Set a hangup confirmation dialog
Call Kit (ZegoUIKitPrebuilt) ends a call by default when the user clicks the End Call button or the Android’s Back button.
If you want to add a confirmation dialog box to double confirm whether the user wants to hang up a call, you can use the hangUpConfirmInfo
config: After configuring the hangUpConfirmInfo parameter, ZegoUIKitPrebuilt will pop up a confirmation dialog box with the default style before ending the call, showing the confirmation info you set.
The effect will be like this:
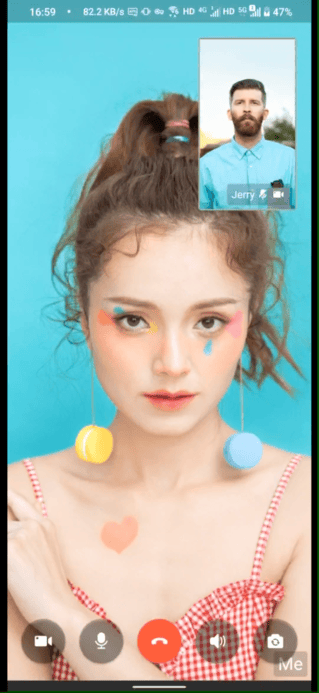
Here is the reference code:
public class CallActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_call);
long appID = YourAppID;
String appSign = YourAppSign;
String userID = "userID";
String userName = "userName";
String callID = "testCallID";
// Modify your custom configurations here.
ZegoUIKitPrebuiltCallConfig config = ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall();
config.hangUpConfirmDialogInfo = new ZegoHangUpConfirmDialogInfo();
config.hangUpConfirmDialogInfo.title= "Hangup confirm";
config.hangUpConfirmDialogInfo.message= "Do you want to hangup?";
config.hangUpConfirmDialogInfo.cancelButtonName= "Cancel";
config.hangUpConfirmDialogInfo.confirmButtonName= "Confirm";
ZegoUIKitPrebuiltCallFragment fragment = ZegoUIKitPrebuiltCallFragment
.newInstance(appID, appSign, callID, userID, userName, config);
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.fragment_container, fragment)
.commitNow();
}
}
public class MainActivity extends AppCompatActivity {
long appID = YourAppID;
String appSign = YourAppSign;
String userID = "userID";
String userName = "userName";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initCallInviteService();
}
public void initCallInviteService() {
ZegoUIKitPrebuiltCallInvitationConfig callInvitationConfig = new ZegoUIKitPrebuiltCallInvitationConfig(),
callInvitationConfig.provider = new ZegoUIKitPrebuiltCallConfigProvider() {
@Override
public ZegoUIKitPrebuiltCallConfig requireConfig(ZegoCallInvitationData invitationData) {
ZegoUIKitPrebuiltCallConfig config;
boolean isVideoCall = invitationData.type == ZegoInvitationType.VIDEO_CALL.getValue();
boolean isGroupCall = invitationData.invitees.size() > 1;
if (isVideoCall && isGroupCall) {
config = ZegoUIKitPrebuiltCallConfig.groupVideoCall();
} else if (!isVideoCall && isGroupCall) {
config = ZegoUIKitPrebuiltCallConfig.groupVoiceCall();
} else if (!isVideoCall) {
config = ZegoUIKitPrebuiltCallConfig.oneOnOneVoiceCall();
} else {
config = ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall();
}
// Modify your custom calling configurations here.
config.hangUpConfirmDialogInfo = new ZegoHangUpConfirmDialogInfo();
config.hangUpConfirmDialogInfo.title= "Hangup confirm";
config.hangUpConfirmDialogInfo.message= "Do you want to hangup?";
config.hangUpConfirmDialogInfo.cancelButtonName= "Cancel";
config.hangUpConfirmDialogInfo.confirmButtonName= "Confirm";
return config;
}
);
ZegoUIKitPrebuiltCallService.init(getApplication(), appID, appSign, userID, userName,
callInvitationConfig);
}
@Override
protected void onDestroy() {
super.onDestroy();
ZegoUIKitPrebuiltCallService.logout();
}
}
If you want to listen for hang-up events, for example, to save the call recording when ending the call, ZegoUIKitPrebuiltCallConfig provides a leaveCallListener
, and the leaveCallListener
will be triggered when the call ends. And sure, you can also implement custom business logic in the LeaveCallListener
.