Customize the menu bar button list
Customize the menu bar button list
Call Kit (ZegoUIKitPrebuiltCall) allows you to configure the buttons of the menu bar. To remove the default buttons or add custom ones to the bottom menu bar, you can configure the bottomMenuBarConfig
:
(Similarly, to configure top menu bar buttons or add custom buttons to the top menu bar, use the topMenuBarConfig
.)
buttons
: Built-in buttons placed in the menu bar. By default, all buttons are displayed. If you need to hide some buttons, use this to configure them.maxCount
: Maximum number of buttons that can be displayed by the menu bar. Value range [1 - 5], the default value is 5.extendButtons
: Use this configuration to add your own custom buttons to menu bar.
If the total number of built-in buttons and custom buttons does not exceed 5, all buttons will be displayed. Otherwise, other buttons that cannot be displayed will be hidden in the three dots (⋮) button. Clicking this button will pop up the bottom sheet to display the remaining buttons.
The effect will be like this:
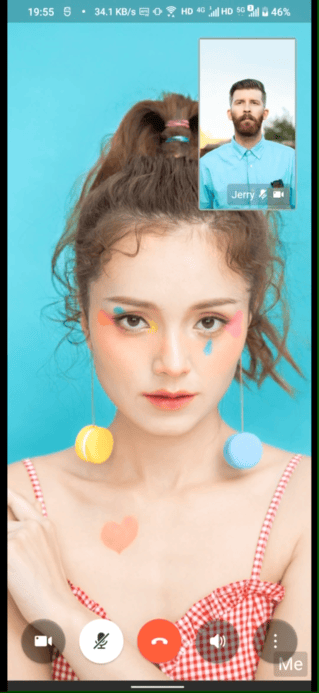
Here is the reference code:
public class CallActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_call);
long appID = YourAppID;
String appSign = YourAppSign;
String userID = "userID";
String userName = "userName";
String callID = "testCallID";
// Modify your custom configurations here.
ZegoUIKitPrebuiltCallConfig config = ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall();
config.bottomMenuBarConfig.buttons = Arrays.asList(ZegoMenuBarButtonName.TOGGLE_CAMERA_BUTTON,ZegoMenuBarButtonName.SWITCH_CAMERA_BUTTON, ZegoMenuBarButtonName.HANG_UP_BUTTON,ZegoMenuBarButtonName.TOGGLE_MICROPHONE_BUTTON, ZegoMenuBarButtonName.SWITCH_AUDIO_OUTPUT_BUTTON);
ZegoUIKitPrebuiltCallFragment fragment = ZegoUIKitPrebuiltCallFragment
.newInstance(appID, appSign, callID, userID, userName, config);
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.fragment_container, fragment)
.commitNow();
}
}
public class MainActivity extends AppCompatActivity {
long appID = YourAppID;
String appSign = YourAppSign;
String userID = "userID";
String userName = "userName";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initCallInviteService();
}
public void initCallInviteService() {
ZegoUIKitPrebuiltCallInvitationConfig callInvitationConfig = new ZegoUIKitPrebuiltCallInvitationConfig();
callInvitationConfig.provider = new ZegoUIKitPrebuiltCallConfigProvider() {
@Override
public ZegoUIKitPrebuiltCallConfig requireConfig(ZegoCallInvitationData invitationData) {
ZegoUIKitPrebuiltCallConfig config = ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall();
// Modify your custom calling configurations here.
config.bottomMenuBarConfig.buttons = Arrays.asList(ZegoMenuBarButtonName.TOGGLE_CAMERA_BUTTON,ZegoMenuBarButtonName.SWITCH_CAMERA_BUTTON, ZegoMenuBarButtonName.HANG_UP_BUTTON,ZegoMenuBarButtonName.TOGGLE_MICROPHONE_BUTTON, ZegoMenuBarButtonName.SWITCH_AUDIO_OUTPUT_BUTTON);
return config;
}
};
ZegoUIKitPrebuiltCallService.init(getApplication(), appID, appSign, userID, userName, callInvitationConfig);
}
@Override
protected void onDestroy() {
super.onDestroy();
ZegoUIKitPrebuiltCallService.logout();
}
}
Customize the hidden behavior of the menu bar
The Call Kit (ZegoUIkitPrebuiltCall) supports automatic or manual hiding of the menu bar. You can control this by using the following two configurations in the ZegoBottomMenuBarConfig
:
hideByClick
: Whether the menu bar can be hidden by clicking on the unresponsive area, enabled by default.hideAutomatically
: Whether the menu bar is automatically hidden after 5 seconds of user inactivity, enabled by default.
Add chat button to the menu bar
You can modify config.bottomMenuBarConfig.buttons
to add a chat button to the menubar.
Here is the reference code:
config.bottomMenuBarConfig.buttons = Arrays.asList(ZegoMenuBarButtonName.CHAT_BUTTON, ZegoMenuBarButtonName.TOGGLE_CAMERA_BUTTON,ZegoMenuBarButtonName.SWITCH_CAMERA_BUTTON, ZegoMenuBarButtonName.HANG_UP_BUTTON,ZegoMenuBarButtonName.TOGGLE_MICROPHONE_BUTTON, ZegoMenuBarButtonName.SWITCH_AUDIO_OUTPUT_BUTTON);
Customize the enum button images
You can modify config.bottomMenuBarConfig.buttonConfig
to change the enum button images.
config.bottomMenuBarConfig.buttonConfig.toggleCameraOnImage = drawableXXX;