Customize the menu bar
Customize menu bar buttons
The Live Streaming Kit (ZegoUIKitPrebuiltLiveStreaming) allows you to configure the buttons of the menu bar.
You can remove the default buttons or add custom ones. If necessary, you can configure the bottomMenuBar
:
hostButtons
: Use this to set the prebuilt-in buttons for a host to use.coHostButtons
: Use this to set the prebuilt-in buttons for a co-host to use.audienceButtons
: Use this to set the prebuilt-in buttons for an audience to use.hostExtendButtons
: Use this to set the custom buttons for a host to use.coHostExtendButtons
: Use this to set the custom buttons for a co-host to use.audienceExtendButtons
: Use this to set the custom buttons for an audience to use.maxCount
: Maximum number of buttons that can be displayed by menu bar. Value range [1 - 5], the default value is 5.showInRoomMessageButton
: Whether to display the message button, displayed by default.
If the total number of built-in buttons and custom buttons does not exceed 5, all buttons will be displayed.
Otherwise, other buttons that cannot be displayed will be hidden in the three dots (⋮) button. Clicking this button will pop up the bottom sheet to display the remaining buttons. The effect is as follows:
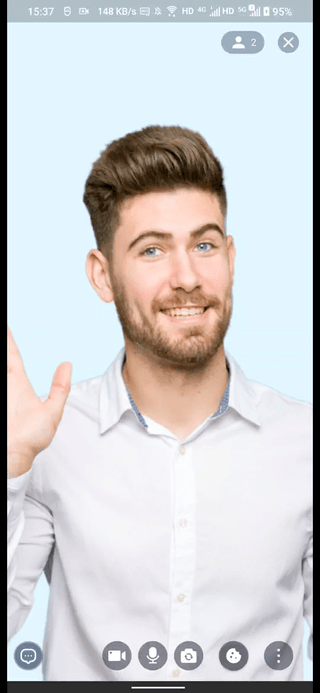
Here is the reference code:
Untitled
class LivePage extends StatelessWidget {
const LivePage({Key? key, required this.liveID, this.isHost = false})
: super(key: key);
final String liveID;
final bool isHost;
@override
Widget build(BuildContext context) {
List<IconData> customIcons = [
Icons.cookie,
Icons.phone,
Icons.speaker,
Icons.air,
Icons.blender,
Icons.file_copy,
Icons.place,
Icons.phone_android,
Icons.phone_iphone,
];
return ZegoUIKitPrebuiltLiveStreaming(
appID: YourSecret.appID,
appSign: YourSecret.appSign,
userID: userID,
userName: 'user_$userID',
liveID: liveID,
// Modify your custom configurations here.
config: isHost
? ZegoUIKitPrebuiltLiveStreamingConfig.host()
: ZegoUIKitPrebuiltLiveStreamingConfig.audience()
..bottomMenuBar = ZegoLiveStreamingBottomMenuBarConfig(
maxCount: 5,
hostButtons: [
ZegoLiveStreamingMenuBarButtonName.switchCameraButton,
ZegoLiveStreamingMenuBarButtonName.toggleCameraButton,
ZegoLiveStreamingMenuBarButtonName.toggleMicrophoneButton,
],
hostExtendButtons: customIcons
.map(
(customIcon) => ZegoLiveStreamingMenuBarExtendButton(
child: ElevatedButton(
style: ElevatedButton.styleFrom(
fixedSize: const Size(40, 40),
shape: const CircleBorder(),
primary: const Color(0xff2C2F3E).withOpacity(0.6),
),
onPressed: () {},
child: Icon(customIcon),
),
),
)
.toList(),
coHostButtons: [
ZegoLiveStreamingMenuBarButtonName.switchCameraButton,
ZegoLiveStreamingMenuBarButtonName.toggleCameraButton,
ZegoLiveStreamingMenuBarButtonName.toggleMicrophoneButton,
ZegoLiveStreamingMenuBarButtonName.coHostControlButton,
],
),
);
}
}
1
Customize styles
In addition, we also support customizing some styles. Currently, we support the following:
padding
: padding for the top/bottom menu bar.margin
: margin for the top/bottom menu bar.backgroundColor
: background color for the top/bottom menu bar.height
: height for the top/bottom menu bar, height = border + padding + content.
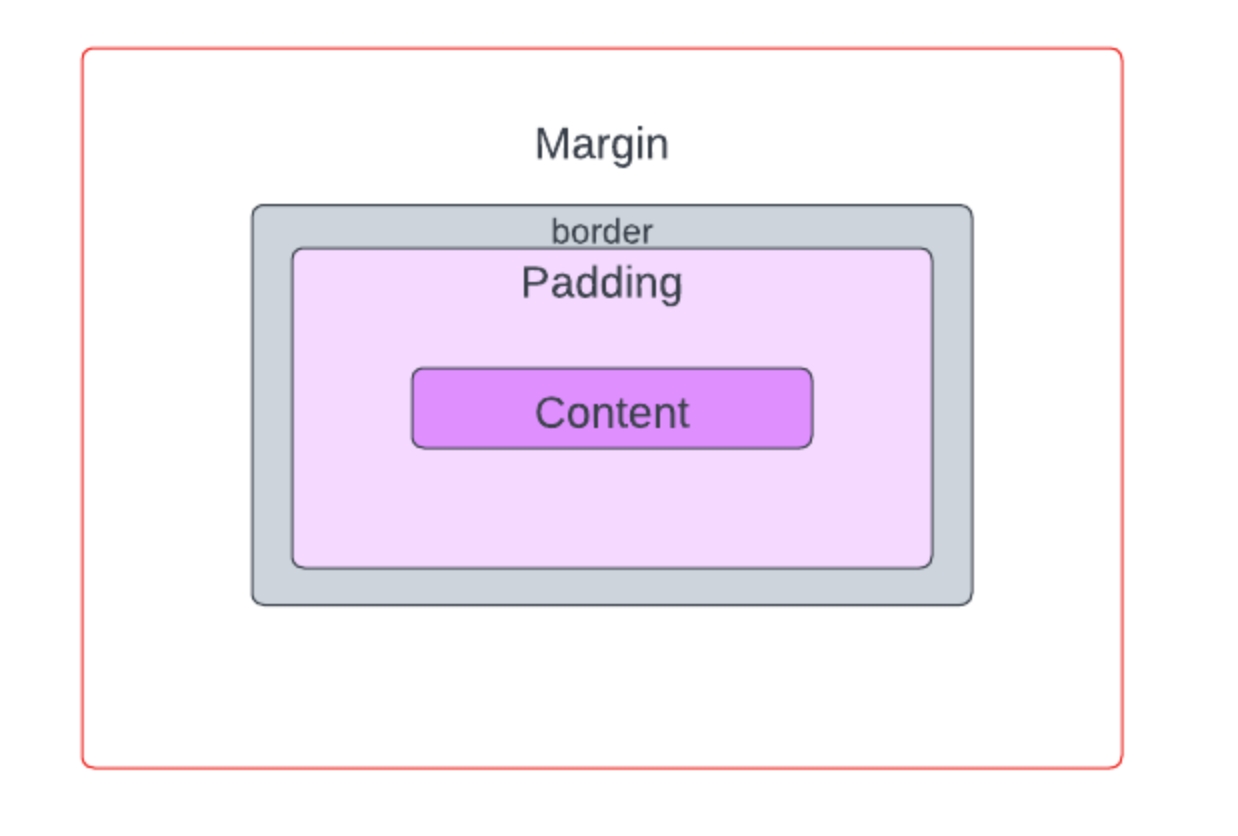
Here is the reference code:
Untitled
class LivePage extends StatelessWidget {
const LivePage({Key? key, required this.liveID, this.isHost = false})
: super(key: key);
final String liveID;
final bool isHost;
@override
Widget build(BuildContext context) {
return ZegoUIKitPrebuiltLiveStreaming(
appID: YourSecret.appID,
appSign: YourSecret.appSign,
userID: userID,
userName: 'user_$userID',
liveID: liveID,
// Modify your custom configurations here.
config: isHost
? ZegoUIKitPrebuiltLiveStreamingConfig.host()
: ZegoUIKitPrebuiltLiveStreamingConfig.audience()
..topMenuBar.height = 100
..topMenuBar.padding = const EdgeInsets.symmetric(
vertical: 10,
horizontal: 0,
)
..topMenuBar.margin = const EdgeInsets.symmetric(
vertical: 20,
horizontal: 0,
)
..topMenuBar.backgroundColor = Colors.red.withOpacity(0.2),
);
}
}
1
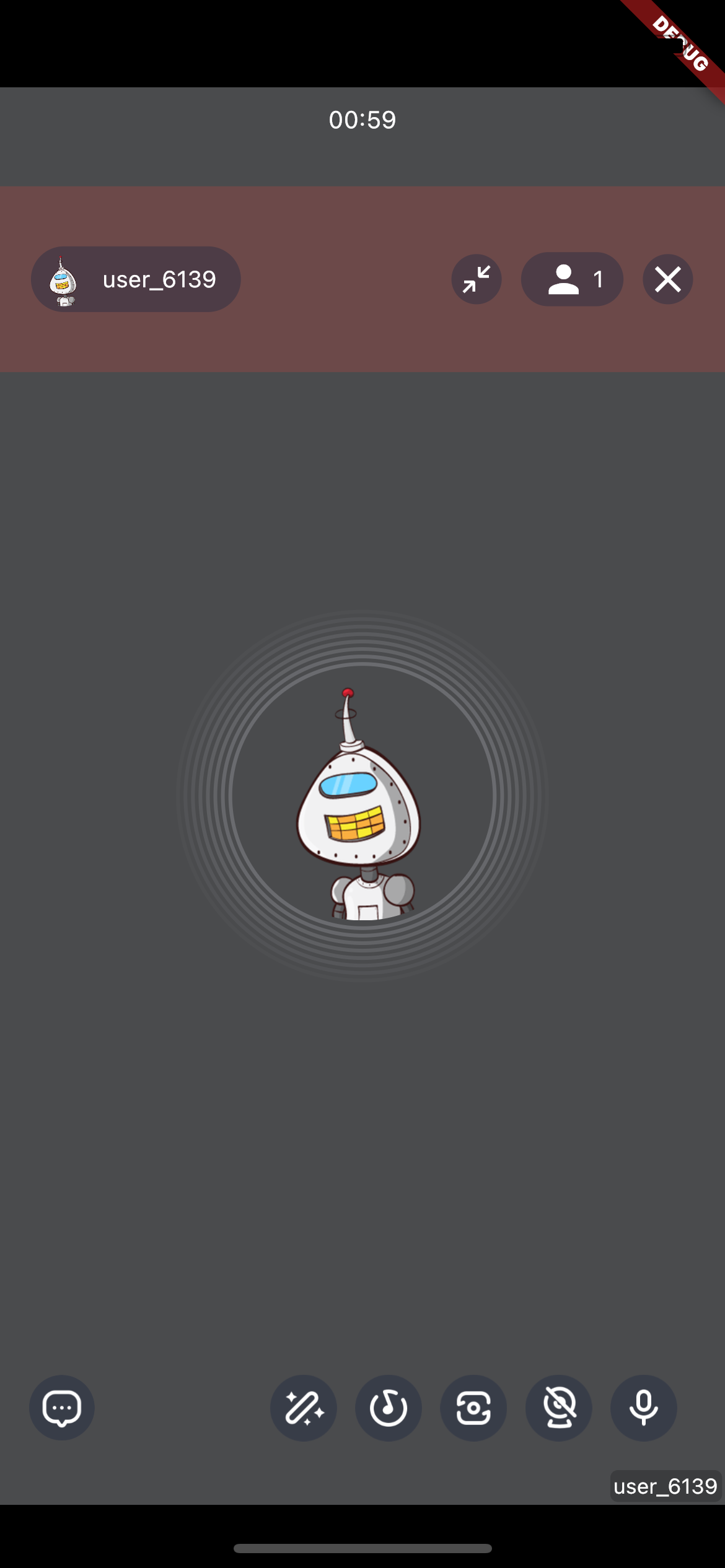