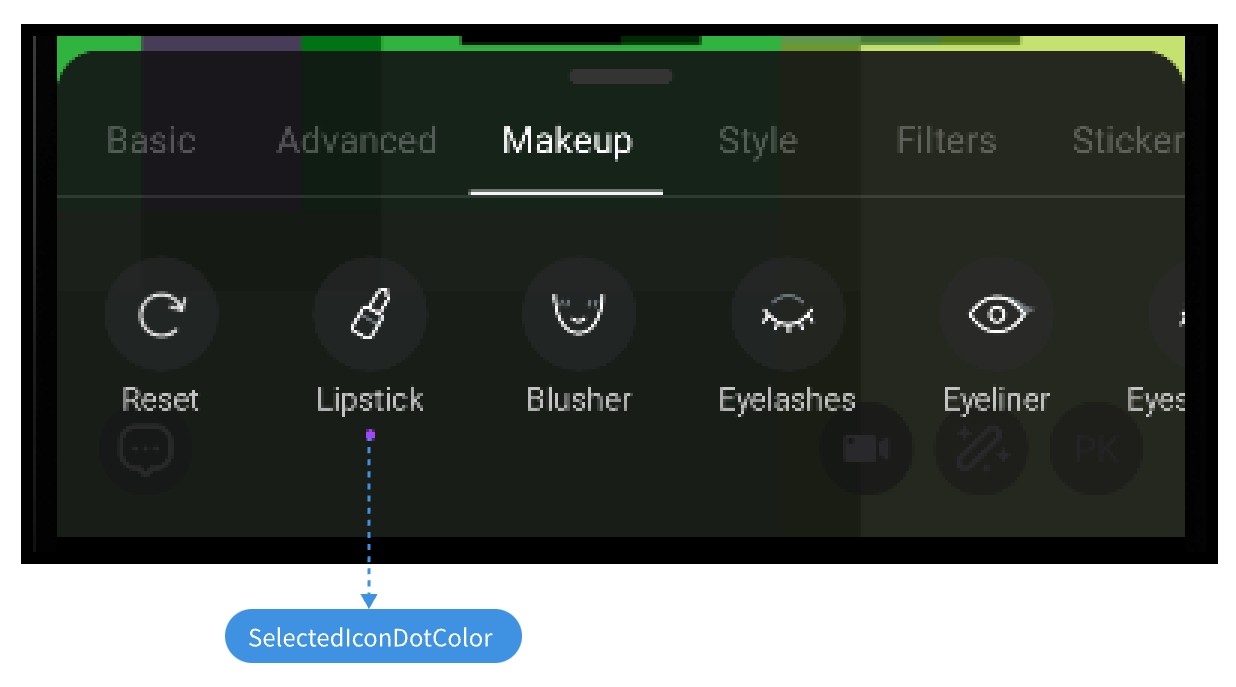
Customize text
To customize the text related to the advanced beauty effects feature, you can use ZegoBeautyPluginInnerText
like the following:
ZegoUIKitPrebuiltCallConfig config;
// ...
config.beautyConfig.innerText.titleFilter = "Filters";
config.beautyConfig.innerText.beautyBasicSmoothing = "Smoothing";
config.beautyConfig.innerText.beautyMakeupLipstick = "Lipstick";
//...
Customize the background color
To customize the background color of the view, refer to the following:
ZegoUIKitPrebuiltCallConfig config;
// ...
config.beautyConfig.uiConfig.backgroundColor = Color.RED;
//...
Customize the text color
To customize the text color, refer to the following:
ZegoUIKitPrebuiltCallConfig config;
// ...
config.beautyConfig.uiConfig.selectedHeaderTitleTextColor = Color.GREEN;
config.beautyConfig.uiConfig.selectedTextColor = Color.CYAN;
//...
Customize the back button icon
To customize the back button icon, refer to the following:
ZegoUIKitPrebuiltCallConfig config;
// ...
config.beautyConfig.uiConfig.backIcon = getDrawable(R.drawable.custom_back_icon);
//...
Save the last used beauty parameters.
To save the last used beauty parameters, please set ZegoUIKitPrebuiltCallConfig.beautyConfig.saveLastBeautyParam
to true.
ZegoUIKitPrebuiltCallConfig config;
// ...
config.beautyConfig.saveLastBeautyParam = true;
If you need to reset the beauty effects, please use ZegoUIKitPrebuiltCallService.resetAllBeautiesToDefault()
.
Resource management
The downloaded resources mentioned above contain resources for all features. However, if you do not need all the features, you can delete some unnecessary resources to reduce the size of the app package.
Resource description
-
Essential resources (required by all features, cannot be deleted)
UntitledBeautyResources/CommonResources.bundle BeautyResources/FaceDetection.model
1 -
Essential resources for stickers (do not delete if you need to use the sticker feature)
UntitledBeautyResources/StickerBaseResources.bundle
1 -
Essential resources for background segmentation (do not delete if you need to use the background segmentation feature)
UntitledBeautyResources/BackgroundSegmentation.model
1 -
Feature resource folder
Each resource in the feature resource folder corresponds to a specific feature. If you need to use a certain feature, you should keep the corresponding resource folder. Otherwise, you may delete it.
UntitledBeautyResources/AdvancedResources
1
Detailed feature resource
Listen to BeautyPlugin Events
You can listen to BeautyPlugin events by config.beautyConfig.beautyEventHandler
,if you have enabled faceDetection in config.beautyConfig
,you can receive face detection data in onFaceDetectionResult
.
Please pay special attention to the onInitResult
callback. If the initialization fails, the beauty function cannot be used. This is usually because the applicationID and appID of the current application are inconsistent with the registered applicationID and appID, or the license has expired.
ZegoUIKitPrebuiltCallConfig config;
// ...
config.beautyConfig.beautyEventHandler = new IBeautyEventHandler() {
@Override
public void onInitResult(int errorCode, String message) {
super.onInitResult(errorCode, message);
Log.d(TAG, "onInitResult() called with: errorCode = [" + errorCode + "], message = [" + message + "]");
}
@Override
public void onError(int errorCode, String message) {
super.onError(errorCode, message);
Log.d(TAG, "onError() called with: errorCode = [" + errorCode + "], message = [" + message + "]");
}
@Override
public void onFaceDetectionResult(ZegoBeautyPluginFaceDetectionResult[] results) {
super.onFaceDetectionResult(results);
Log.d(TAG, "onFaceDetectionResult() called with: results = [" + results + "]");
}
};
//...