Respond to messages with emoticons
Overview
An emoticon response to a message indicates how a user responds to a message. The emoticon response feature is usually used to respond to a message in a private chat or group chat by adding an emoticon response to or removing an emoticon response from the message. Further, the emoticon response feature can be used in scenarios such as group voting and group voting result confirmation.
The preceding figure shows merely a UI example of an emoticon response. ZEGO Instant Messaging (ZIM) SDK does not provide aesthetics resources for emoticon responses. You must add aesthetics resources as needed.
Procedure
ZIM SDK allows you to respond to a specific message in a private chat or group chat. The procedure is described in the following figure, in which a client B responds to a message from a client A by adding an emoticon response to or removing an emoticon response from the message.
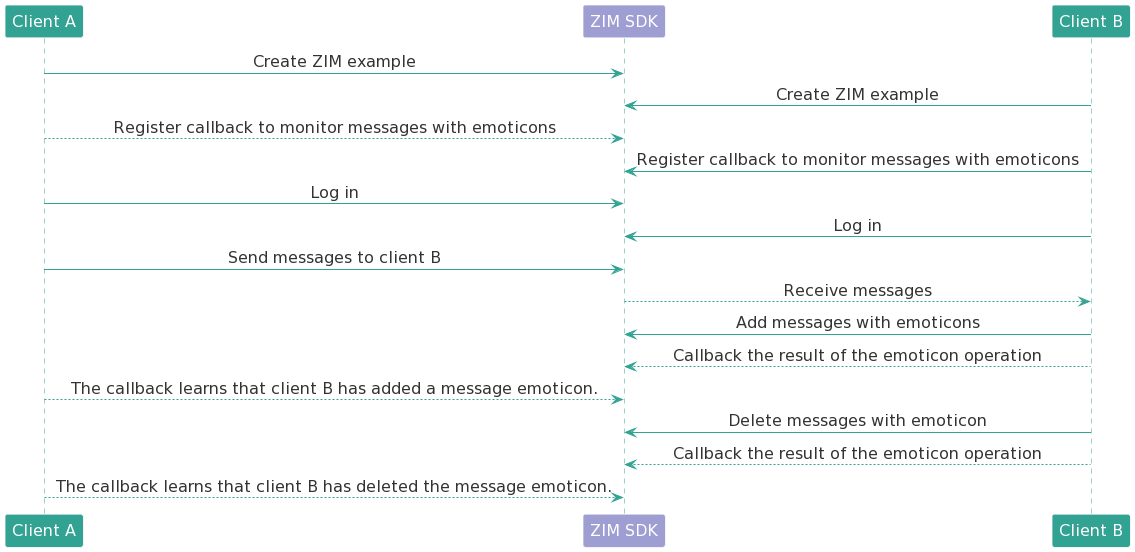
- The client A and the client B each create a ZIM instance and register the messageReactionsChanged callback of the ZIMEventHandler class to listen to emoticon response changes.
- The client A and the client B log in to ZIM SDK.
- The client A sends a private-chat message to the client B, and the client B adds an emoticon response to the message.
- The client B calls the addMessageReaction operation and set the
reactionType
and message
parameters to specify the message to which an emoticon response is added.
- The client B obtains the addition result by invoking the ZIMMessageReactionAddedCallback callback.
- The client A receives a notification about an emoticon response change by invoking the messageReactionsChanged callback.
- The client B removes the preceding emoticon response.
- The client B calls the deleteMessageReaction operation and set the
reactionType
and message
parameters to specify the message whose emoticon response is to be removed.
- The client B obtains the removal result by invoking the ZIMMessageReactionDeletedCallback callback.
- The client A receives a notification about an emoticon response change by invoking the messageReactionsChanged callback.
1. Listen to an emoticon response change
After a user creates a ZIM instance, the user must register the messageReactionsChanged callback of the ZIMEventHandler class to listen to emoticon response changes. This way, when other users add emoticon responses to or remove emoticon responses from a specific message, the user that registers the onMessageReactionsChanged
callback can obtain relevant emoticon response information, such as the types of the emoticon responses and the number of users who add emoticon responses or remove emoticon responses. In general, this callback can return information about a maximum of five users. For more user information details, see the "Query details of emoticon responses" section of the Respond to messages with emoticons topic.
// Received message with emoticons callback.
public void onMessageReactionsChanged(ZIM zim, ArrayList<ZIMMessageReaction> reactions) {
}
1
// Received message with emoticons callback.
- (void)zim:(ZIM *)zim messageReactionsChanged:(NSArray<ZIMMessageReaction *> *)reactions;
1
// Received message with emoticons callback.
- (void)zim:(ZIM *)zim messageReactionsChanged:(NSArray<ZIMMessageReaction *> *)reactions;
1
// Received message with emoticons callback.
virtual void onMessageReactionsChanged(ZIM * /*zim*/,
const std::vector<ZIMMessageReaction> & /*reactions*/) {}
1
// Receive message reaction callback
ZIM.GetInstance().onMessageReactionsChanged += (
ZIM zim,
List<ZIMMessageReaction> reactionList) =>
{
};
1
// The callback for listening to emoticon response changes
ZIMEventHandler.onMessageReactionsChanged = (ZIM zim, List<ZIMMessageReaction> infos){
};
1
// Received message with emoticons callback.
zim.on('messageReactionsChanged', function (zim, { reactions }) {
console.log(reactions);
});
1
2. Add an emoticon response
You can call the addMessageReaction operation to add an emoticon response to any message sent in a private chat or a group chat. You can obtain the addition result by invoking the ZIMMessageReactionAddedCallback callback. In general, this Result
can return information about a maximum of five users. For more user information details, see the "Query details of emoticon responses" section of the Respond to messages with emoticons topic.
-
If you repeatedly call this operation to add emoticon responses to the same message, an error may occur.
-
By default, a maximum of 100 types of emoticon responses can be given to a message. To expand the upper limit, contact ZEGO technical support.
// Add an emoticon response.
String reactionType = "key";
zim.addMessageReaction(reactionType, message, new ZIMMessageReactionAddedCallback() {
@Override
public void onMessageReactionAdded(ZIMMessageReaction reaction, ZIMError error) {
if (error.code == ZIMErrorCode.SUCCESS){
// The operation is successful.
}else {
// The operation fails.
}
}
});
1
// Add an emoticon response.
NSString* reactionType = @"key";
[zim addMessageReaction:reactionType message:message callback:^(ZIMMessageReaction * _Nonnull reaction, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == 0){
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Add an emoticon response.
NSString* reactionType = @"key";
[zim addMessageReaction:reactionType message:message callback:^(ZIMMessageReaction * _Nonnull reaction, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == 0){
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Add an emoticon response.
auto reactionType = "key";
zim->addMessageReaction(reactionType,messagePtr, [=](const ZIMMessageReaction &reaction, const ZIMError &errorInfo) {
if (errorInfo.code == 0) {
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Add a message reaction
string reactionType = "key";
ZIM.GetInstance().AddMessageReaction(reactionType, message, (ZIMMessageReaction reaction, ZIMError errorInfo) =>
{
if (errorInfo.code == ZIMErrorCode.Success){
// Operation succeeded
}else {
// Operation failed
}
});
1
// Add an emoticon response.
ZIM.getInstance()!.addMessageReaction('key', ZIMTextMessage(message: 'message')).then((value) {
// The emoticon response is added.
}).catchError((onError){
// The emoticon response fails to be added.
});
1
const reactionType = "key";
zim.deleteMessageReaction(reactionType, message)
.then(function (reaction) {
// The operation is successful, and the status list of the message is updated on the UI.
})
.catch(function (err) {
// The operation fails.
});
1
3. Remove an emoticon response
After you add an emoticon response to a message, you can call the deleteMessageReaction operation to remove the emoticon response. You can obtain the removal result by invoking the ZIMMessageReactionDeletedCallback callback. In general, this Callback
can return information about a maximum of five users. For more user information details, see the "Query details of emoticon responses" section of the Respond to messages with emoticons topic.
You can call this operation to remove an emoticon response that you added.
// Remove an emoticon response.
String reactionType = "key";
zim.deleteMessageReaction(reactionType, message, new ZIMMessageReactionDeletedCallback() {
@Override
public void onMessageReactionDeleted(ZIMMessageReaction reaction, ZIMError error) {
if (error.code == ZIMErrorCode.SUCCESS){
// The operation is successful.
}else {
// The operation fails.
}
}
});
1
// Remove an emoticon response.
NSString* reactionType = @"key";
[zim deleteMessageReaction:reactionType message:message callback:^(ZIMMessageReaction * _Nonnull reaction, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == 0){
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Remove an emoticon response.
NSString* reactionType = @"key";
[zim deleteMessageReaction:reactionType message:message callback:^(ZIMMessageReaction * _Nonnull reaction, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == 0){
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Remove an emoticon response.
auto reactionType = "key";
zim->deleteMessageReaction(reactionType,messagePtr, [=](const ZIMMessageReaction &reaction, const ZIMError &errorInfo) {
if (errorInfo.code == 0) {
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Delete a message reaction
string reactionType = "key";
ZIM.GetInstance().DeleteMessageReaction(reactionType, message, (ZIMMessageReaction reaction, ZIMError errorInfo) =>
{
if (errorInfo.code == ZIMErrorCode.Success){
// Operation succeeded
}else {
// Operation failed
}
});
1
// Remove an emoticon response.
ZIM.getInstance()!.deleteMessageReaction('Key', ZIMTextMessage(message: 'message')).then((value) {
// The emoticon response is removed.
}).catchError((onError){
// The emoticon response fails to be removed.
});
1
const reactionType = "key";
zim.deleteMessageReaction(reactionType, message)
.then(function (reaction) {
//The operation is successful, and the status list of the message is updated on the UI.
})
.catch(function (err) {
//The operation fails.
});
1
What's more
Query details of emoticon responses
You can query only brief information about the users that listen to, add, and remove emoticon responses. By default, you can query information about a maximum of five users. To expand the upper limit, contact ZEGO technical support. To query specific users who give a specific type of emoticon responses to a specific message, call the queryMessageReactionUserListByMessage operation. You can obtain the operation result by invoking the ZIMMessageReactionUserListQueriedCallback callback.
// Query details of emoticon responses.
ZIMMessageReactionUserQueryConfig config =new ZIMMessageReactionUserQueryConfig();
config.reactionType = "key";
config.count = 10;
config.nextFlag = 0;
zim.queryMessageReactionUserList(message, config,new ZIMMessageReactionUserListQueriedCallback() {
@Override
public void onMessageReactionUserListQueried(ZIMMessage message, ArrayList<ZIMMessageReactionUserInfo> userList,
String reactionType, long nextFlag, int totalCount,
ZIMError error) {
if (error.code == ZIMErrorCode.SUCCESS){
// The operation is successful.
}else {
// The operation fails.
}
}
});
1
// Query details of emoticon responses.
ZIMMessageReactionUserQueryConfig *config = [[ZIMMessageReactionUserQueryConfig alloc] init];
config.nextFlag = 0;
config.reactionType = @"key";
config.count = 20;
[zim queryMessageReactionUserListByMessage:message config:config callback:^(ZIMMessage * _Nonnull message, NSArray<ZIMMessageReactionUserInfo *> * _Nonnull userList, NSString * _Nonnull reactionType, long long nextFlag, int totalCount, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == 0){
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Query details of emoticon responses.
ZIMMessageReactionUserQueryConfig *config = [[ZIMMessageReactionUserQueryConfig alloc] init];
config.nextFlag = 0;
config.reactionType = @"key";
config.count = 20;
[zim queryMessageReactionUserListByMessage:message config:config callback:^(ZIMMessage * _Nonnull message, NSArray<ZIMMessageReactionUserInfo *> * _Nonnull userList, NSString * _Nonnull reactionType, long long nextFlag, int totalCount, ZIMError * _Nonnull errorInfo) {
if(errorInfo.code == 0){
// The operation is successful.
}
else{
// The operation fails.
}
}];
1
// Query message reaction user details.
ZIMMessageReactionUserQueryConfig config;
config.nextFlag = 0;
// If count exceeds 100, an error will be returned
config.count = 10;
config.reactionType = "key";
zim->queryMessageReactionUserList(messagePtr, config, [=](const std::shared_ptr<ZIMMessage> &message,
const std::vector<ZIMMessageReactionUserInfo> &userList, const std::string &reactionType,
const long long nextFlag, const unsigned int totalCount, const ZIMError &errorInfo) {
if (errorInfo.code == 0) {
// Operation successful
}
else {
// Operation failed
}
});
1
// Query reaction details
ZIMMessageReactionUserQueryConfig config = new ZIMMessageReactionUserQueryConfig();
config.reactionType = "key";
// An error will be returned if count exceeds 100
config.count = 10;
config.nextFlag = 0;
ZIM.GetInstance().QueryConversationList(config, (List<ZIMConversation> conversationList, ZIMError errorInfo) =>
{
if (errorInfo.code == ZIMErrorCode.SUCCESS){
// Operation succeeded
}else {
// Operation failed
}
});
1
// Query details of emoticon responses.
ZIMMessageReactionUsersQueryConfig config = ZIMMessageReactionUsersQueryConfig(reactionType: "key");
config.count = 20;
config.nextFlag = 0;
ZIM.getInstance()!.queryMessageReactionUserList(ZIMTextMessage(message: 'message'), config).then((value) {
// The operation is successful. The details of the emoticon responses can be rendered on the UI.
}).catchError((onError){
// The operation fails.
});
1
const config = {
nextFlag: 0,
reactionType: "key",
count: 20,
};
zim.queryMessageReactionUserList(message, config)
.then(function (res) {
// The operation is successful, and the status list of the message is updated on the UI.
})
.catch(function (err) {
// The operation fails.
});
1