Update a push notification
Overview
When sending a new notification, you can also retract the previous push notification. This feature is commonly used to cancel a call invitation, retract a message, and other scenarios.
How it works
ZPNs supports including the "mutable-content":1
field when sending APNs push notifications. This allows your app to intercept the push message, modify its content, and then display it. For more details, please refer to Description of Mutable-content in the Official Documentation of Apple Developer.
Configure resourceID
Contact ZEGO technical support to configure the resourceID that carries "mutable-content":1
.
Sender
When sending offline push notifications with ZIMPushConfig, please fill in the above resourceID
.
Taking sending a call invitation as an example:
ZIMCallInviteConfig *config = [[ZIMCallInviteConfig alloc] init];
config.timeout = 60;
config.mode = ZIMCallInvitationModeGeneral;
ZIMPushConfig *pushConfig = [[ZIMPushConfig alloc] init];
pushConfig.resourcesID = KeyCenter.resourceID;
pushConfig.title = [ZGZIMManager shared].myUserID;
pushConfig.content = @"Invite you to voice call";
// payload carries a custom identifier
pushConfig.payload = @"{\"customId\":\"1\"}";
config.pushConfig = pushConfig;
// Send a call invitation
[[ZGZIMManager shared] callInviteWithInvitees:self.selectMemberList config:config callback:^(NSString * _Nonnull callID, ZIMCallInvitationSentInfo * _Nonnull info, ZIMError * _Nonnull errorInfo) {}];
Receiver
Open Xcode, select the target under TARGETS, and go to Signing & Capabilities > Capabilities. Enable Push Notification (for offline push notifications).
-
Click "File > New > Target..."
-
In the pop-up window, select "iOS > Notification Service Extension".
-
Enter the Product Name and other information for the extension.
After creating the extension, a "xxxExtension" folder (xxx is the Product Name entered when adding the extension) will be generated in the project. You will use the NotificationService class file in it.
Select the extension target under TARGETS, and then go to "Signing & Capabilities > Capabilities > Push Notification" to enable offline push notifications.
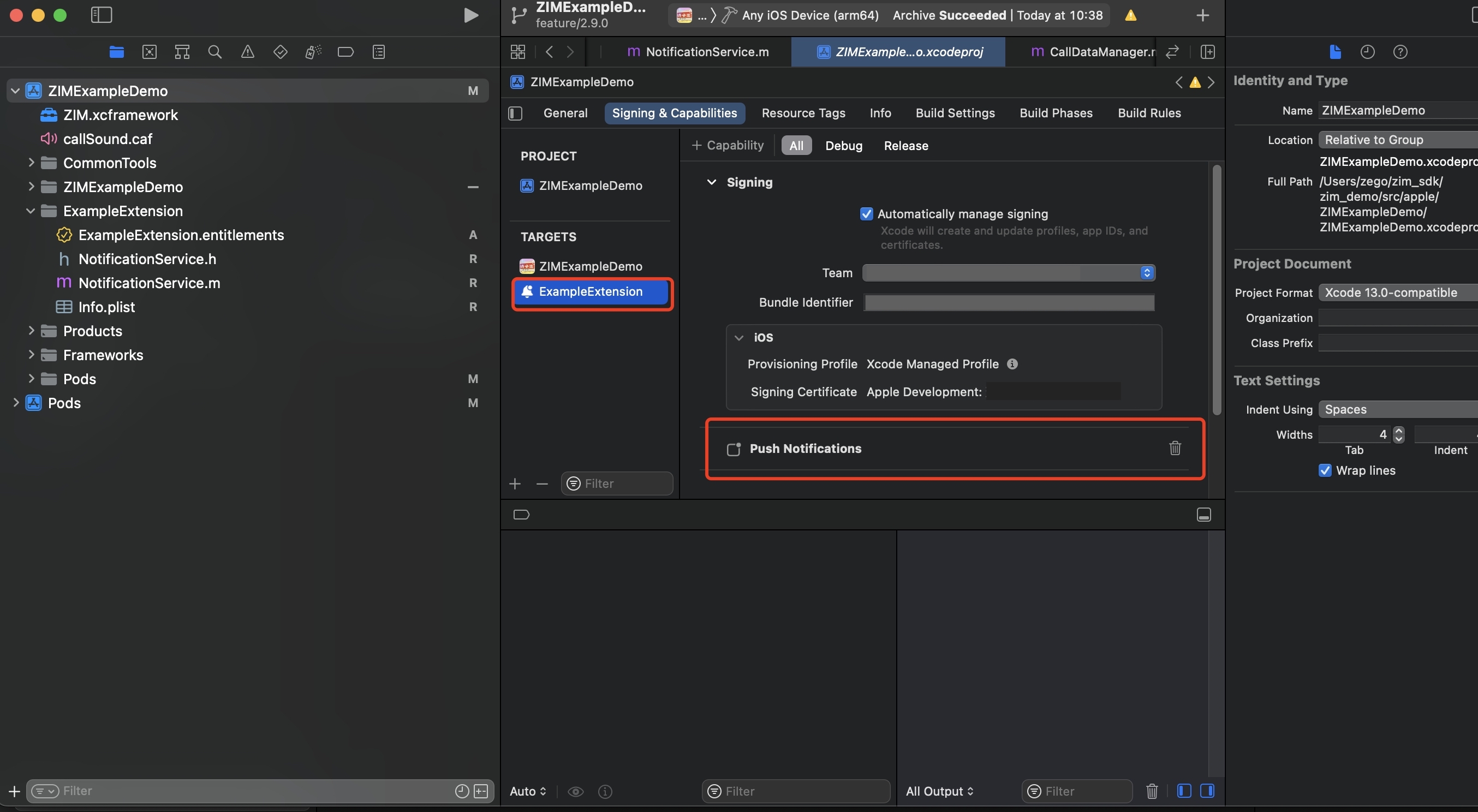
If the iOS version of the used device is lower than the version specified here, the extension will not take effect on that device.
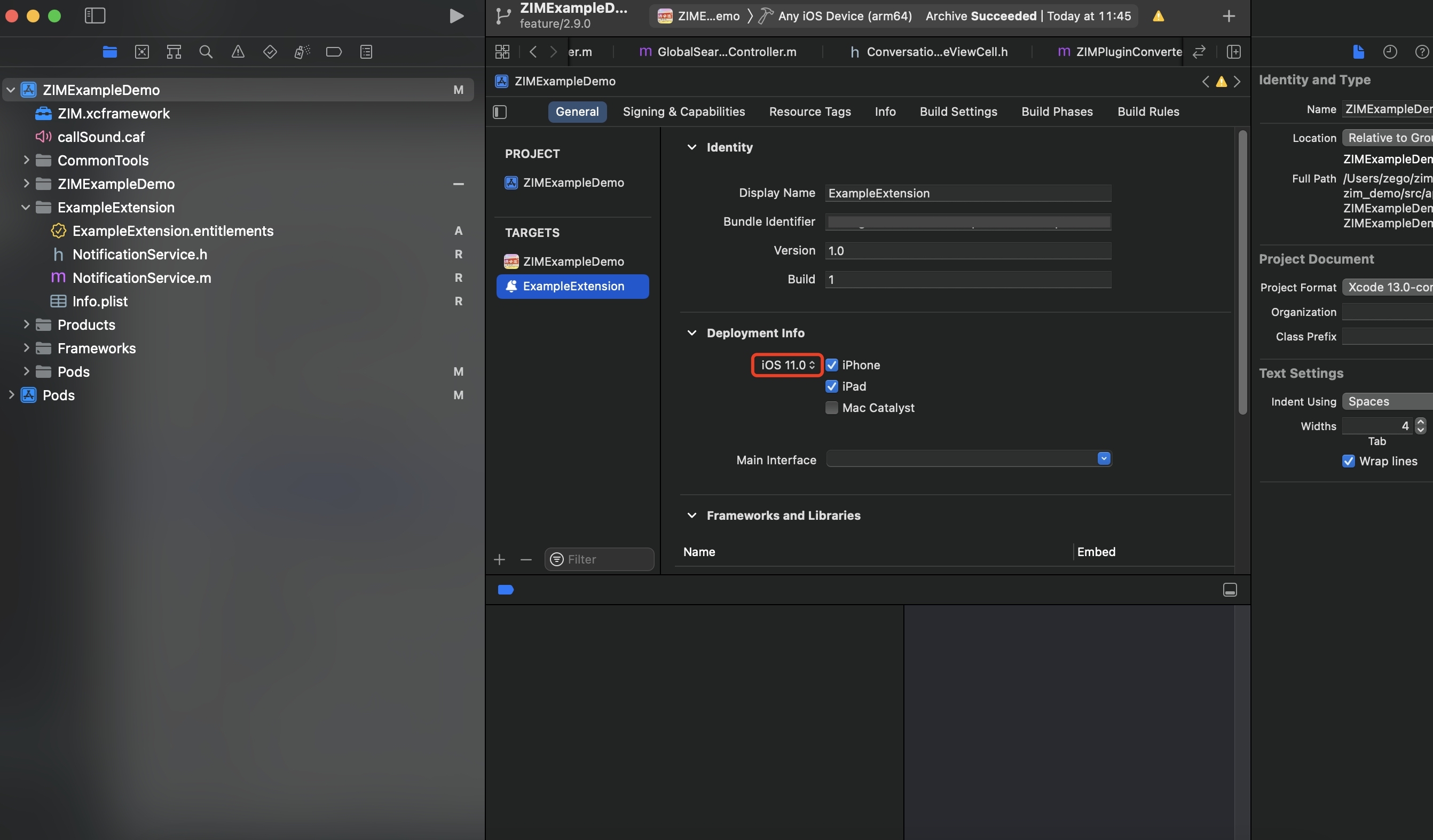
In the NotificationService.m file in the "xxxExtension" folder (xxx is the Product Name entered when adding the extension), write the business logic to update the notification. The example code is as follows:
// NotificationService.m
// NotificationService
#import "NotificationService.h"
#import <Intents/Intents.h>
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;
@end
@implementation NotificationService
// When push interception is enabled, this method will be triggered when a push notification with "mutable-content":1 is received.
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler {
self.contentHandler = contentHandler;
self.bestAttemptContent = [request.content mutableCopy];
// Title
NSString *title = self.bestAttemptContent.title;
// Subtitle
NSString *subtitle = self.bestAttemptContent.subtitle;
// Body
NSString *body = self.bestAttemptContent.body;
// Get the payload string carried by the push message
NSString *payload = [self.bestAttemptContent.userInfo objectForKey:@"payload"];
if(payload == nil){
self.contentHandler(self.bestAttemptContent);
return;
}
// Parse the JSON string and convert it to NSDictionary
NSData *jsonData = [payload dataUsingEncoding:NSUTF8StringEncoding];
NSError *error = nil;
NSDictionary *payload_json_map = [NSJSONSerialization JSONObjectWithData:jsonData options:kNilOptions error:&error];
if (error) {
self.contentHandler(self.bestAttemptContent);
return;
}
// Extract the unique identifier carried by the payload
NSString *customId = [payload_json_map objectForKey:@"customId"];
if(customId != nil){
[UNUserNotificationCenter.currentNotificationCenter getDeliveredNotificationsWithCompletionHandler:^(NSArray<UNNotification *> * _Nonnull notifications) {
NSString *target_id = nil;
// Traverse the existing notifications, find the notification with the same unique identifier, and delete it
for (int index = 0; index < notifications.count; index ++) {
if([[notifications[index].request.content.userInfo objectForKey:@"customId"] isEqual: customId]){
target_id = notifications[index].request.identifier;
break;
}
}
if(target_id != nil){
[UNUserNotificationCenter.currentNotificationCenter removeDeliveredNotificationsWithIdentifiers:@[target_id]];
}
contentHandler(self.bestAttemptContent);
}];
}else{
contentHandler(self.bestAttemptContent);
}
}
@end