Get message history
Function overview
This document describes how to query the message history and specific messages of one-to-one, group, and in-room chats with the ZIM SDK.
This feature allows you to get historical messages of types other than command messages and pop-up messages.
Get the full message history
After logging in to ZIM SDK, users can use the queryHistoryMessageByConversationID method to retrieve the message history of one-to-one chats
, group chats
, and rooms chats
by providing the parameters conversationID and config.
Taking the example of client A retrieving the conversation history with client B in a one-on-one chat:
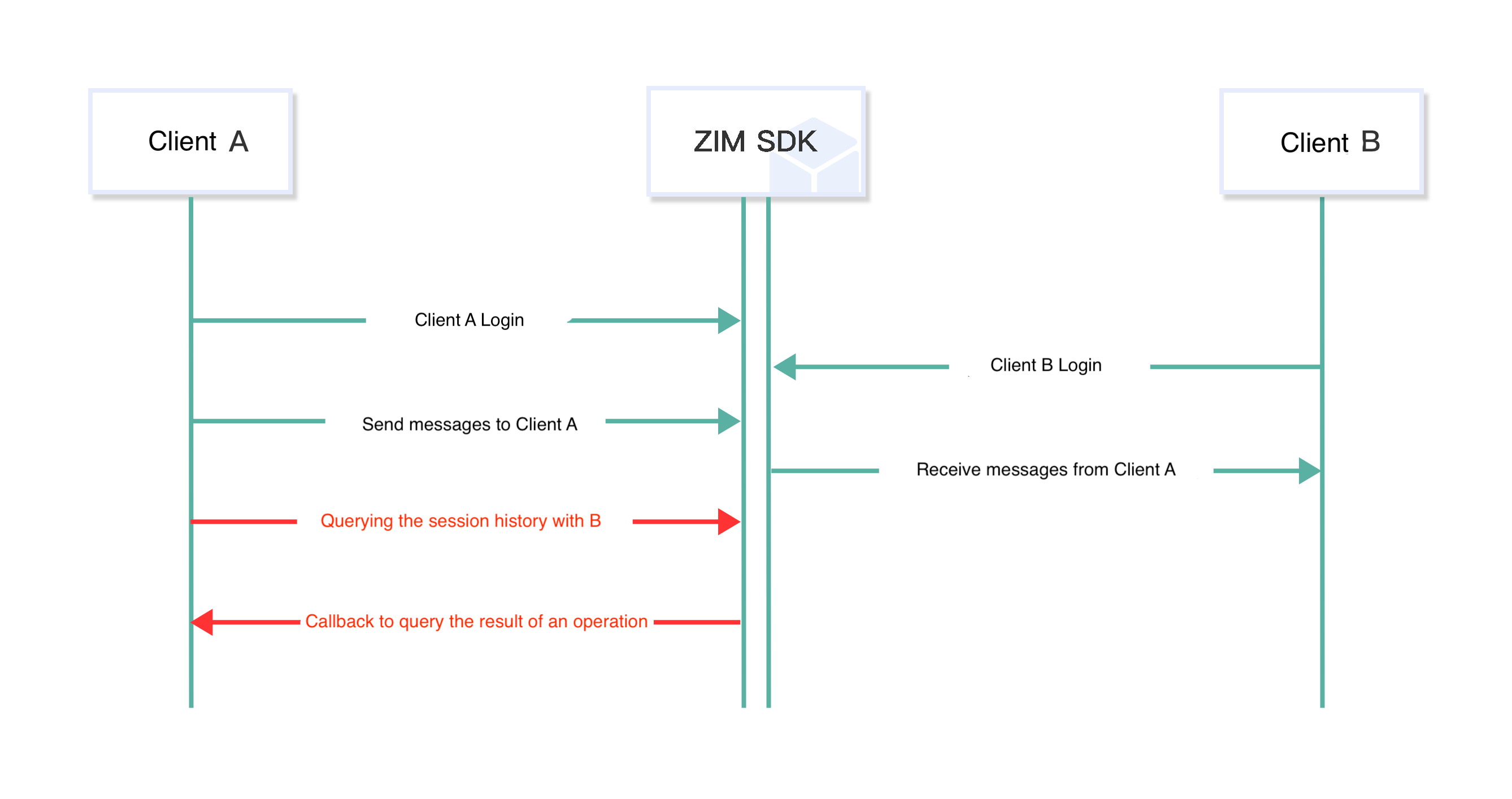
- Client A and B log in to the ZIM SDK and send/receive one-on-one chat messages to each other.
- When client A needs to retrieve the conversation records with B:
- Client A first logs in to the ZIM SDK.
- Client A calls the queryHistoryMessageByConversationID interface and passes the conversationID and config parameters to start retrieving.
- The retrieved results will be notified to client A through the ZIMMessageQueriedCallback callback interface.
// Get the chat history of a one-on-one chat
NSString *conversationID = @"xxxx";
// Retrieve messages from the back to the front, 20 messages at a time
ZIMMessageQueryConfig *config = [[ZIMMessageQueryConfig alloc] init];
config.count = 20;
config.reverse = true;
// nextMessage is null for the first retrieval
config.nextMessage = nil
[zim queryHistoryMessageByConversationID:conversationID conversationType:conversationType config:config callback:^(NSArray<ZIMMessage *> * _Nonnull messageList, ZIMError * _Nonnull errorInfo) {
// Developers can listen to this callback to get the message list.
// When scrolling down to the topmost message on the screen, retrieve earlier messages
// Save the frontmost message as the anchor for the messages
if (fetchMore) {
// nextMessage is the first message in the current retrieved message list for subsequent pagination retrieval
config.nextMessage = messageList[0];
// Recursively call the retrieval function
}
}];
Get specific messages
ZIM supports querying specific messages in a one-to-one or group conversation based on messageSeq
(the sequence number of the message in the conversation) list (up to a maximum of 10) by calling queryMessagesByMessageSeqs.
This interface is used when you only know the messageSeq
of a message and do not know the complete structure of the message. For example, if a message in a conversation replies to a historical message, members of the conversation can use the repliedInfo.messageSeq
of the reply to obtain the messageSeq
of the historical message. At this time, you can call this interface to obtain the complete structure of the historical message.
NSArray<NSNumber *> messageSeqs = @[]; // The maximum length is 10
NSString *conversationID = @"YOUR_CONVERSATION_ID";
ZIMConversationType conversationType = ZIMConversationTypePeer; // Conversation type: one-to-one: ZIMConversationTypePeer, group: ZIMConversationTypeGroup
[[ZIM getInstance] queryMessagesByMessageSeqs:messageSeqs conversationID:conversationID conversationType:conversationType callback:^(NSString * _Nonnull conversationID, ZIMConversationType conversationType, NSArray<ZIMMessage *> * _Nonnull messageList, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == ZIMErrorCodeSuccess) {
// Query successful
} else {
// Query failed
}
}];