Quick start (with cohosting)
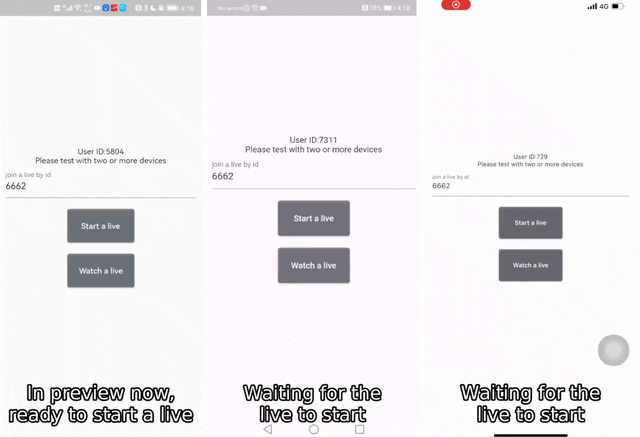
Prerequisites
- Go to ZEGOCLOUD Admin Console to create a UIKit project.
- Get the AppID and AppSign of the project.
If you don't know how to create a project and obtain an app ID, please refer to this guide.
Integrate the SDK
Add ZegoUIKitPrebuiltLiveStreaming as dependencies
Run the following code in your project's root directory:
Untitled
flutter pub add zego_uikit_signaling_plugin
flutter pub add zego_uikit_prebuilt_live_streaming
1
Import the SDK
Now in your Dart code, import the Live Streaming Kit SDK.
Untitled
import 'package:zego_uikit_signaling_plugin/zego_uikit_signaling_plugin.dart';
import 'package:zego_uikit_prebuilt_live_streaming/zego_uikit_prebuilt_live_streaming';
1
Using the Live Streaming Kit
- Specify the
userID
anduserName
for connecting the Live Streaming Kit service. liveID
represents the live stream you want to start or watch.- Set the
config
to theZegoUIKitSignalingPlugin
plug-in.
Note
userID
,userName
, andliveID
can only contain numbers, letters, and underlines (_).- Using the same
liveID
will enter the same live streaming.
Warning
With the same liveID
, only one user can enter the live stream as host. Other users need to enter the live stream as the audience.
Untitled
class LivePage extends StatelessWidget {
final String liveID;
final bool isHost;
const LivePage({Key? key, required this.liveID, this.isHost = false}) : super(key: key);
@override
Widget build(BuildContext context) {
return SafeArea(
child: ZegoUIKitPrebuiltLiveStreaming(
appID: yourAppID, // Fill in the appID that you get from ZEGOCLOUD Admin Console.
appSign: yourAppSign, // Fill in the appSign that you get from ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
liveID: liveID,
config: isHost
? ZegoUIKitPrebuiltLiveStreamingConfig.host(
plugins: [ZegoUIKitSignalingPlugin()],
)
: ZegoUIKitPrebuiltLiveStreamingConfig.audience(
plugins: [ZegoUIKitSignalingPlugin()],
),
),
);
}
}
1
Then, you can start a live stream. And the audience can watch the live stream by entering the liveID
.
Config your project
Android
iOS
Run & Test
Now you can simply click the Run or Debug button to run and test your App on the device.
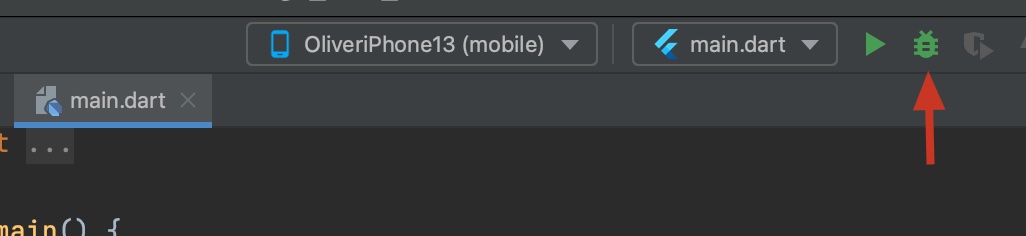
Resources
Sample code
Click here to get the complete sample code.
API reference
Click here for detailed explanations of all APIs.